Building a Flutter DateTime Picker in just 15 minutes
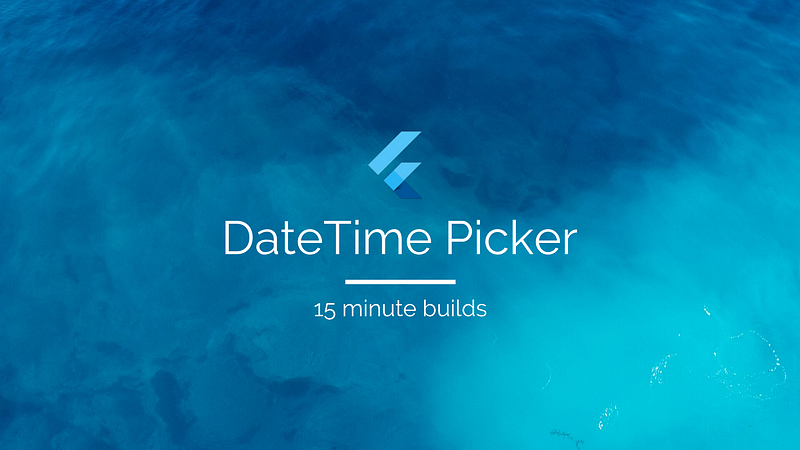
Introduction
With the Flutter DateTime Picker plugin, you can add date & time pickers to your native application. The plugin’s interface is inspired by iOS Cupertino style menu.
What you’ll build?
In this tutorial, you’ll build a mobile app featuring a DateTime Picker using the Flutter SDK. Your app will:
- Display separate Date & Time Pickers with a minimalistic interface
- Display the selected data as outputs to console
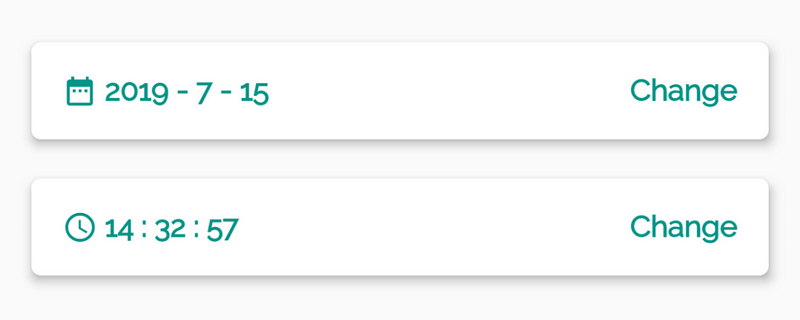
This tutorial focuses on adding a DateTime Picker to a Flutter app. Non-relevant concepts and code blocks are glossed over and are provided for you to simply copy and paste.
Github Repository | @ShivamGoyal1899
An app featuring DateTimePicker using the Flutter SDK - ShivamGoyal1899/DateTimePickergithub.com
Package Used | flutter_datetime_picker
Setting up Flutter on your machine
The detailed steps to install Flutter on your personal computer & getting started with Flutter is available at the following blog
Learn what is Flutter and how to set it up on Windows and Mac systems and get started with Flutter appsmedium.com
Coding the component
Component Syntax
The basic format of a DateTime Picker looks like the one below:
FlatButton( onPressed: () { DatePicker.showDatePicker(context, showTitleActions: true, minTime: DateTime(2000, 1, 1) maxTime: DateTime(2022, 12, 31), onChanged: (date) {print('change $date');}, onConfirm: (date) {print('confirm $date');}, currentTime: DateTime.now(), locale: LocaleType.en);}, child: Text('Show DateTime Picker',) );
Adding DateTime Picker plugin as a dependency
Adding additional capability to a Flutter app is easy using Pub packages. In this tutorial, you introduce the DateTime Picker plugin by adding a single line to the pubspec.yaml
file.
Importing plugin to main.dart file
Import flutter_datetime_picker dependency to your main.dart file by adding the following line at the starting of the file:
import 'package:flutter_datetime_picker/flutter_datetime_picker.dart';
Putting Code in action
Amend your main.dart file as per the following code:
Building & running the application
- Connect your Emulator or physical Android device to test the application.
- Click on Build & Run.
- And Boooom, your app is ready.
The final build would look like the below illustration.
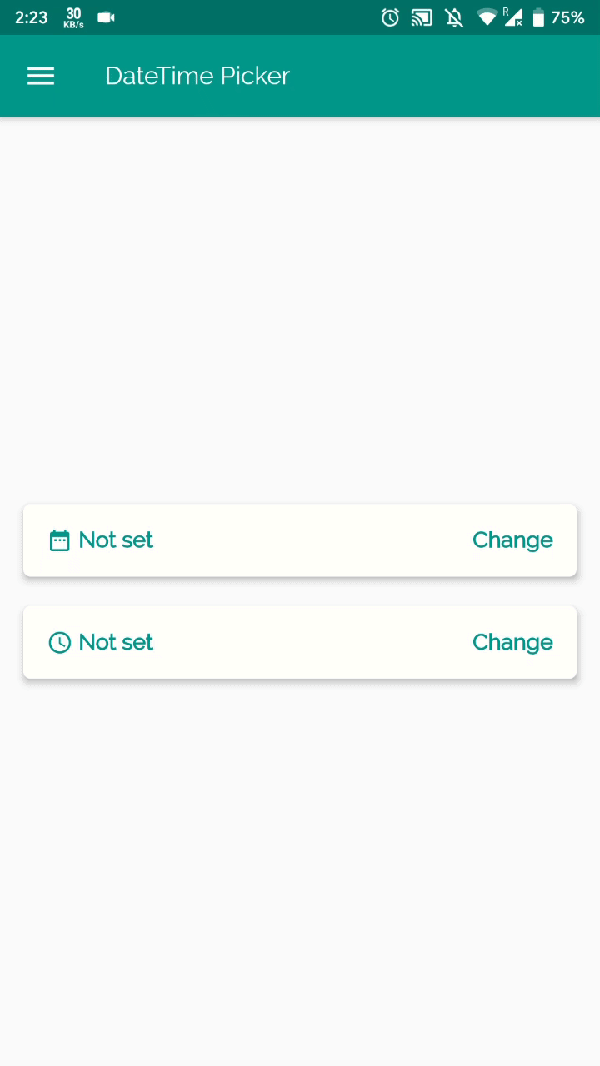
Customization Options
There are three functional variations of the plugin available as follows:
- Solo DatePicker
- Solo TimePicker
- Dual DateTimePicker
Language Options
There are various language options available to implement the plugin for international use. For changing the language of the component amend the following with preferred LocaleType.
locale: LocaleType.en
- English(en)
- Persian(fa)
- Chinese(zh)
- Dutch(nl)
- Russian(ru)
- Italian(it)
- French(fr)
- Spanish(es)
- Polish (pl)
- Portuguese(pt)
- Korean(ko)
- Arabic(ar)
- Turkish(tr)
- Japanese(jp)
- German(de)
- Danish(da)
- Bengali(bn)
- Vietnamese(vi)
- Armenian(hy)
Further Customisations
If you want to customize your own style of date time picker, there is a class called CommonPickerModel, every type of date time picker is extended from this class, you can refer to other picker models (eg. DatePickerModel), and write your custom one, then pass this model to showPicker method, so that your own date time picker will appear, it’s easy, and will perfectly meet your demand.
How to customize your own picker model:
class CustomPicker extends CommonPickerModel { String digits(int value, int length) { return '$value'.padLeft(length, "0"); }
CustomPicker({DateTime currentTime, LocaleType locale}) : super(locale: locale) { this.currentTime = currentTime ?? DateTime.now(); this.setLeftIndex(this.currentTime.hour); this.setMiddleIndex(this.currentTime.minute); this.setRightIndex(this.currentTime.second); }
@override String leftStringAtIndex(int index) { if (index >= 0 && index < 24) { return this.digits(index, 2); } else { return null; } }
@override String middleStringAtIndex(int index) { if (index >= 0 && index < 60) { return this.digits(index, 2); } else { return null; } }
@override String rightStringAtIndex(int index) { if (index >= 0 && index < 60) { return this.digits(index, 2); } else { return null; } }
@override String leftDivider() { return "|"; }
@override String rightDivider() { return "|"; }
@override List<int> layoutProportions() { return [1, 2, 1]; }
@override DateTime finalTime() { return currentTime.isUtc ? DateTime.utc(currentTime.year, currentTime.month, currentTime.day, this.currentLeftIndex(), this.currentMiddleIndex(), this.currentRightIndex()) : DateTime(currentTime.year, currentTime.month, currentTime.day, this.currentLeftIndex(), this.currentMiddleIndex(), this.currentRightIndex()); } }