Guide on React Native Full App
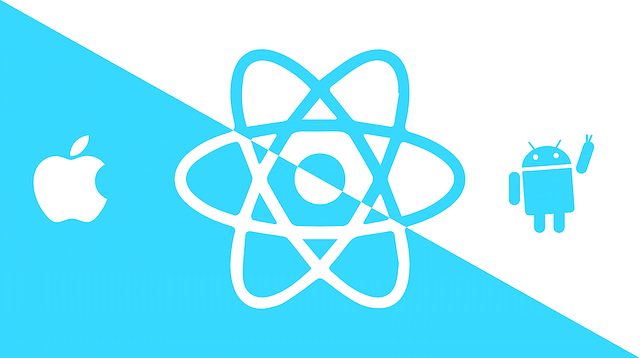
React Native lets you build mobile apps using only JavaScript. It uses the same design as React, letting you compose a rich mobile UI using declarative components.
What Is React Native?
React Native is a JavaScript framework for writing real, natively rendering mobile applications for iOS and Android. It’s based on React, Facebook’s JavaScript library for building user interfaces, but instead of targeting the browser, it targets mobile platforms. In other words: web developers can now write mobile applications that look and feel truly “native,” all from the comfort of a JavaScript library that we already know and love. Plus, because most of the code you write can be shared between platforms, React Native makes it easy to simultaneously develop for both Android and iOS.
Similar to React for the Web, React Native applications are written using a mixture of JavaScript and XML markup, known as JSX. Then, under the hood, the React Native “bridge” invokes the native rendering APIs in Objective-C (for iOS) or Java (for Android). Thus, your application will render using real mobile UI components, not webviews, and will look and feel like any other mobile application. React Native also exposes JavaScript interfaces for platform APIs, so your React Native apps can access platform features like the phone camera, or the user’s location.
React Native currently supports both iOS and Android and has the potential to expand to future platforms as well. In this blog, we’ll cover both iOS and Android. The vast majority of the code we write will be cross-platform. And yes: you can really use React Native to build production-ready mobile applications! Some example: Facebook, Palantir, and TaskRabbit are already using it in production for user-facing applications.
Advantages of React Native
The fact that React Native actually renders using its host platform’s standard rendering APIs enables it to stand out from most existing methods of cross-platform application development, like Cordova or Ionic. Existing methods of writing mobile applications using combinations of JavaScript, HTML, and CSS typically render using webviews. While this approach can work, it also comes with drawbacks, especially around performance. Additionally, they do not usually have access to the host platform’s set of native UI elements. When these frameworks do try to mimic native UI elements, the results usually “feel” just a little off; reverse-engineering all the fine details of things like animations takes an enormous amount of effort, and they can quickly become out of date.
In contrast, React Native actually translates your markup to real, native UI elements, leveraging existing means of rendering views on whatever platform you are working with. Additionally, React works separately from the main UI thread, so your application can maintain high performance without sacrificing capability. The update cycle in React Native is the same as in React: when props
or state
change, React Native re-renders the views. The major difference between React Native and React in the browser is that React Native does this by leveraging the UI libraries of its host platform, rather than using HTML and CSS markup.
For developers accustomed to working on the Web with React, this means you can write mobile apps with the performance and look and feel of a native application while using familiar tools. React Native also represents an improvement over normal mobile development in two other areas: the developer experience and cross-platform development potential.
A React Native app is a real mobile app
The apps you are building with React Native aren’t mobile web apps because React Native uses the same fundamental UI building blocks as regular iOS and Android apps. Instead of using Swift, Kotlin or Java, you are putting those building blocks together using JavaScript and React.
Don’t waste time recompiling
React Native lets you build your app faster. Instead of recompiling, you can reload your app instantly. With Hot Reloading, you can even run new code while retaining your application state. Give it a try — it’s a magical experience.
Use native code when you need to
React Native combines smoothly with components written in Swift, Java, or Objective-C. It’s simple to drop down to native code if you need to optimize a few aspects of your application. It’s also easy to build part of your app in React Native, and part of your app using the native code directly — that’s how the Facebook app works.
Developer Experience
If you’ve ever developed for mobile before, you might be surprised by how easy React Native is to work with. The React Native team has baked strong developer tools and meaningful error messages into the framework, so working with robust tools is a natural part of your development experience.
For instance, because React Native is “just” JavaScript, you don’t need to rebuild your application in order to see your changes reflected; instead, you can hit Command+R to refresh your application just as you would any other web page. All of those minutes spent waiting for your application to build can really add up, and in contrast, React Native’s quick iteration cycle feels like a godsend.
Additionally, React Native lets you take advantage of intelligent debugging tools and error reporting. If you are comfortable with Chrome or Safari’s developer tools, you will be happy to know that you can use them for mobile development, as well. Likewise, you can use whatever text editor you prefer for JavaScript editing: React Native does not force you to work in Xcode to develop for iOS or Android Studio for Android development.
Besides the day-to-day improvements to your development experience, React Native also has the potential to positively impact your product release cycle. For instance, Apple permits JavaScript-based changes to an app’s behavior to be loaded over the air with no additional review cycle necessary.
All of these small perks add up to saving you and your fellow developers time and energy, allowing you to focus on the more interesting parts of your work and be more productive overall.
Code Reuse and Knowledge Sharing
Working with React Native can dramatically shrink the resources required to build mobile applications. Any developer who knows how to write React code can now target the Web, iOS, and Android, all with the same skillset. By removing the need to “silo” developers based on their target platform, React Native lets your team iterate more quickly, and share knowledge and resources more effectively.
Besides shared knowledge, much of your code can be shared, too. Not all the code you write will be cross-platform, and depending on what functionality you need on a specific platform, you may occasionally need to dip into Objective-C or Java. But reusing code across platforms is surprisingly easy with React Native. For example, the Facebook Ads Manager application for Android shares 87% of its codebase with the iOS version, as noted in the React Europe 2015 keynote.
Risks and Drawbacks
As with anything, using React Native is not without its downsides, and whether or not React Native is a good fit for your team really depends on your individual situation.
The largest risk is probably React Native’s maturity, as the project is still relatively young. iOS support was released in March 2015, and Android support was released in September 2015. The documentation certainly has room for improvement and continues to evolve. Some features on iOS and Android still aren’t supported, and the community is still discovering best practices. The good news is that in the vast majority of cases, you can implement support for missing APIs yourself.
Because React Native introduces another layer to your project, it can also make debugging hairier, especially at the intersection of React and the host platform.
React Native is still young, and the usual caveats that go along with working with new technologies apply here. Still, on the whole, I think you’ll see that the benefits outweigh the risks.
How Does React Native Work?
The idea of writing mobile applications in JavaScript feels a little odd. How is it possible to use React in a mobile environment? In order to understand the technical underpinnings of React Native, first, we’ll need to recall one of React’s features, the Virtual DOM.
In React, the Virtual DOM acts as a layer between the developer’s description of how things ought to look, and the work is done to actually render your application onto the page. To render interactive user interfaces in a browser, developers must edit the browser’s DOM or Document Object Model. This is an expensive step, and excessive writes to the DOM have a significant impact on performance. Rather than directly render changes on the page, React computes the necessary changes by using an in-memory version of the DOM, and rerenders the minimal amount necessary.
Installation
In this step, I will help you install and build your first React Native app. If you already have React Native installed, you can skip this step. and yes we will cover Expo installation in this step because Expo is the easiest way to start building a new React Native application. It allows you to start a project without installing or configuring any tools to build native code — no Xcode or Android Studio installation required (see Caveats).
Assuming that you have Node installed, you can use npm to install the Expo CLI command line utility:
npm install -g expo-cli
Then run the following commands to create a new React Native project called “AwesomeProject”:
expo init AwesomeProject
cd AwesomeProject
npm start
This will start a development server for you.
Running your React Native application
Install the Expo client app on your iOS or Android phone and connect to the same wireless network as your computer. On Android, use the Expo app to scan the QR code from your terminal to open your project. On iOS, follow on-screen instructions to get a link.
Modifying your app
Now that you have successfully run the app, let’s modify it. Open App.js
in your text editor of choice and edit some lines. The application should reload automatically once you save your changes.
That’s it! Congratulations! You’ve successfully run and modified your first React Native app.
Running your app on a simulator or virtual device
Expo CLI makes it really easy to run your React Native app on a physical device without setting up a development environment. If you want to run your app on the iOS Simulator or an Android Virtual Device, please refer to the instructions for building projects with native code to learn how to install Xcode and set up your Android development environment.
Once you’ve set these up, you can launch your app on an Android Virtual Device by running npm run android
, or on the iOS Simulator by running npm run ios
(macOS only).
Caveats
Because you don’t build any native code when using Expo to create a project, it’s not possible to include custom native modules beyond the React Native APIs and components that are available in the Expo client app.
If you know that you’ll eventually need to include your own native code, Expo is still a good way to get started. In that case you’ll just need to “eject” eventually to create your own native builds. If you do eject, the “Building Projects with Native Code” instructions will be required to continue working on your project.
Expo CLI configures your project to use the most recent React Native version that is supported by the Expo client app. The Expo client app usually gains support for a given React Native version about a week after the React Native version is released as stable. You can check this document to find out what versions are supported.
If you’re integrating React Native into an existing project, you’ll want to skip Expo CLI and go directly to setting up the native build environment. Select “Building Projects with Native Code” above for instructions on configuring a native build environment for React Native.
Follow these instructions if you need to build native code in your project. For example, if you are integrating React Native into an existing application, or if you “ejected” from Expo or Create React Native App, you’ll need this section.
The instructions are a bit different depending on your development operating system, and whether you want to start developing for iOS or Android. If you want to develop for both iOS and Android, that’s fine — you just have to pick one to start with, since the setup is a bit different.
Installing dependencies
You will need Node, the React Native command line interface, Python2, a JDK, and Android Studio.
While you can use any editor of your choice to develop your app, you will need to install Android Studio in order to set up the necessary tooling to build your React Native app for Android.
A Mac is required to build projects with native code for iOS. You can follow the Quick Start to learn how to build your app using Expo instead.
How to use React Native Full app
React native full app is loaded with a large number of options, layouts, and functionalities. This is a complete starter app to get started with react-native
Why use a template at all?
There are several benefits of making your app from a template like above. Let’s go through the key benefits
- Cheaper — You save thousands of dollars spent in UI development. This is a crucial phase where a majority of startups end up spending most of their time and money. Designing a feature from scratch is not worth if you can just pick the feature off the shelf for fraction of the price.
- Faster — You save weeks (if not months) of time which you would otherwise spend on designing and developing your app’s UI. Faster time to market is often the deal maker for an app startup.
- Keep your team smaller — If you are an early stage startup, and the co-founders are not developers, it is not wise to hire separate developers for UI designing, and then UI development on different devices. Using a hybrid app (same technology for both iOS and Android) halves your problem, but you would still love to have an off-the-shelf template. This way you can jump to feature integration directly and keep your team smaller
- Standard and Bug-free — One of the biggest advantages of using a good app template is the standard feature and code structure. It is often seen that the founding team develops an app with whatever they know. This results in a badly written (although working) code. Instead, you can use an off-the-shelf code written with proper standards, and mostly bug-free. You cut costs, save time and get better quality code. How much more can one ask for!
- Standard features — Other than great code standards, you get standard features already working and device tested in templates. E.g. A tinder card format has been a popular layout for the past many years. Creating the layout on your own from scratch is not worth if you can just pick it up from an app template like react-native full app
What do you get with a template like React native full app
A complete feature comparison table has been formulated for your reference. Take a look below:
Isn’t the cost too much for Full App template?
Well, let’s do the Math.
React Native Full App contains features that can easily create at least 10 independent apps for you. If each app’s UI take even a mere 50–100 hours of your time, at a development rate of $50–100/hr, you can imagine the cost you will bear for the UI development alone.
You get all of that stuff ready-made in Ionic 4 Full App. Adjusting the template to your use might take 5–10 hours. So in total, you can save anywhere between $2500-$10000. If you are a development agency or a freelancer, creating multiple apps for clients, then the template serves you an even bigger advantage.
So one question will arise in your mind that React Native full App has lots of plugin and UI components in it so how can I use a single component from Full app. wait I will explain you this as well
So we will take all module one by one.
Firebase Crud, Storage and Auth Module:-
For using Firebase Auth and CRUD module you have to take firebase folder from App
, views
and components
folder from our app and paste it into your project. Now go to Navigation
folder in our app, open AppNavigation.tsx
file, copy this line (Note: check path in your folder structure),
import HomePage from '../views/firebase/HomePage/HomePage'; import UploadPage from '../views/firebase/UploadPage';
paste this into your navigation file. and add this line
Crud: { screen: HomePage },
In your navigator in AppNavigation file like this.
const AppNavigator = createDrawerNavigator({ Crud: { screen: HomePage } })
for Authentication check of a user, Just Copy AuthNavigation.tsx
file, And paste into your navigation folder.
Modify your index file for navigation like this
const SwitchNavigator = createSwitchNavigator({
Landing: AuthCheck,
Auth: AuthNavigator,
App: AppNavigator
}, {
initialRouteName: 'Landing'
});
This code checks user auth and navigates the user to the home page if he is already logged in where he can perform all CRUD operations. And if he is not logged in it will redirect him to Login Page.
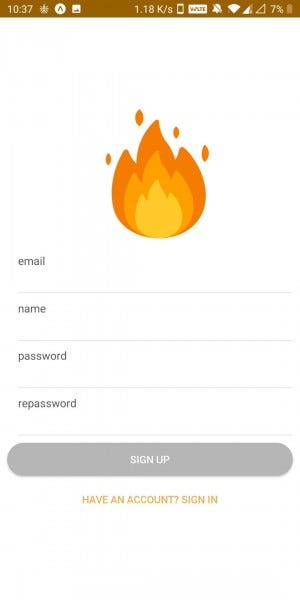
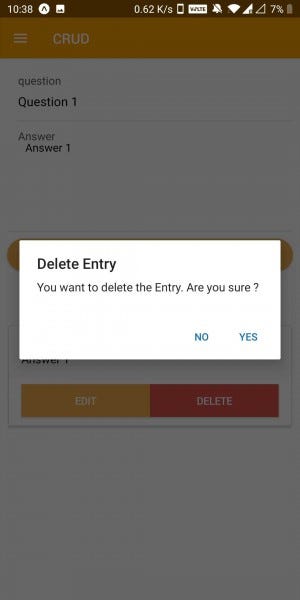
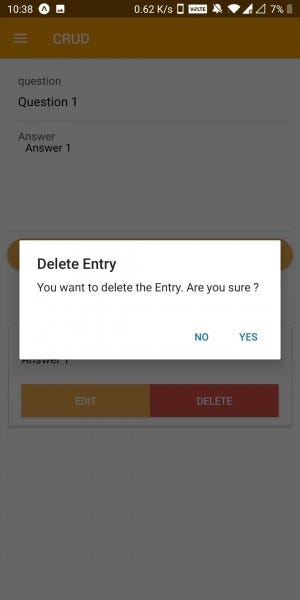
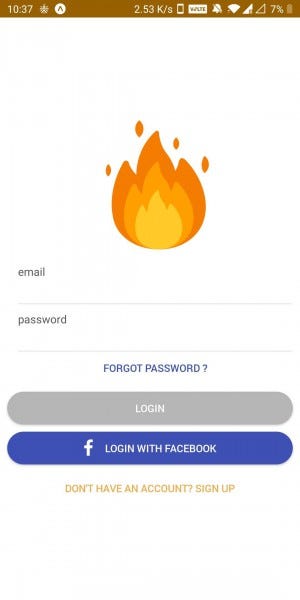
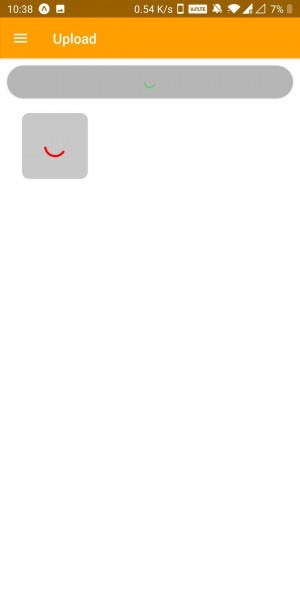
Tinder Module:-
For using Tinder module you have to take TinderCards
folder from views/start
folder and take TinderTabs
and ChatInput
folder from components/star
folder
Follow all Navigation steps as I explained in the Firebase module.
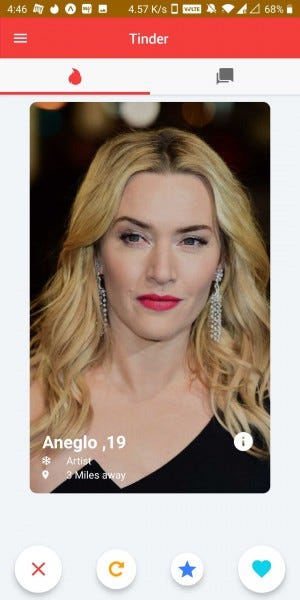
Grocery Module:-
For adding grocery module just copy grocery folder from Views and component folder and paste into your working directory, change all imports according to your folder structure
Follow all Navigation steps as I explained in the Firebase module.
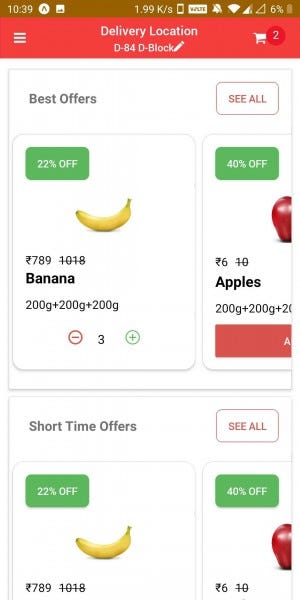
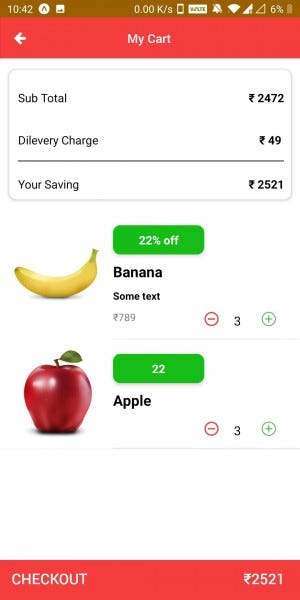
Instagram Module:-
For using Instagram module you have to take Instagram
folder from views/start
folder and take picker
, header
and scrollableCards
folder from components/star
folder
Follow all Navigation steps as I explained in the Firebase module.
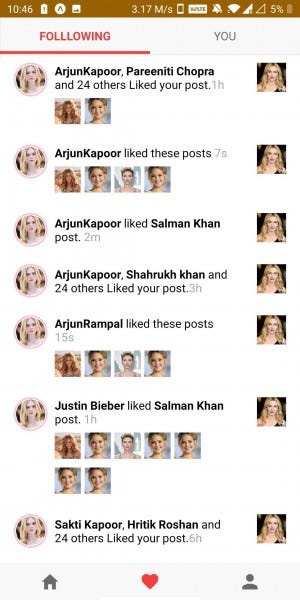
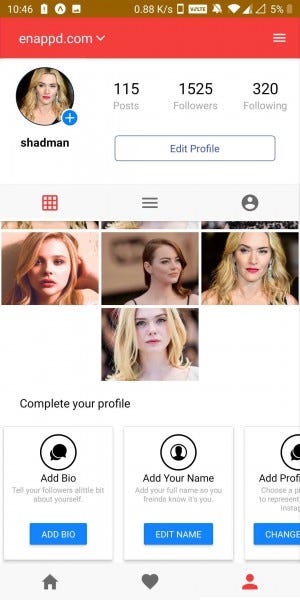
Netflix Module:-
For using Netflix module you have to take Netflix
folder from views/start
folder and take header
and netflixCards
folder from components/star
folder
Follow all Navigation steps as I explained in the Firebase module.
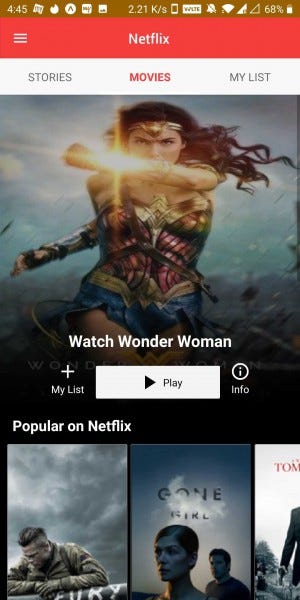
Redux Module:-
For using Redux module you have to take Redux folder from app and views folder, and grocery
folder from components
because we have added redux in grocery app module.
Follow all Navigation steps as I explained in the Firebase module.
How to use plugins from React Native full app:-
We have lots of plugin in our full app for and the plugin has its separate directory in views. So you to take folder for that plugin from views folder
Follow all Navigation steps as I explained in the Firebase module.
And yes you are ready to go.
Happy Coding.
FOUND THIS POST INTERESTING?
Also check out our other blog posts related to Firebase in Ionic 4, Geolocation in Ionic 4, QR Code and scanners in Ionic 4 and Payment gateways in Ionic 4
Also, check out this interesting post on How to create games in Ionic 4 with Phaser
Other Products from Enappd:
· Ticket Booking app starter (Ionic4)- Evento
· E-commerce app starter (Ionic 4)- Shoppr
· Premium Chat themes (Ionic4)– Chatter
· Gym | Fitness | Yoga app starter (Ionic 4)- FitCulture
· Yoga app starter (Ionic 4)- Yogi
· Real estate | House search | Flat search app starter (Ionic 4)
· Short Viral news App starter (Ionic 4)
· Grocery shopping & delivery app starter (Ionic 3)
· Buzzfeed style app / viral news app starter (Ionic 3)
· Doctor search app / Doctor appointment app starter (Ionic 3)
· Hotel booking app starter (Ionic 3)
· Online video course app/ E-learning starter (Ionic 3)
· Food delivery app / Food search app starter (Ionic 3)
· Video streaming app starter (React Native)
· Spotify style app / Music streaming app starter (React Native)
Summary
React Native is an exciting framework that enables web developers to create robust mobile applications using their existing JavaScript knowledge. It offers faster mobile development, and more efficient code sharing across iOS, Android, and the Web, without sacrificing the end user’s experience or application quality. The tradeoff is that it’s new, and still a work in progress. If your team can handle the uncertainty that comes with working with new technology and wants to develop mobile applications for more than just one platform, you should be looking at React Native.