Navigations in React Native App
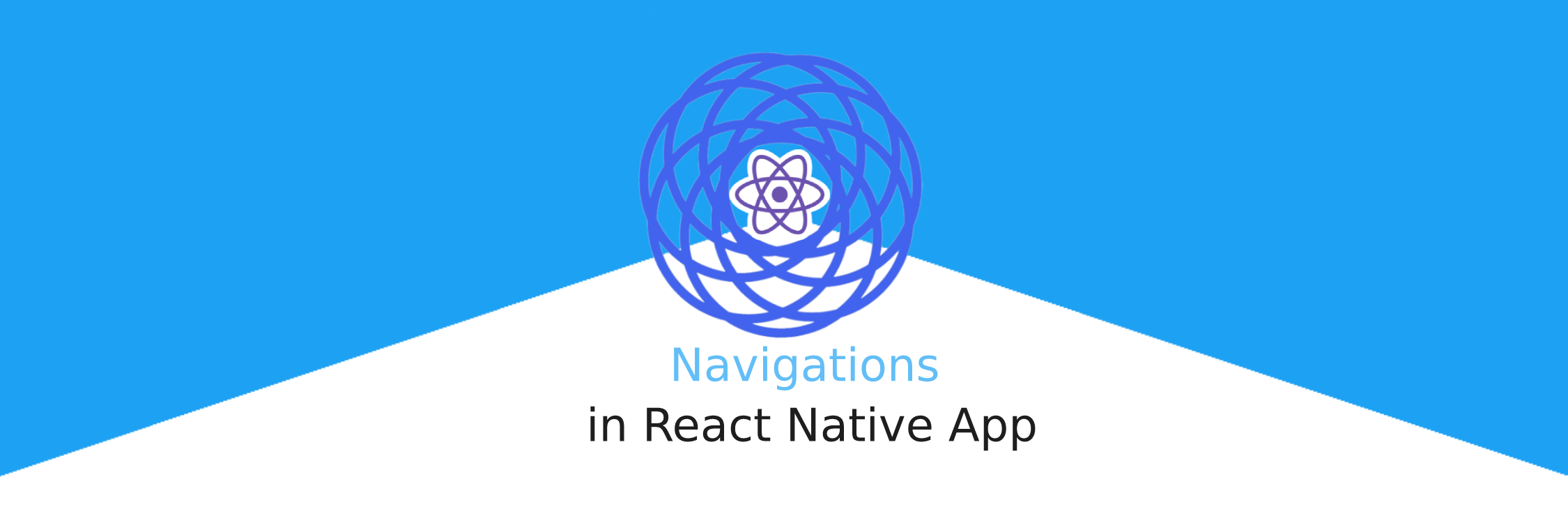
In this project, we will learn different navigation methods including StackNavigator, Drawer Navigator and SwitchNavigator provided by react-native. We will also see the different configurations and properties of these navigations styles using react-navigation
.
Complete source code of this tutorial is available here — RNNavigations
What is React-Native?
TLDR; — React Native (RN) creates cross-platform apps, more “native” than web-view apps made by Cordova / Ionic. But React Native is yet to release a stable (1.0.0_) version of the framework.
React Native is a JavaScript framework for writing natively rendering mobile applications. It’ is based on React, Facebook’s JavaScript library for building user interfaces, but instead of targeting the browser, it targets mobile platforms. Because most of the code you write can be shared between platforms, React Native makes it easy to simultaneously develop for both Android and iOS.
React Native applications render using real mobile UI components, not web-views, and will look and feel like any other native mobile application. React Native also exposes JavaScript interfaces for platform APIs, so your React Native apps can access platform features like the phone camera, or the user’s location. Facebook, Palantir, TaskRabbit etc are already using it in production for user-facing applications.
React Native and Navigations
React Navigation is born from the React Native community’s need for an extensible yet easy-to-use navigation solution written entirely in JavaScript (so you can read and understand all of the sources), on top of powerful native primitives.
Before you commit to using React Navigation for your project, you might want to read the anti-pitch — it will help you to understand the tradeoffs that we have chosen along with the areas where we consider the library to be deficient currently.
Post structure
We will go in a step-by-step manner to explore all the features of react-navigation. This is my break-down of the blog
STEPS
- Create a simple React Native app
- Install packages for
react-navigation
,stack-navigation
,drawer-navigation
, andbottom-tab-navigation
. - Set up all the navigation structure for different navigations.
- Use different navigation options for different navigations.
We have three major objectives
- React-Navigation set up for different navigation styles.
- Use each navigation routes for different sections in the application.
- Passing parameters using navigation from one page to another.
Let’s dive right in!
Step 1— Create a basic React Native app
First, make sure you have all pre-requisites to create a react-native app as per the official documentation.
At the time of this post, I have React-Native version 0.60.5
Create a blank react-native app (Replace RNavigations
with your own app name)
$ react-native init RNNavigations
This will create a basic React-native app that you can run in a device or simulator. Let’s run the app in android using
$ react-native run-android
You’ll see the default start screen on the device/simulator.
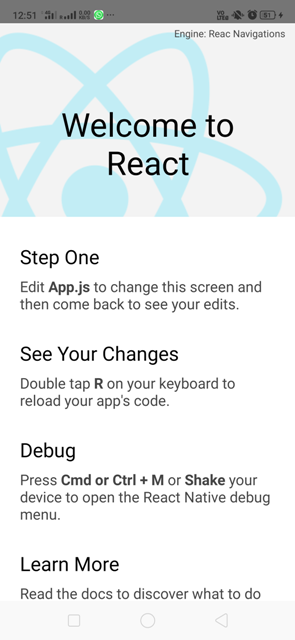
Steps to implement
step 1 — Install react-navigation package using the below command
yarn add react-navigation
# or with npm
# npm install react-navigation
Installing dependencies into a bare React Native project
In your project directory, run yarn add react-native-reanimated react-native-gesture-handler react-native-screens@^1.0.0-alpha.23
.
Next, we need to link these libraries. The steps depend on your React Native version:
- React Native 0.60 and higher
- On newer versions of React Native, linking is automatic.
- To finalize the installation of
react-native-screens
for Android, add the following two lines todependencies
section inandroid/app/build.gradle
:
implementation 'androidx.appcompat:appcompat:1.1.0-rc01'
implementation 'androidx.swiperefreshlayout:swiperefreshlayout:1.1.0-alpha02'
React Native 0.59 and lower
If you’re on an older React Native version, you need to manually link the dependencies. To do that, run:
react-native link react-native-reanimated
react-native link react-native-gesture-handler
react-native link react-native-screens
You also need to configure jetifier to support dependencies using androidx
:
yarn add --dev jetifier
# or with npm
# npm install --save-dev jetifier
To finalize the installation of react-native-gesture-handler
for Android, make the following modifications to MainActivity.java
:
package com.reactnavigation.example;
import com.facebook.react.ReactActivity;
+ import com.facebook.react.ReactActivityDelegate;
+ import com.facebook.react.ReactRootView;
+ import com.swmansion.gesturehandler.react.RNGestureHandlerEnabledRootView;
public class MainActivity extends ReactActivity {
@Override
protected String getMainComponentName() {
return "Example";
}
+ @Override
+ protected ReactActivityDelegate createReactActivityDelegate() {
+ return new ReactActivityDelegate(this, getMainComponentName()) {
+ @Override
+ protected ReactRootView createRootView() {
+ return new RNGestureHandlerEnabledRootView(MainActivity.this);
+ }
+ };
+ }
}
Here in the above MainActivity.java remove the + signs while adding it in your app.
Now in the latest react-navigation, we need to install each navigation separately except switch navigation in our app. So we would need those installations too in our app. Let’s go and do this in a moment.
yarn add react-navigation-stack yarn add react-navigation-drawer yarn add react-navigation-tabs
Now you are all set to play with the react-navigation stuff.
We will implement each navigation methods one by one in the same application as:
- SwitchNavigator
- DrawerNavigator
- BottomTabsNAvigator
- StackNavigator
We are going to use Switch Navigation to divide our navigations into differents slots like Stack Navigation for Application authentications and when we successfully get authenticated then we switch our app to drawer navigations and after performing some stuff inside drawer we will switch to bottom tabs navigator.
Let us know first What the above navigation method is used for?
- SwitchNavigator: —
The purpose of SwitchNavigator is to only ever show one screen at a time. By default, it does not handle back actions and it resets routes to their default state when you switch away. This is the exact behavior that we want from the authentication flow.
- DrawerNavigator: —
The purpose of DrawerNavigator is to show more than one screen option in the side-menu layout at a time. You can click on any menu item and open that particular page on the screen.
- BottomTabsNAvigator: —
A simple tab bar on the bottom of the screen that lets you switch between different routes. Routes are lazily initialized — their screen components are not mounted until they are first focused.
- StackNavigator: —
It provides a way for your app to transition between screens where each new screen is placed on top of a stack.
By default the stack navigator is configured to have the familiar iOS and Android look & feel: new screens slide in from the right on iOS, fade in from the bottom on Android. On iOS, the stack navigator can also be configured to a modal style where screens slide in from the bottom.
Now we have all the required concepts in detail and in easy to understand language.
Now I am going to implement each one by one in an explained way.
Right now my code structure is as below image.
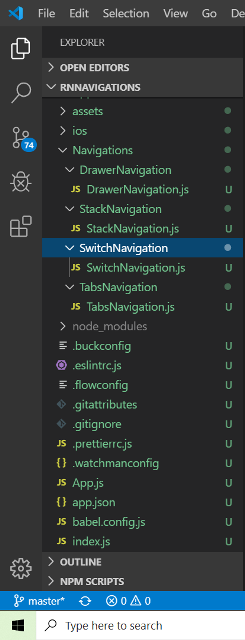
Here all the navigations are in the Navigations folder containing each navigation method.
1: — Switch Navigation
import React, { Component } from 'react';
import { createSwitchNavigator, createAppContainer } from 'react-navigation';
import StackNav from '../StackNavigation/StackNavigation';
import DrawerNav from '../DrawerNavigation/DrawerNavigation';
import TabsNav from '../TabsNavigation/TabsNavigation';
const SwitchNavigator = createSwitchNavigator({
Auth: StackNav,
Drawer: DrawerNav,
Tabs: TabsNav }, { initialRouteName: 'Auth', }) const SwitchNav = createAppContainer(SwitchNavigator); export default (SwitchNav);
The above code contains Switch Navigation. We are using switch navigation to perform some particular tasks on specific navigation and then move to the next methods so that we can not get back.
Here switch navigation has three routes as Auth
, Drawer
, Tabs
.The Auth contains Stack navigation with two route StackHome
and Login
.
In the above switch navigation, we have assigned navigations option as initialRouteName
, Setting this method allows the app to load that route as app loads.
We are going to see how the Switch navigator works with the navigation flow.
2: — Auth with Stack Navigator:—
The auth route contains stack navigation as: —
Stack navigation works on stack flow so It gives us a default header option which we can disable using navigation options as I have done above for login but enabled for home so that I can use the header and default back button advantage to go back to the previous page.
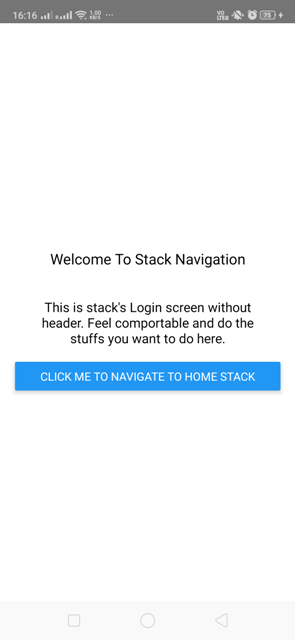
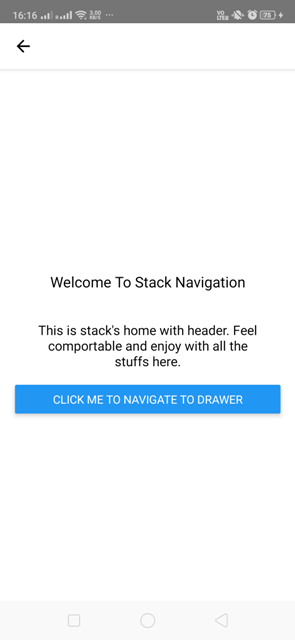
On Clicking on the button to navigate to the drawer, it will navigate us to the Drawer Stack
. To navigate from one page to another page in react-native using react-navigation we use to write a function and call that onPress event as here syntax for navigating one to another page is: —
this.props.navigation.navigate('RouteNameForNavigateTo')
Replace RouteNameForNavigateTo
with your route name.
Here with this navigation syntex we can pass some data as the route parameter and receive that in the destined route using getParam() method as:-
There are two pieces to this:
- Pass params to a route by putting them in an object as a second parameter to the
navigation.navigate
function:this.props.navigation.navigate('RouteName', { /* params go here */ })
- Read the params in your screen component:
this.props.navigation.getParam(paramName, defaultValue)
.
We can send data as a parameter like this with the navigation: —
onPress={() => { this.props.navigation.navigate('Details', { itemId: 86, otherParam: 'anything you want here', });
And receive that data in the destined page like this: —
const receiveData = this.props.navigation.getParam('itemId'); console.log(receiveData);
The most important thing here to keep in mind while passing and receiving parameters is that we need to assign the same name while receiving the params which we had passed while passing it with the route.
We need to receive this in componentDidMount() or any preferred lifecycle method which loads as the page gets navigated or navigated back.
To know more about passing parameters you can visit this link.
3: — Drawer Navigation
After clicking on the “Click me to navigate to drawer” button on the above image it will navigate us to DrawerNavigator
.
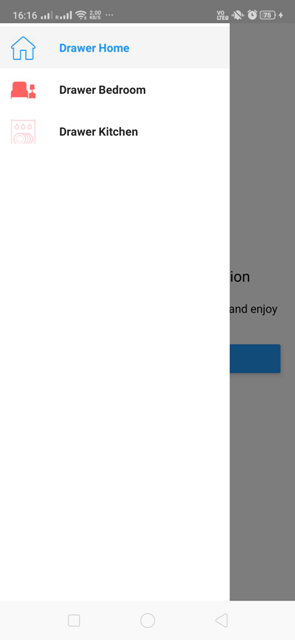
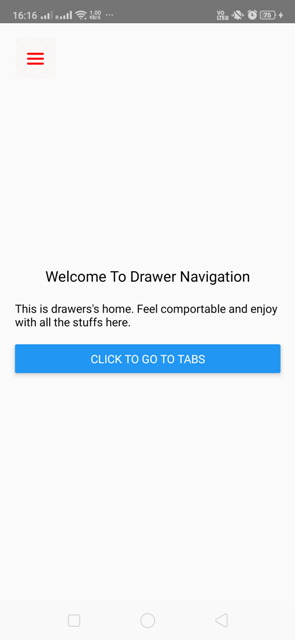
In drawer navigation, I have made some pages for smooth navigation of pages as you can see in the above image.
Some of the basic methods that drawer gives us are as:-
To open and close the drawer, use the following helpers to open and close the drawer:
this.props.navigation.openDrawer();
this.props.navigation.closeDrawer();
If you would like to toggle the drawer you call the following:
this.props.navigation.toggleDrawer();
Each of these functions, behind the scenes, are simply dispatching actions:
this.props.navigation.dispatch(DrawerActions.openDrawer());
this.props.navigation.dispatch(DrawerActions.closeDrawer());
this.props.navigation.dispatch(DrawerActions.toggleDrawer());
If you would like to determine if the drawer is open or closed, you can do the following:
const parent = this.props.navigation.dangerouslyGetParent();
const isDrawerOpen = parent && parent.state && parent.state.isDrawerOpen;
To beautify our drawer or to add icons or images in our side menu, react-navigation provides us navigation options for drawer decoration as: —
navigationOptions: { title: 'Drawer Home', drawerIcon: ({ tintColor }) => ( <Image source={require('../../assets/home.png')} style={[{ width: 30, height: 30 }, { tintColor: tintColor }]} /> ), }
Here instead of the image, you can use an icon from native-base
or vector-icons
.
Here the initial route is used to tell the navigator which page to load first by default using initialRouteName: ‘DHome’
as the second object.
4: — Tab Navigation
We will reach Tabs Navigation by clicking on the button to navigate in Drawer pages. For Tab Navigation, we use createBottomTabNavigator
method to create tab navigation in our app.
Code for tab navigation are as: —
And The output is as: —
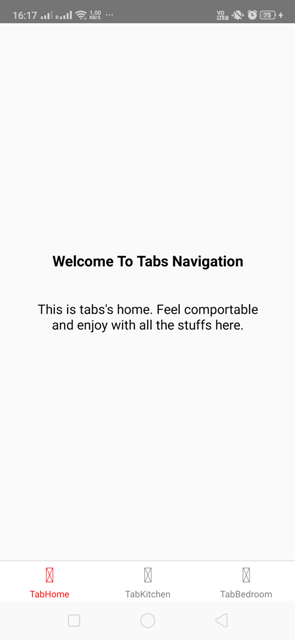
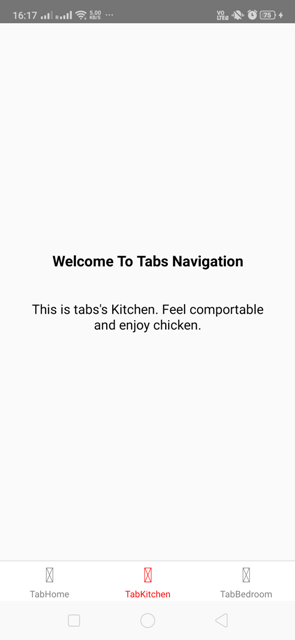
I have created multiple pages for each tab, drawer and stack navigations to navigate from one page to another and my folder structure is like this.
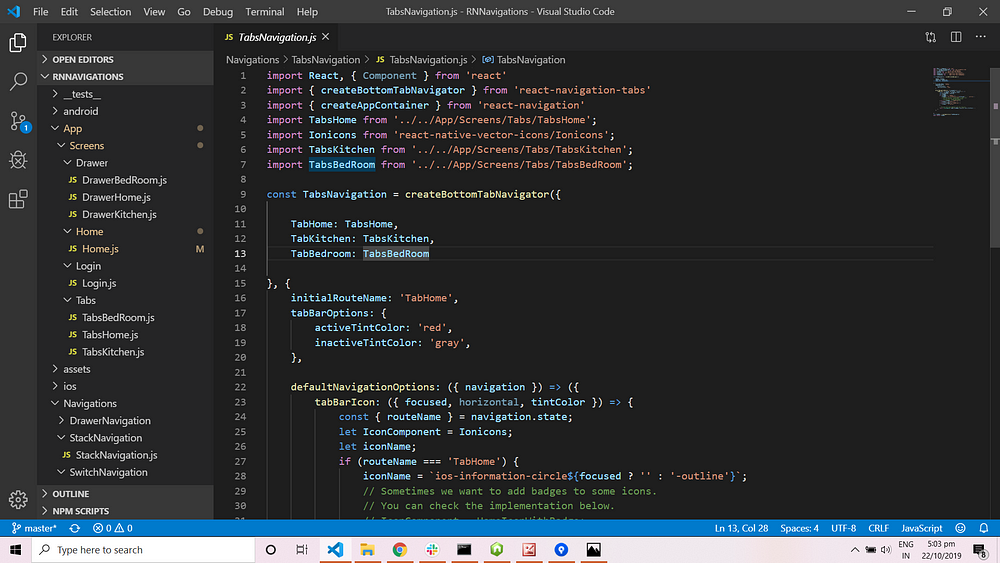
For more detailed options For Tab Navigation, you can check out this link.
Here in the above tab example, we have used tabbarOptions
and initialRouteName
as
{ initialRouteName: 'TabHome', tabBarOptions: { activeTintColor: 'red', inactiveTintColor: 'gray', },
because initialRouteName
tells the app, which page to load first by default when tab component loads and in tabBarOptions activeTintColor
is used to specify the color to the currently active tab and inactiveTintColor is used to specify the color for rest inactive tabs.
The Most important Tip and Trick in react Navigation is that never use very nested navigation because it will create problems while passing parameters and as a result, you will not be able to receive any params using getParam() method and this will not throw any error too.
Conclusion
In this post, we learned how to implement navigations using react-navigation in our cool React native app. We also learned how to handle different types of navigations methods and use their data in the app.
With this tutorial, we also achieved all the navigation methods in the same application.
Complete source code of this tutorial is available here — RNNavigations