How to pick images from Camera & Gallery in React Native app
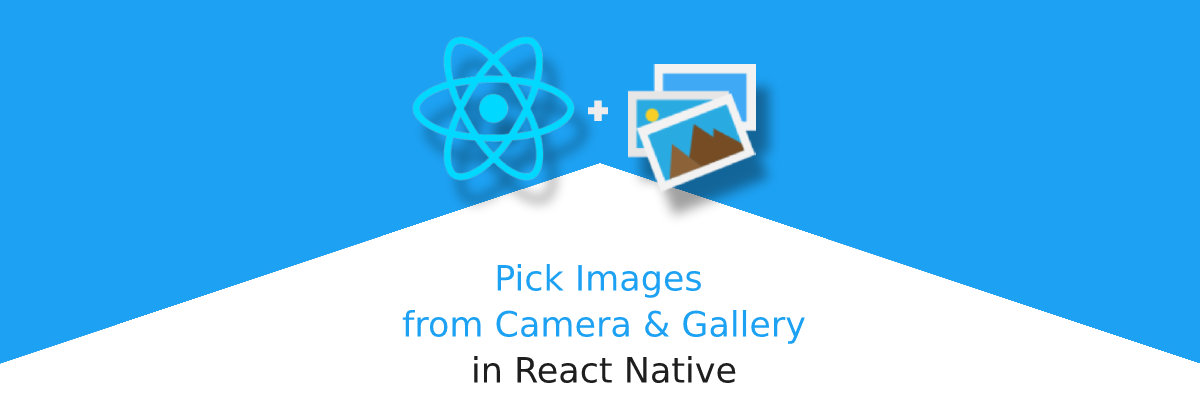
If you would like access to the entire source code, you can check out the GitHub repo here. For step-by-step tutorial, continue reading below.
Picking images from Gallery and Camera is one of the most important and basic functionalities that is required in almost all the apps. Advanced functionalities are built upon this basic core facility. For picking the image in React Native, we will use a popular and superior library called react-native-image-picker
. This provides the ImagePicker component in which you can provide the image picking option from Gallery or Camera.
We’ll follow a stepped approach to create an ImagePicker app in React Native. Following are the steps
- Step 1 — Create a basic React Native app
- Step 2 — Set up React Native Image Picker
- Step 3 — Use React Native Image Picker to pick images in app
So let’s dive right in!
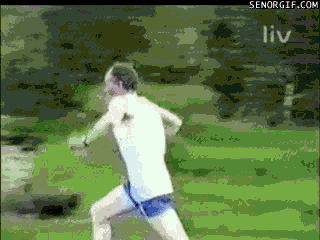
Step 1 — Create a basic React Native app
Getting started with React Native will help you to know more about the way you can make a React Native project. We are going to use react-native init
to make our React Native App or we can run npx react-native init
.
Assuming that you have node installed, you can use npm to install the react-native-cli
command-line utility. Open the terminal and run the following command to the bottom
npm install -g react-native-cli
Now, let’s create a new React Native project, e.g. RNimagePicker
react-native init RNimagePicker
When the above command is done, open the main folder in your preferred editor. When we open the project in a code editor, its structure looks like this.
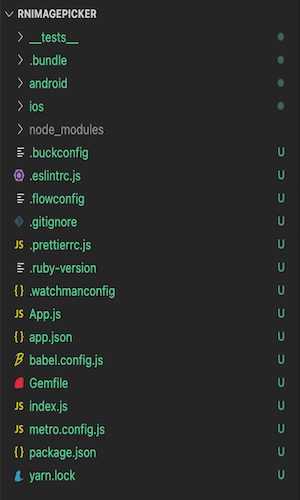
Step 2 — Set up React Native Image Picker
To use React Native Image Picker we need to install react-native-image-picker
dependency.
Installation of Dependency
To add React Native Image Picker to our React Native Project, we need to run the following command at the root of the project
npm install react-native-image-picker --save
or
yarn add react-native-image-picker
This command will copy all the dependencies into your node_modules directory, You can find the directory in node_modules
the directory named react-native-image-picker
Methods provided by react-native-image-picker
launchCamera()
method launch the camera and helps us to take the photo. It returns the file data with different attributes like URI, base64 URL and other fields. More on this later.
launchImageLibrary()
methods launch the Photo library and we can select photos and videos. It returns the file data with attributes like URI, base64 URL. More on this later.
Permissions
Android :- No permission needed for android.
iOS :- Adding appropriate keys to the Info.plist,
If you are allowing user to select image/video from photos, add NSPhotoLibraryUsageDescription
.
If you are allowing user to capture image add NSCameraUsageDescription
key also.
If you are allowing user to capture video add NSCameraUsageDescription
add NSMicrophoneUsageDescription
key also.
Step — 3 How to Use React Native Image Picker
React Native Image Picker library provides methods in which you can set the options like the title of the picker, your custom buttons (name and title of the button) and storage options like skipBackup, etc.
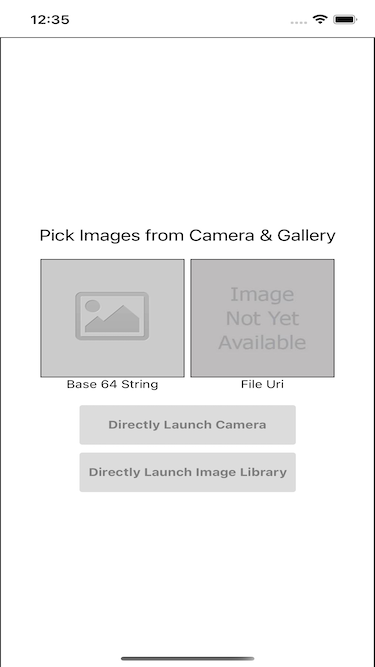
Launch Camera
To implement the functionality of launching the Camera as you click on “Direct Launch Camera”, and use the code below. It will open the camera and will show the clicked image on the Image Component.
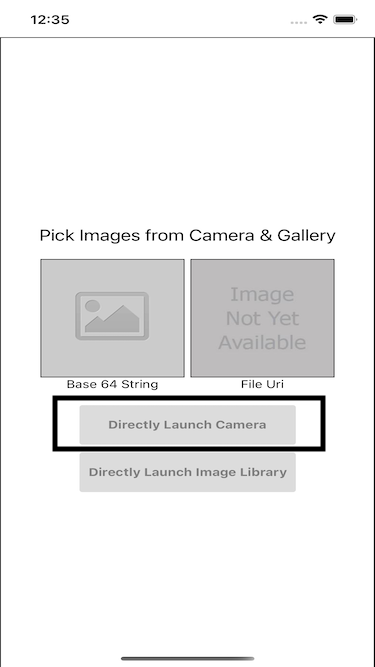
Launch Image Library
To Launch the Image Library you can use the following code below. It will directly open the Image Library and will show the Selected image on the Image Component.
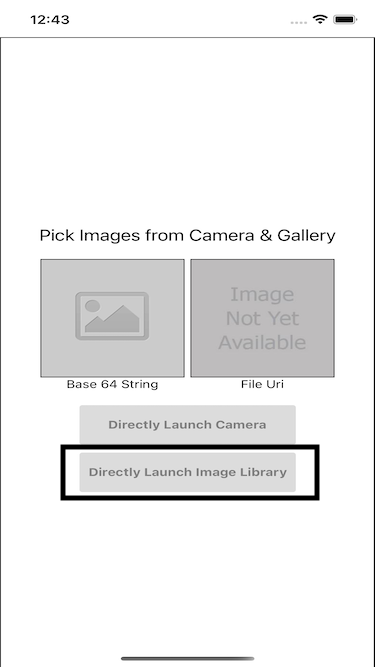
App.js
Below is the explanation over methods used in appImagePicker.js file: -
- launchNativeImageLibrary [Line 83 in above code] :- This function is responsible to set the options (for image picker) and call the image picker method to open native library to select the image. This function is called on “Directly Launch Image Library” [Line 130].
- launchNativeCamera [Line 58] :- This function is responsible for setting camera options and calling image picker method to open camera. This function is called on “Directly Launch Camera” [Line 126].
- renderFileData [Line 30] :- This function is responsible for creating a base64 URL, that can be used to show the images in Image tag (image view).
- renderFileUri [Line 43] :- This function is responsible for using the file URI, that can be used to show the images in Image tag (image view).
This React Native module allows you to use native UI to select a photo/video from the device library as well as from the camera directly.
Your Screen should look like this:
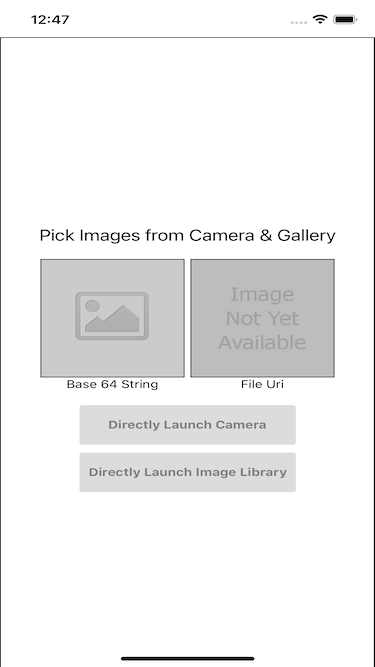
iOS Simulator screens
Finally, for your reference all the screens for this image picker functionality will look similar to those shown below:
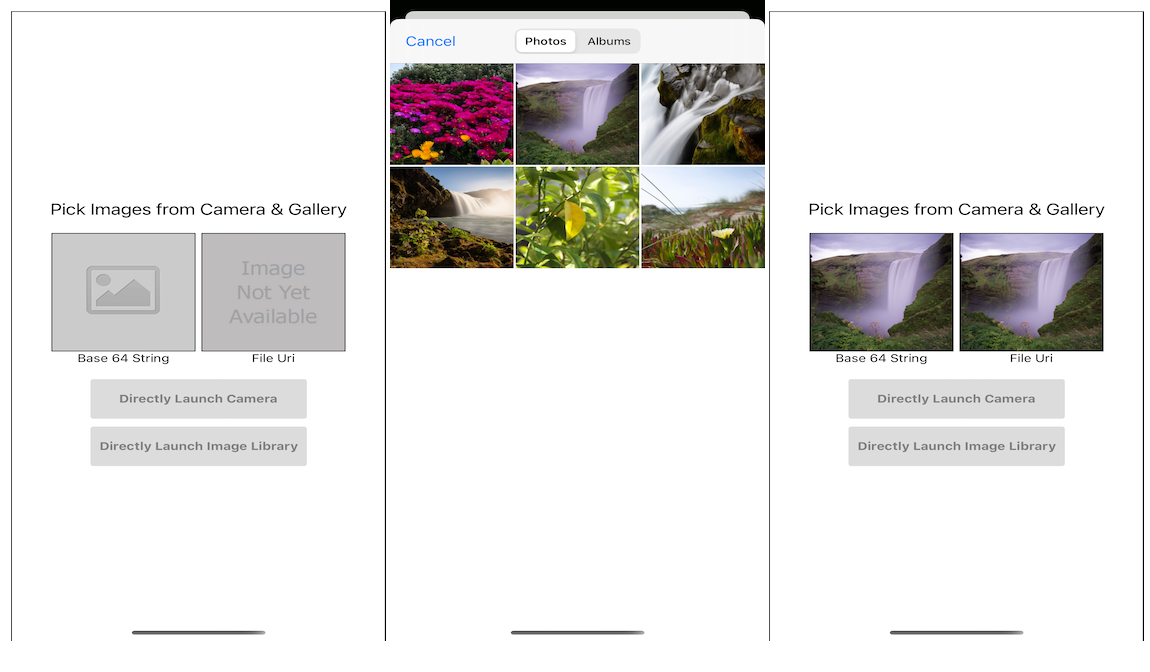
For iOS simulator, opening Camera won’t support but it will work on Real device.
React-native-image-picker vs Expo ImagePicker
As a final note, if you wondering about if this approach work in Expo also, the answer is — not exactly. you will need to use expo Imagepicker for that expo-image-picker
Conclusion
In this post, you learned in a quick fashion to implement React Native Image Picker in your React Native App. This enables your app to pick images/videos from the Camera and Gallery. It is one of the most important and basic functionalities that is needed in almost all the apps.
Still, Image Picker not working in React Native app, why? For this, you can find the complete code in this Github repo over here.
Next Steps
If you liked this blog, you will also find the following React Native blogs interesting and helpful. Feel free to ask any questions in the comment section
- Firebase — Integrate Firebase | Analytics | Push notifications | Firebase CRUD
- How To in React Native — Geolocation | Life cycle hooks | Image Picker | Redux implementation | Make API calls | Navigation | Translation | Barcode & QR code scan | Send & Read SMS | Google Vision | Pull to Refresh
- Payments — Apple Pay | Stripe payments
- Authentication — Google Login| Facebook login | Phone Auth | Twitter login
- Create Instagram / Whatsapp Story Feature in React Native
- React Native life cycle hooks | Implement Redux | Async actions with Redux
- Create Awesome Apps in React Native using Full App
If you need a base to start your next React Native app, you can make your next awesome app using React Native Full App
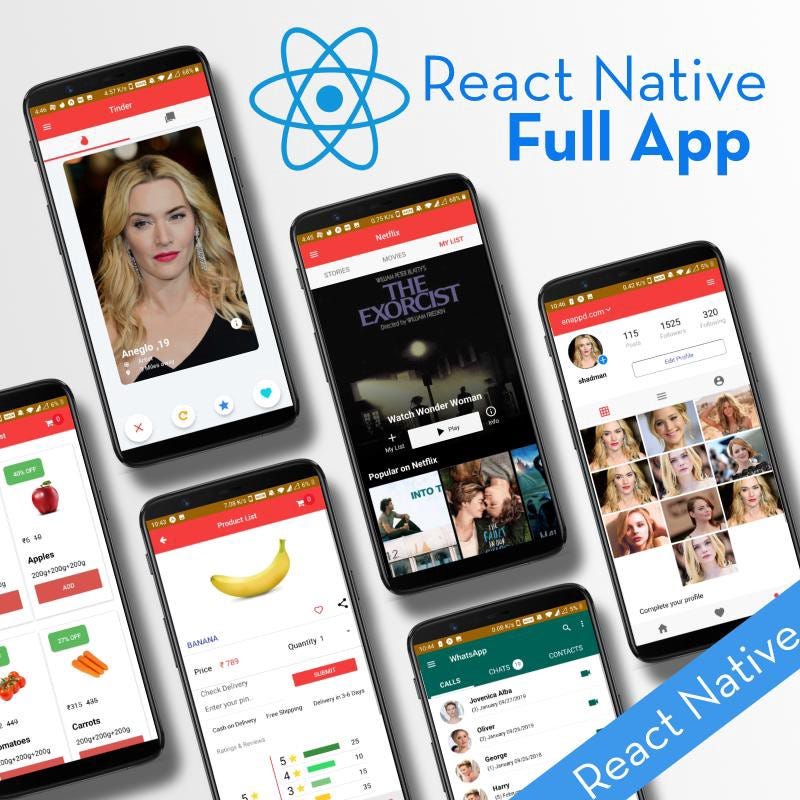