React Native API calls with Fetch and Axios
In this tutorial, we will implement most popular ways for API calls into React Native application.
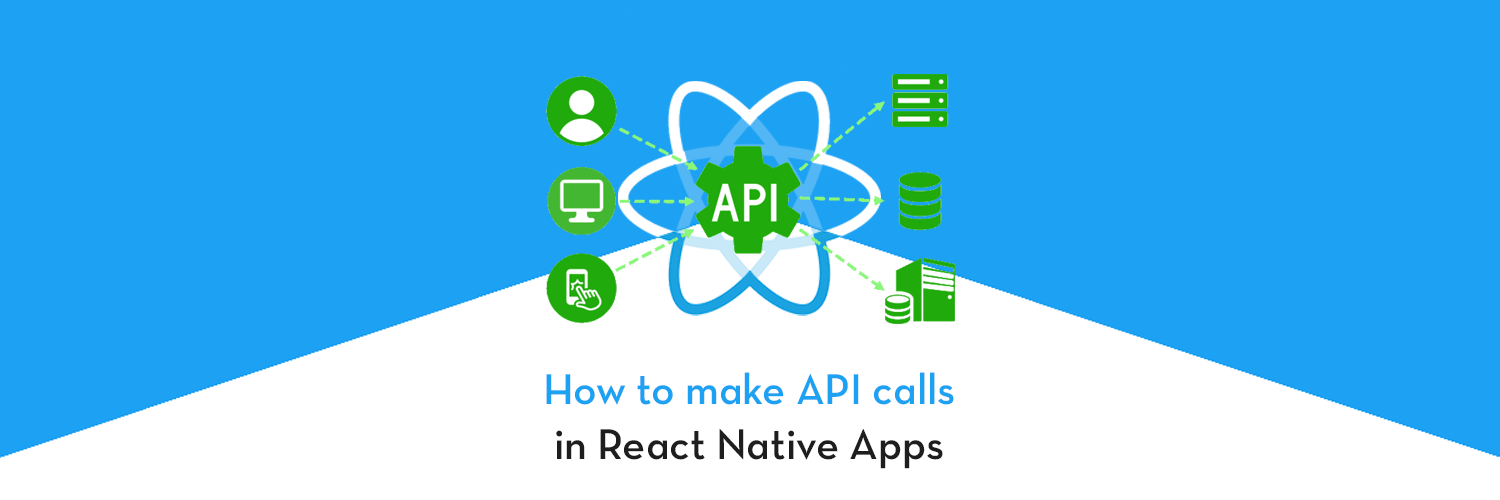
We will build a project and along with it learn different methods for API calls including 1) fetch()
method and 2) Axios
method provided by React-Native. We will also see the different configurations and properties of these methods using fetch
and axios
in react native applications.
Complete source code of this tutorial is available here — RN-API__Methods
What is React-Native?
TLDR; — React Native (RN) creates cross-platform apps, more “native” than web-view apps made by Cordova / Ionic. But React Native is yet to release a stable (1.0.0_) version of the framework.
React Native is a JavaScript framework for writing natively rendering mobile applications. It’ is based on React, Facebook’s JavaScript library for building user interfaces, but instead of targeting the browser, it targets mobile platforms. Because most of the code you write can be shared between platforms, React Native makes it easy to simultaneously develop for both Android and iOS.
React Native applications render using real mobile UI components, not web-views, and will look and feel like any other native mobile application. React Native also exposes JavaScript interfaces for platform APIs, so your React Native apps can access platform features like the phone camera, or the user’s location. Facebook, Palantir, TaskRabbit etc are already using it in production for user-facing applications.
Why Do We Need APIs?
Most web and mobile apps store data in the cloud communicate with a service. For example, a service that gets the current weather in your local area, or returns a list of GIFs based on a search term.
Databases and web services have something called an API (Application Programming Interface) which apps can use to communicate with through a URL. In other words, an API allows apps to retrieve or send data to and from databases or web services.
Apps use HTTP requests, for example, GET, POST and PUT, to communicate with APIs.
React Native and API Calls
Many mobile apps need to load resources from a remote URL. You may want to make a POST request to a REST API, or you may need to fetch a chunk of static content from another server.
Post structure
We will go in a step-by-step manner to explore all the methods for API calls. This is my break-down of the blog
STEPS
- Create a simple React Native app
- Install package
axios
. - Use
fetch
andaxios
methods for API calls.
Let’s dive right in!
Step 1 — Create a basic React Native app
First, make sure you have all pre-requisites to create a react-native app as per the official documentation.
At the time of this post, I have React-Native version 0.69.3
Create a blank react-native app (Replace APICALLS
with your own app name)
$ react-native init APICALLS
This will create a basic React-native app that you can run in a device or simulator. Let’s run the app in iOS using
$ react-native run-ios
You’ll see the default start screen on the device/simulator.
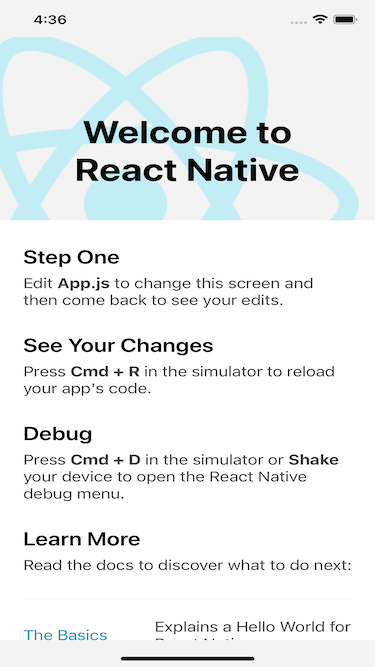
Step 2— Install Axios in React Native App
You can use following command to install Axios :
yarn add axios
# or with npm
npm i axios — save
Right now my App.js structure looks like :-
I have used Hooks and implemented the concept of functional component in this app as ApiContainer.js
In this ApiContainer.js I have imported the Axios library at the top of the file.
import axios from 'axios';
Let’s get started and start implementing these methods in our cool React-Native application but before that let us make some buttons for UI purpose so that we can click those to get responses and show the result. I am providing the completed code of ApiContainer.js later in blog. Right now lets understand Fetch and Axios methods.
Step 2— Fetch vs Axios for API calls
We have made a UI as shown below to call these 2 methods from 2 different buttons. [Code is later in blog ]
View Results: —
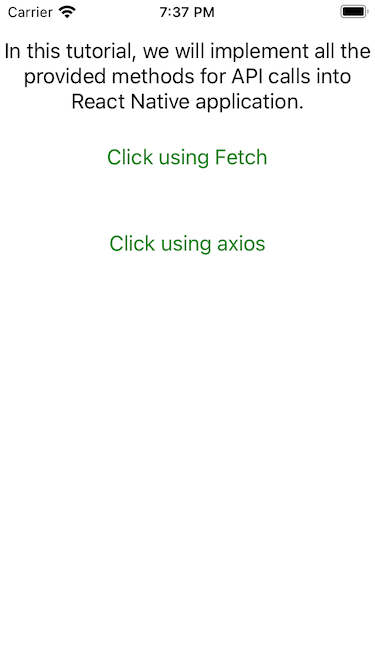
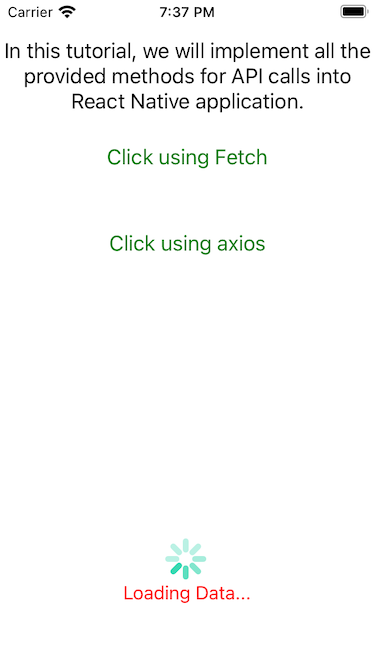
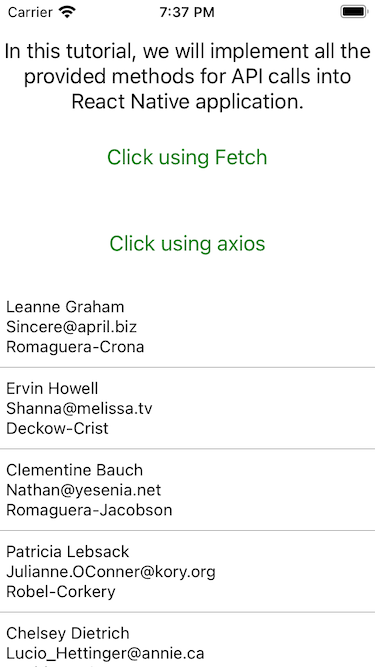
Fetch API
React Native provides the Fetch API for your networking needs. Fetch will seem familiar if you have used XMLHttpRequest
or other networking APIs before.
Making requests
In order to fetch content from an arbitrary URL, you can pass the URL to fetch:
fetch('Your URL to fetch data from');
Fetch also takes an optional second argument that allows you to customize the HTTP request. You may want to specify additional headers, or make a POST request:
fetch('Your URL to fetch data from', {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
firstParam: 'yourValue',
secondParam: 'yourOtherValue',
}),
});
Networking is an inherently asynchronous operation. Fetch methods will return a Promise that makes it straightforward to write code that works in an asynchronous manner:
function getMoviesFromApiAsync() {
return fetch('Your URL to fetch data from')
.then((response) => response.json())
.then((responseJson) => {
return responseJson.movies;
})
.catch((error) => {
console.error(error);
});
}
Using async
/await
syntax in React Native app:
async function getMoviesFromApi() {
try {
let response = await fetch(
'Your URL to fetch data from',
);
let responseJson = await response.json();
console.log(responseJSon);
return responseJson;
} catch (error) {
console.error(error);
}
}
Don’t forget to catch any errors that may be thrown by fetch
, otherwise, they will be dropped silently.
Code View for Fetch Method: —
Adding Authorisation Header in fetch Request
Some times we need Authorization header, if app is behind a login based system.
To add authorisation header in fetch API, we have to add headers attribute and pass it as second parameter in fetch API.
fetch('url', { method: 'GET', headers: new Headers({ 'Authorization':
`Bearer ${token}`,
'Content-Type': 'application/json',}), });
Axios Library
Another method to API Call is Axios. Axios is a hugely popular HTTP client that allows us to make different requests (GET, POST, PUT and DELETE) from the browser.
Axios has defined different inbuilt methods to call each method type. Below are those inbuilt methods :-
- POST method → It is called using
.post()
method. It takes url, data and header as its arguments. - GET method → It is called using
.get()
method. It takes url and header as arguments. - PUT method → It is called using
.put()
method. It takes url, data and header as arguments. - DELETE method → It is called using
.delete()
method. It takes url and header as arguments.
Now, we will show use of Axios with React Native to make requests to an API, use the returned data in our React app.
Code View for Axios Method: —
Code View for APIContainer.js
Code View for APIStyles.js
In the above gist , you can see I have used the setTimeout method for data to be loaded — and while it loads I will show a loader.
Adding Authorisation Header in Axios Request
To add the Authorisation header in axios get()
method, we just have to pass second argument as headers. Below is the code, which can be used to add authorisation header in Axios.
axios.get( 'url', { headers: { 'Content-Type': 'application/json', Authorization: `Bearer ${token}`, // auth token }, } ).then((response) => { console.log(response) // handling response })
Axios vs Fetch comparison :
Both Axios and fetch are good in their own way, but I will explain their pros over each other.
Axios pros over fetch
- We do not have to handle request and response headers, as in fetch for each method type we have to set header values.
- Response is in readable format rather than in fetch we have to use json() or other methods to make it in readable format.
- API Calling is easier with axios, as we just have to call post(), get(), delete() or put() method, rather in fetch we have to pass params in fetch API itself.
Fetch pros over axios
- Fetch is inbuilt JavaScript API, that is more Standard than Axios (3rd party package)
- We do not have to import fetch from any package, rather Axios needs to be imported for axios package.
Which is Better — Axios or Fetch ?
In regards to React Native, Axios will be my first choice as it’s easier to use. Axios already handles some of corner cases by it self as it is developed over fetch API by some of the expert developers.
Complete source code of this tutorial is available here — RN-API__Methods.
Conclusion
In this post, we learned how to implement REST API’s using fetch() and axios methods in our React native app. We also learned how to handle responses from these methods and use their data in the app.
We have also seen How to send Authorisation header from Fetch API and How to send Authorisation header from Axios Library in React Native.
Complete source code of this tutorial is available here — RN-API__Methods.
Next Steps
If you liked this blog, you will also find the following React Native blogs interesting and helpful. Feel free to ask any questions in the comment section
- Firebase — Integrate Firebase | Analytics | Push notifications | Firebase CRUD
- How To in React Native — Geolocation | Life cycle hooks | Image Picker | Redux implementation | Make API calls | Navigation | Translation | Barcode & QR code scan | Send & Read SMS | Google Vision | Pull to Refresh
- Payments — Apple Pay | Stripe payments
- Authentication — Google Login| Facebook login | Phone Auth | Twitter login
- Create Instagram / Whatsapp Story Feature in React Native
- React Native life cycle hooks | Implement Redux | Async actions with Redux
- Create Awesome Apps in React Native using Full App
If you need a base to start your next React Native app, you can make your next awesome app using React Native Full App
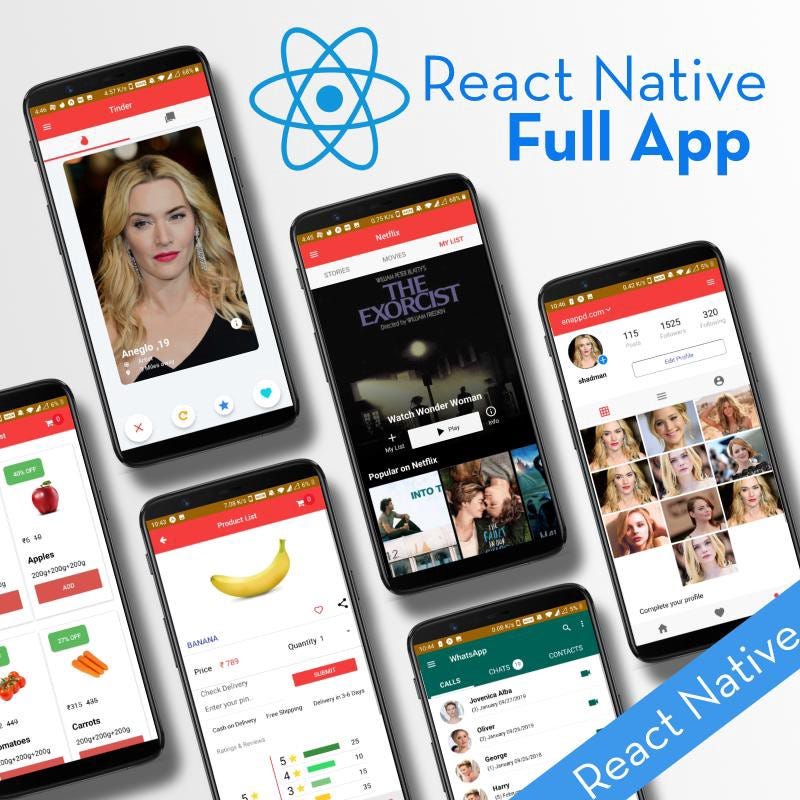