Redux for Beginners — Zero to Hero
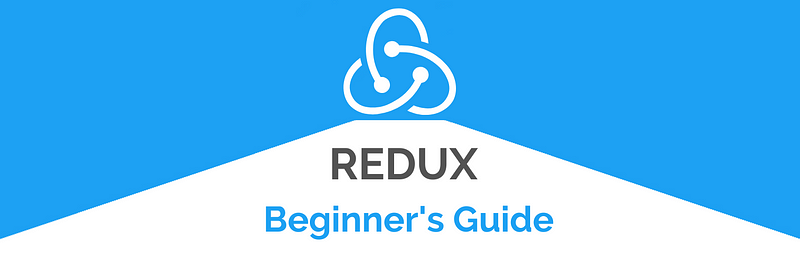
In this article, we will learn basic Redux concepts which you can apply to any application. You can apply it to React or any other framework. Redux is a state management tool independent of React. In an upcoming blog, we will learn how to use this with React.
The complete video content of this blog is here :
You can also check the Hindi version of this Video
Why Redux is used in React?
Let’s start with an example of a React-like application structure. Any application in React has this kind of multi-level component hierarchy.
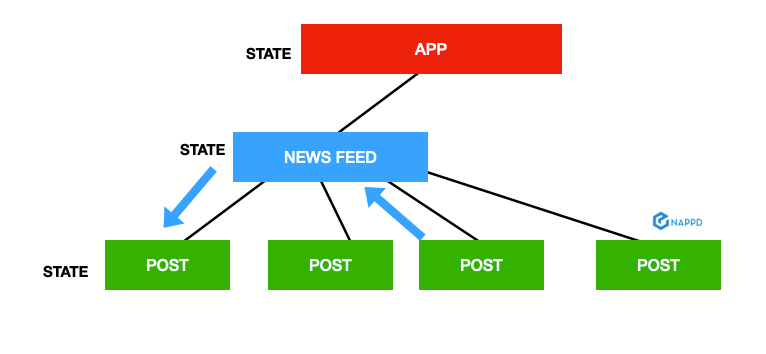
There is an App component at the top, then we have a news feed component, and then some post components. So we have taken a Facebook kind of architecture. In each of these components, you have States. Generally, when we update the state you get a new UI. Now updating the state some events can be called in two ways. Data can flow from top to bottom, which is very common in react and quite easy also — through props. The other way is to change the bottom to top data, in which we pass some functions through props — which is kind of complex.
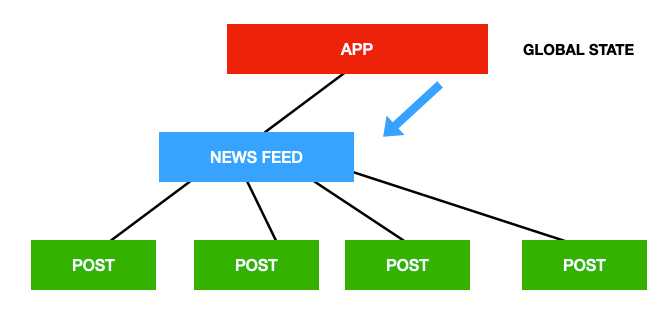
Now Redux changes this whole scenario. And with Redux we want to have a global state at the top. This Global state is easy to change with redux and easy to pass on to lower components through the downward data flow. Downward data flow, as you know — we do through props and that is quite easy to do in react or any other language.
When should we use Redux?
Now let’s see when redux is needed. There are some scenarios in only which you should use redux :
- When your app is moderately complex or overly Complex :). If you have a very simple app you should not use redux.
- The other scenario is when you have a lot of components in your hierarchy. If you have a lot of component levels then obviously it is good to have Stated at the top using redux
- Also if you have a lot of changes in State going on you should use Redux. If you have no changes in the state or very rare changes, It’s not useful to use redux. So change in state frequency also affect your use case.
Redux Bank Analogy
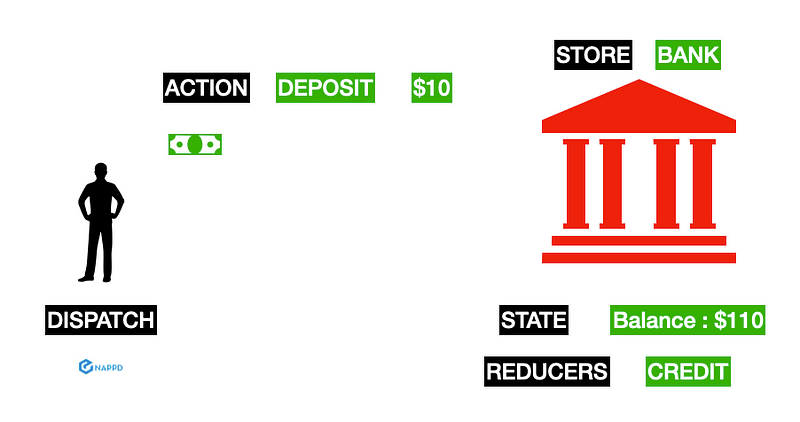
Now let’s take a simple analogy of a Bank and a user. You go to your bank and suppose you have a balance of $100. Now let’s say you want to deposit some money in the bank. The deposit is the kind of action you want to take. You deposit say $10 in the bank. This procedure is called credit in bank terms. Because you have been credited with $10. And now your balance is updated to $110. Now let’s see this whole system in the Redux way. Your bank is the Redux Store where everything like records and procedures is stored. Your balance record is Redux State. The state can be your other information also but in this case just to simplify, it is the balance of $100. Now Dispatch is a method that has sent an Action. Here dispatch is your manual visit to the bank counter. And Action you have taken is called deposit action. The amount you have deposited is also a kind of data associated with the action. Now the bank has some procedures like credit procedures, which will be called Reducers in Redux terms.
Now you can see with any deposit Action of $10, the balance becomes $110. So this is an update to a new balance or you can say new State.

Let’s take another scenario, in this scenario you want to take some money out of the bank. so you dispatched different Action called withdrawal to withdraw $10. Suppose your State or your balance right now is $110. There will be a Reducer called debit which will run and it will cut $10. Now your next balance is $100. So Reducer works on 2 inputs 1) your action, and 2) your previous state, and gives out a new State. Later in the code, we can see, how to use this reducer with those inputs — action, and state to give a new state.
So let’s revise all the concepts which we have learned till now.
You have a Store, in which you have a State like your account info.
In Store, you also have Reducers — which can be different procedures like credit or debit.
You have dispatch also. In our case dispatcher is someone who goes to the bank. In real applications, it will be events — like the onclick function. All those things are the dispatcher.
Then action can be of 2 types deposit or withdrawal. There is an amount also with these types. So, deposit and withdrawal are called Action types, and this amount we called an Action payload.
Redux Terminology: Javascript way
Now let’s understand the terms in a JavaScript program context.
State is a global state object of your app. Like a nested Javascript object. Right now assume that the only state you have is balance.
An Action is an object that has a type field like a deposit here. And the payload is 10.
Reducers are functions that receive two things — the current state and action object. Depending on that, they give you a new state.
Store is the container in which you have all the reducers and because reducers work on actions you can say the store contains everything inside it.
Dispatch is a way in which you call the actions. The store has a dispatch method that can be used to dispatch some action.
Redux: How do things work?
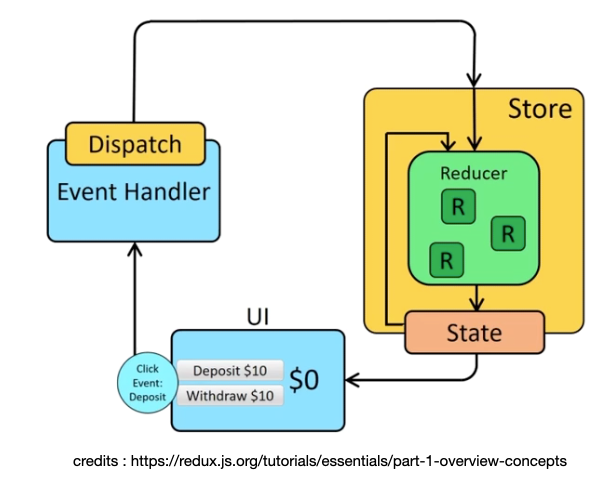
Now let’s understand the sequence of things going on in an app. We have taken this diagram from the official redux documentation. Here you can see on the right side there is a store and in that there are 3 reducers and also there is a state which is attached to the store. Also, we can see the UI below. It has deposit and withdrawal, two buttons. When you press these buttons — an event will occur. There is an event handler shown in this diagram. In the handler, we always call the dispatch method of Store, which dispatches an action.
Let’s see all these things in action. Everything starts from UI. When a user comes it presses some button, which generates an event. This event goes to the event handler. The event handler dispatches action and action comes to the reducer. Inside the reducer, action work alongside with previous state and provides a new state. Like here the previous state was $0 and the action added $10, Now the new state is updated with $10. Now the UI shows a new balance.
Redux: Code Example
We have understood the architecture and workings of Redux. Let’s jump into some simple code.
So here is a simple JavaScript file Index.js and it is attached to an index.html.
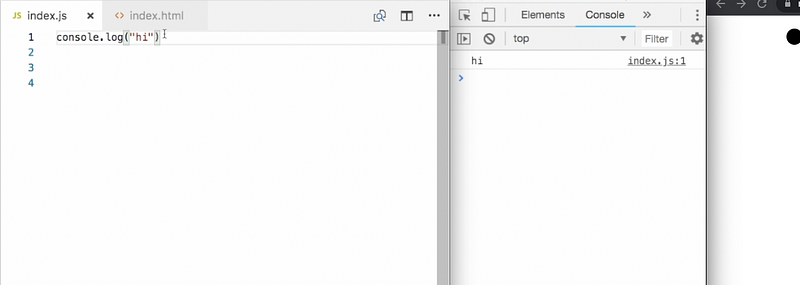
Side by side in the console we are getting the output. The console is printing “hi” right now.
Installing Redux
To install the Redux package through:
npm install redux // or yarn add redux
How to Create a Store in Redux?
I am importing the first thing from the redux package, it is called createStore
import {createStore} from 'redux';
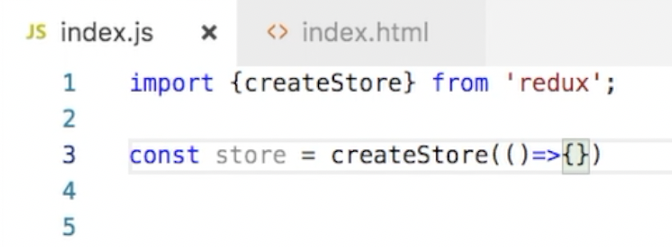
Now using createStore, let's create a store. You can put an empty object, but it will throw an error because it requires a reducer and reducers are functions. So, I put an empty arrow function. This will not throw an error but right now this is also not valid because there should be some valid reducers. We will correct it later.
How to Create an Action in Redux?
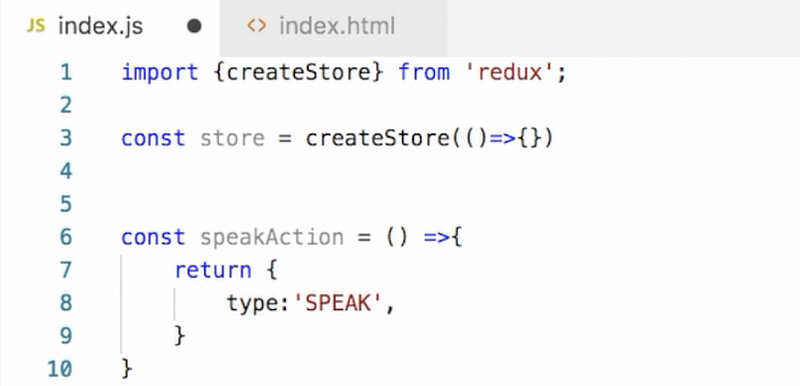
Let’s move to the next thing and we will create some kind of action. So I’ll say SPEAK action and this is an again arrow function, this is actually an Action Generator — it returns an action object. What it returns must have a type and a payload. The type we put in capitals and right now there is no payload, just to make this simple.
How to Create a Reducer in Redux?
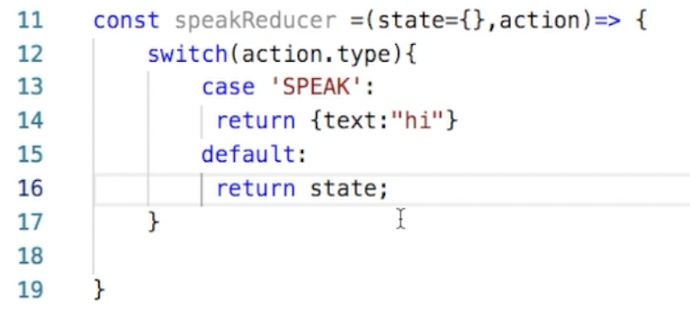
Now I’ll create a speak reducer. As we see there are two inputs — state, with the default state taken as a blank object, and action. We will put the switch block, and in the switch, you can write action.type. In the first case in the switch, we will write as speak. In this case, we will return some value. So I’ll just return— {text: ‘hi’}. I am kind of rewriting the whole state itself which is not the best usually. Generally, we take the previous state and modify it but just for this example I am doing this. Finally, the default case is returned which will return the unmodified state.
So, in any case, we return to a state. If there is an Action type that matches the reducer returns the state otherwise it returns the default state.
How to use a Dispatch in Redux?
Now let’s move the store down, as the store uses the reducer — and the definition should be earlier to the usage.
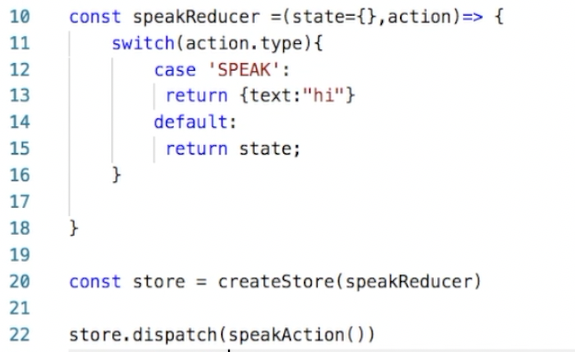
Now you can call store.dispatch and let’s dispatch speakAction. Right now, how we will know what happened after dispatch? As we have not written any code to observe the State changes.
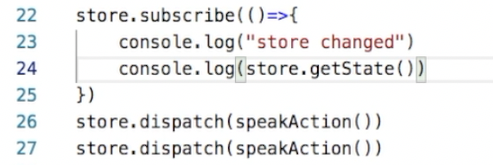
So we will use the subscribe method, where you subscribe to the updates of the store. And in console.log we will write store changed. Whenever there is some change in store your subscription will detect it. So I’ll put dispatch after the subscription. Now see, as the state changes something comes on the console. We can’t see what is changed in the state. For that, we have a getState method. And after that we can check console logs :
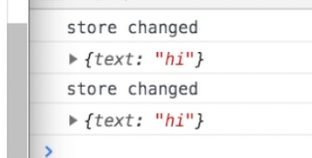
This is not a very meaningful action, because every time we call the action same state comes up — like { text: “ hi” }. So let’s make a change and we put the input to the action generator function.
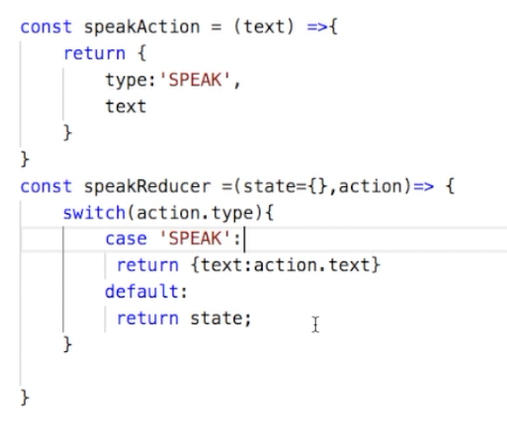
And this text I put as a payload for our action. We have this text passed as object shorthand. In reducer we can pass the value of this payload, and we can write action. text.
Now to test, I pass two different payloads hello and Hey. Now you see there are two different state outputs in the console, for each of these calls.
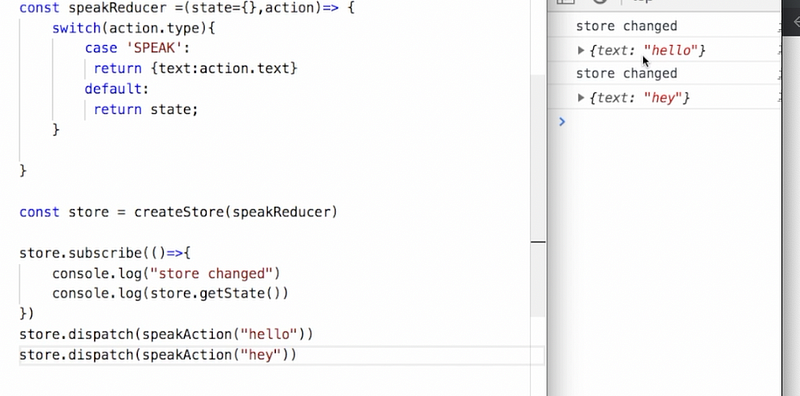
So let’s see the whole process.
- We called this action generator, which has returned an action with a type and text as a payload.
- Now the reducer gets called, in the switch we see the action type, it matches SPEAK type. This returned an object, which is your new state.
- Because we use getState which gives you the Global state store, you can see the changes in the subscribe method console. logs.
Please don’t worry about — how this subscription works, and where we have to put it in real-world apps. Generally in a library like React-Redux, subscription is automatic and you just have to dispatch actions. In the next blog, we will learn about React-Redux. Right now our purpose was to understand the concept of redux and why it is used for state management in any application.
Conclusion :
In this article, we have covered the fundamentals of Redux. After a solid understanding of Redux terms and architecture — you can confidently use it in any application. How to use Redux with React? We will answer this in the next blog on React-Redux library.
Next Steps
If you liked this blog, you will also find the following React Native blogs interesting and helpful. Feel free to ask any questions in the comment section
- Firebase — Integrate Firebase | Analytics | Push notifications | Firebase CRUD
- How To in React Native — Geolocation | Life cycle hooks | Image Picker | Redux implementation | Make API calls | Navigation | Translation | Barcode & QR code scan | Send & Read SMS | Google Vision | Pull to Refresh
- Payments — Apple Pay | Stripe payments
- Authentication — Google Login| Facebook login | Phone Auth | Twitter login
- Create Instagram / Whatsapp Story Feature in React Native
- React Native life cycle hooks | Implement Redux | Async actions with Redux
- Create Awesome Apps in React Native using Full App
React Native Full App
If you need a base to start your next React Native app, you can make your next awesome app using React Native Full App
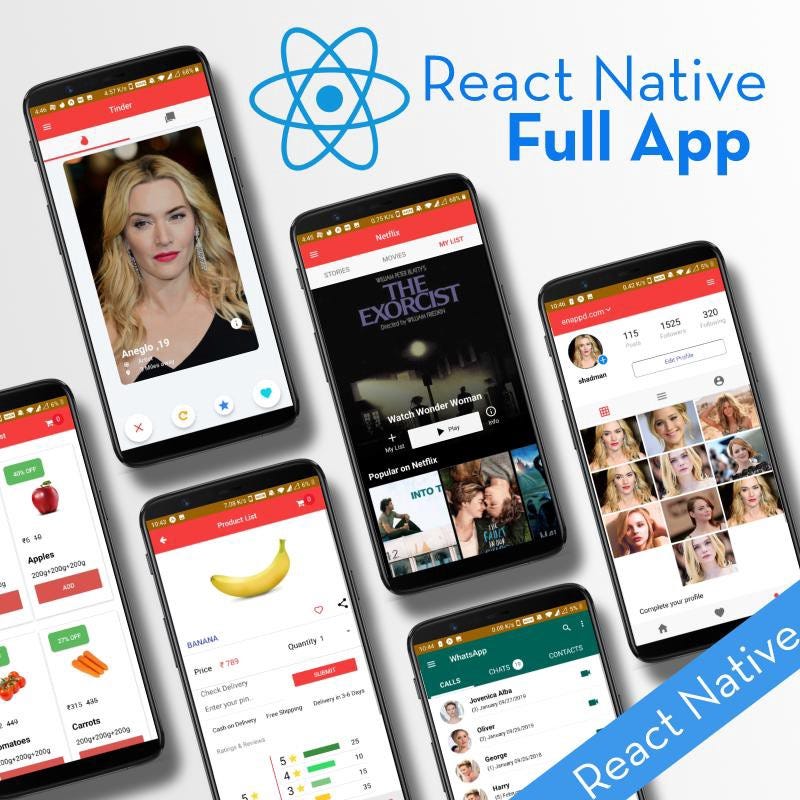
Ionic React Full App with Capacitor
If you need a base to start your next Ionic 5 React Capacitor app, you can make your next awesome app using Ionic 5 React Full App in Capacitor
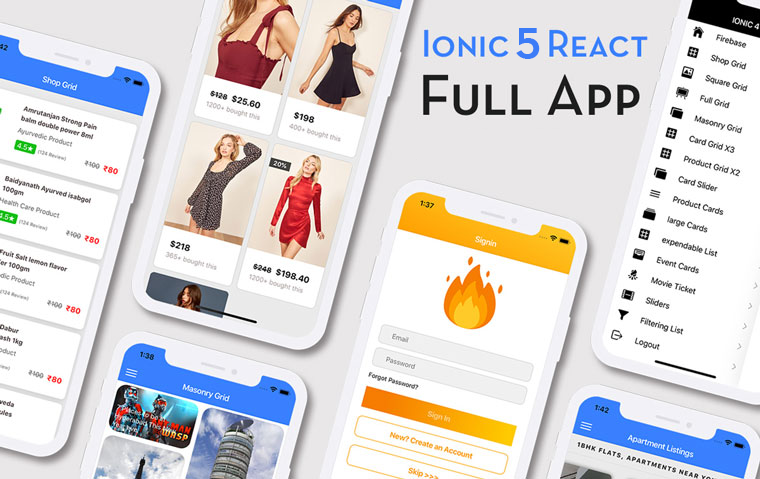