Beginners Guide to Firebase Database, Auth, and Hosting — Using Ionic Angular App
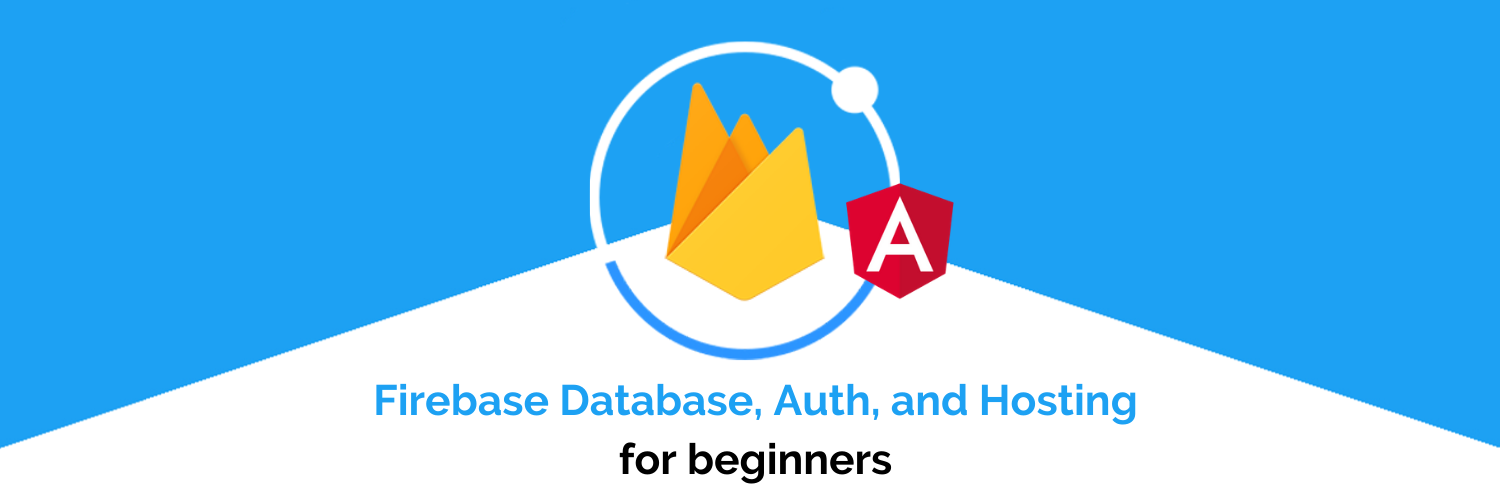
Firebase is a mobile and web application development platform developed by Firebase Inc. in 2011, then was acquired by Google in 2014. Firebase is a toolset to “build, improve, and grow your app”. It gives you functionality like analytics, databases, realtime-databases, cloud messaging, cloud functions (serverless function), user authentication, and crash reporting so you can move quickly and focus on your users.
Today Firebase is one of the fastest-growing platforms for application development. Some of the reasons are
- You don’t need to write a back-end from scratch. Firebase is a ready-made back-end, with a DB attached to it. You can also write some server-side code using the Firebase Cloud Functions, which essentially makes your architecture serverless.
- Cloud Firestore.
Cloud Firestore is a cloud-hosted, NoSQL database that your iOS, Android, and web apps can access directly via native SDKs. Cloud Firestore is also available in native Node.js, Java, Python, Unity, C++, and Go SDKs, in addition to REST and RPC APIs. - It’s R-E-A-L T-I-M-E.
If you are a developer, you understand the importance of a real-time back-end/database in today’s app market. Things like chat, news feeds, ratings, bookings, etc all are very easy if you factor in real-time operations. Firebase has 2 kinds of databases — one is a real-time database built for performance and very minimal data. The second one is Firestore which is also a reliable alternative to the NoSQL paradigm — with a slight change in querying and indexing. - Cloud Functions.
The most useful weapon of the firebase, It helps us to create our own server without worrying about any setup we have to just run some commands and then write our server script. - Simple Authentication operations.
The very first thing required in a user-facing application is login/register operations. Firebase handles this very smoothly and with minimum coding effort. You can integrate a number of social authentication services like Facebook, Google, Email, Phone, etc. with Firebase as well. - You get tonnes of additional features in-built e.g. push notifications, analytics, crash-analytics, etc 😍😍
- It also provides a free and secure hosting solution for your Static files. Like Angular and React-based sites.
- It’s free, up to a certain usage limit. But this is pretty awesome for developers who are trying things, or making MVPs, or even for small-scale app businesses. 🤑
In this article, we will focus on Firebase with Ionic Angular — Hosting, Authentication, and FireStore Database Connection. Firebase has been around since Ionic 1 and has been changing ever since. So here are some of the latest plugins and functionalities you can implement using Firebase with Ionic 6.
1. Firebase Hosting — Ionic 6 App
Hosting or deploying an Ionic 6 app on firebase is a very easy task.
let us see how easy it is…..
Step 1: —
So before starting first, we have to create a firebase project, If you have one then you can jump directly to step 2.
1. Go to https://console.firebase.google.com/
2. Click on add a project and then name your project and click on continue, This will create a fresh firebase project for you.
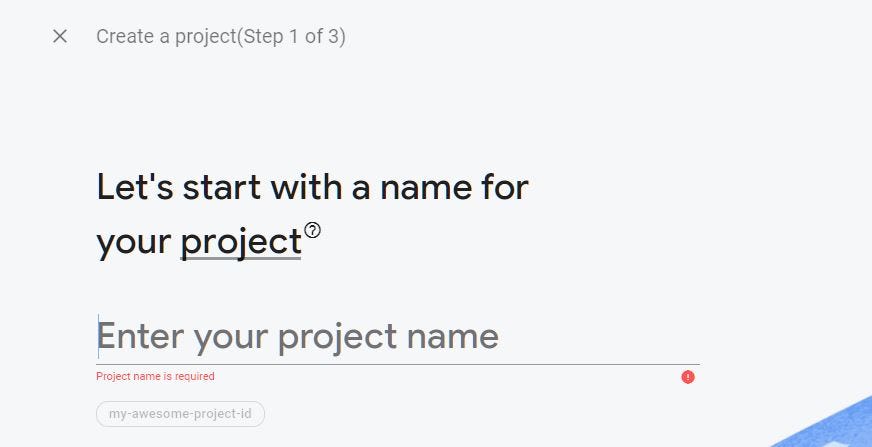
Now in the next step, your project is ready, click on Continue.
Step 2: —
Next, in a Terminal, install the Firebase CLI:
npm install -g firebase-tools
With the Firebase CLI installed, as a first time user, you will need to login into the Google account that you are using with this firebase
firebase login
Finally, run firebase init
within your Ionic project.
Now the dialog will bring up some questions and we need to select Hosting (using space, then hit enter).
The CLI prompts (you can answer these options by choosing up/down arrow keys in terminal/cmd and using the Space bar to select):
“Which Firebase CLI features do you want to set up for this folder?” Choose “Hosting: Configure and deploy Firebase Hosting sites.”
“Select a default Firebase project for this directory:” Choose the project you created on the Firebase website.
“What do you want to use as your public directory?” Enter “www”.
Note: Answering these next two questions will ensure that routing, hard reload, and deep linking work in the app:
Configure as a single-page app (rewrite all urls to /index.html)?” Enter “Yes”.
“File www/index.html already exists. Overwrite?” Enter “No”.
A firebase.json
config file is generated, configuring the app for deployment.
The complete firebase.json
looks like:
{
"hosting": {
"public": "www",
"ignore": [
"firebase.json",
"**/.*",
"**/node_modules/**"
],
"rewrites": [
{
"source": "**",
"destination": "/index.html"
}
],
}
For more information about the firebase.json
properties, see the Firebase documentation.
Step 3: —
Next, build an optimized production version of the app by running:
ionic build --prod
Be sure the output app is available in www
the folder which you mentioned in the config file above. You can also open this folder and check index.html
file by opening it on a local server — it should be the same app you are going to deploy. If for any reason this folder is not created or the available index.html
is not what your application looks like — you might need to check previous steps. Firebase also provides a command called firebase serve
to check applications locally.
Step 4:-
Last, deploy the app by running:
firebase deploy
After the successful deployment, you will see a success message with two links in which one will be your project console and another will be a hosting URL like the below image
Now you can hit the Hosting URL and see your app is hosted on the server.
2. Firebase Authentication in ionic 6—
Ionic has made app authentications too easy for their developer, still not believing? Let us check this out here…
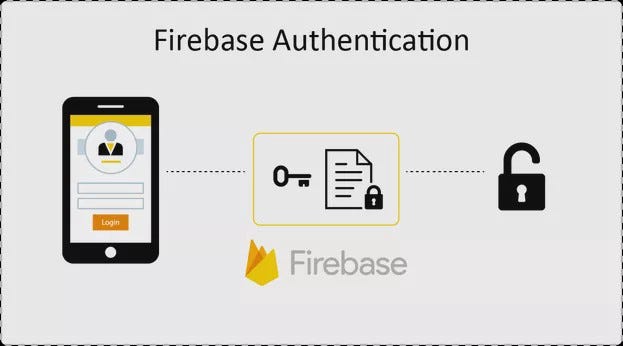
Everyone wants the identity of the user to save their data securely on the cloud to give them a smooth and reliable experience on all of the users’ devices after a single login. Users don’t want to fill the data again and again on every device, so Firebase Authentication provides us with these facilities here.
Firebase Authentication provides backend services, easy-to-use SDKs, and ready-made UI libraries to authenticate users to your app. It supports authentication using passwords, phone numbers, popular federated identity providers like Google, Facebook, Twitter, and more.
You just have to go to Firebase Console and set up your new project, After the creation of the project, you have to select Authentication.
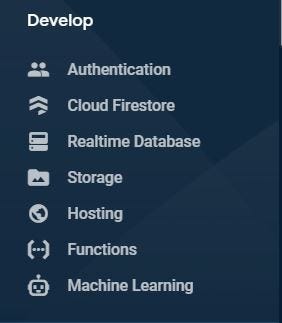
Then you can select Sign-in method And you will see a screen like this-
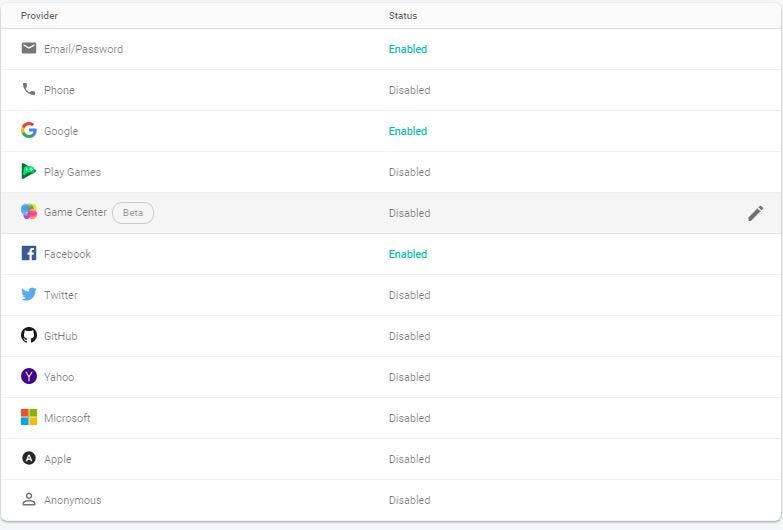
In this part, we are going to see how we can integrate the email/Password, To use all of these services we have to first run the below command and include the library. We have to enable the Email/Password section to work on it.
npm i @angular/fire firebase
Before proceeding, we have to import AngularFireAuthModule in our app.module.ts file. You can check any changes to the Auth Library here
import { AngularFireAuthModule } from '@angular/fire/auth';
And add to the import section of the file.
imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebase), IonicModule.forRoot(), AngularFireAuthModule, AppRoutingModule, IonicStorageModule.forRoot({ name: 'userdb' }), ]
Now we have to import the auth service form angular/fire in page.ts file
import { AngularFireAuth } from '@angular/fire/auth';
And after importing our component we have to inject it and further use its methods for authentication of the users
constructor(private fireAuth: AngularFireAuth) {}
Now we will see how we can log in using email and password using angular/fire.
I. Register a User with Email and Password
this.fireAuth.auth.createUserWithEmailAndPassword(email, password).then(res => { if (res.user) { return(res.user); } else { return(res) }}) .catch(err => { return(err); });
This createUserWithEmailAndPassword function helps us to register a user in firebase, This function helps us to validate various things like if any other user has already registered with the same email then it gives an error, and if we enter an email address that is not in proper form like abc@.com, then it gives an error. Once you have registered using your email and password you can see that in the firebase console.
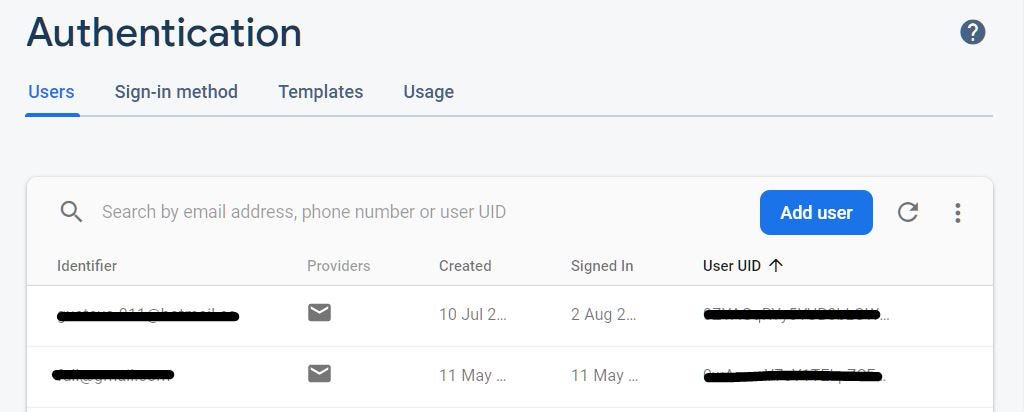
II. Send Password Reset Email
Triggers the Firebase Authentication backend to send a password-reset email to the given email address, which must correspond to an existing user of your app.
this.
fireAuth.auth.
sendPasswordResetEmail() .then((res: any) => console.log(res)) .catch((error: any) => console.error(error));
III. Login with Email & Password
Asynchronously signs in using an email and password.
this.
fireAuth.auth.
signInWithEmailAndPassword(email, password) .then((res: any) => console.log(res)) .catch((error: any) => console.error(error));
This signInWithEmailAndPassword function helps us to check the integrity of the email and password of the user.
IV. Sign-in anonymously
Create and use a temporary anonymous account to authenticate with Firebase.
this.
fireAuth.auth.
signInAnonymously().then(function(userInfo) { // user is signed in });
V. Google login
Uses Google’s idToken and accessToken to sign-in into firebase account. In order to implement it, you can follow our blog.
VI. Facebook login
Uses Facebook’s accessToken to sign-in into firebase account. In order to implement it, you can follow our blog.
VII. Twitter login
Uses Twitter’s token and secret to sign-in to the firebase account. In order to implement it, you can follow our blog.
VIII. Phone login
Use your phone number and SMS verification code mechanism. In order to implement it, you can follow our blog [LINK HERE].
Sign out
Signs out the current user and clears it from the disk cache.
this.
fireAuth.auth.signOut().then(function() {
// user was signed out
});
3. Firebase Firestore Database Connection —
To connect with the database we will be using the same library @angular/fire and using its different methods and components.
npm i @angular/fire firebase
we need to create a new project in Firebase. As we have already created a Firebase project, hence go to the firebase console, where you’ll see the following menu:
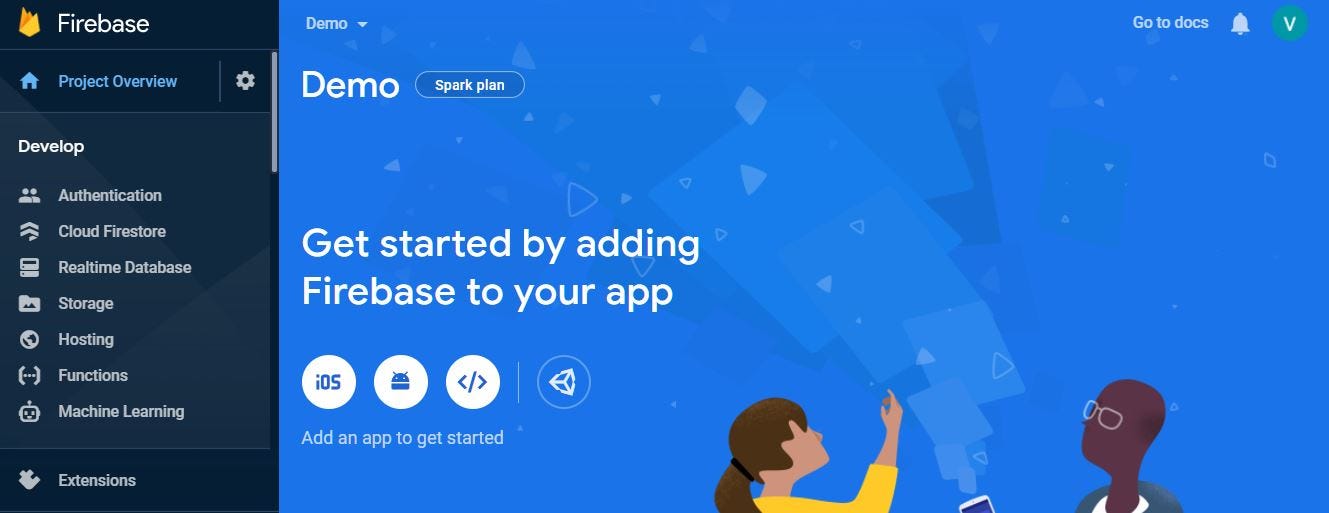
You can click on Add and app to get started to connect your ionic application with Firebase.
It will ask for the Application nickname, which you can put anything you want in my case I am using demoApp.
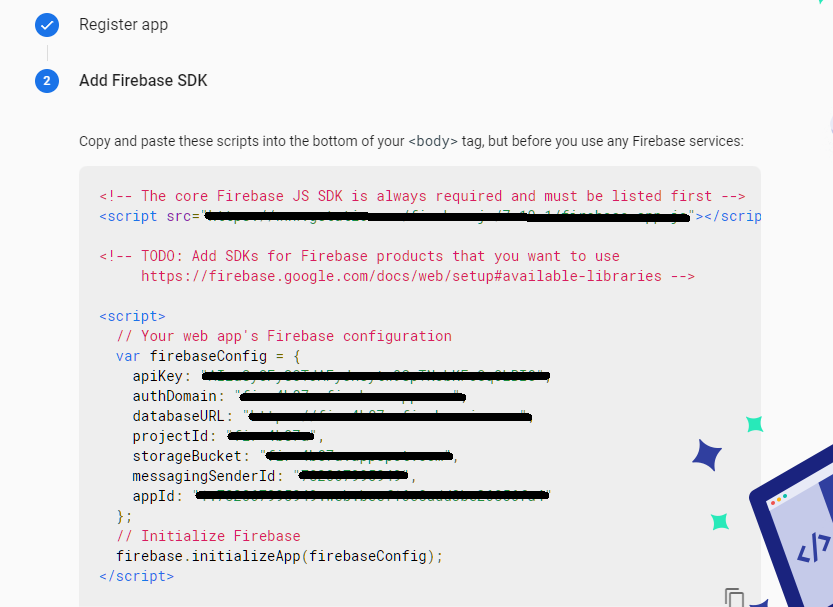
You can copy this firebaseConfig, It helps our application to know about the firebase we will use later on. Now before continuing we have set up the cloud firestore which we are going to use as DB in our application.
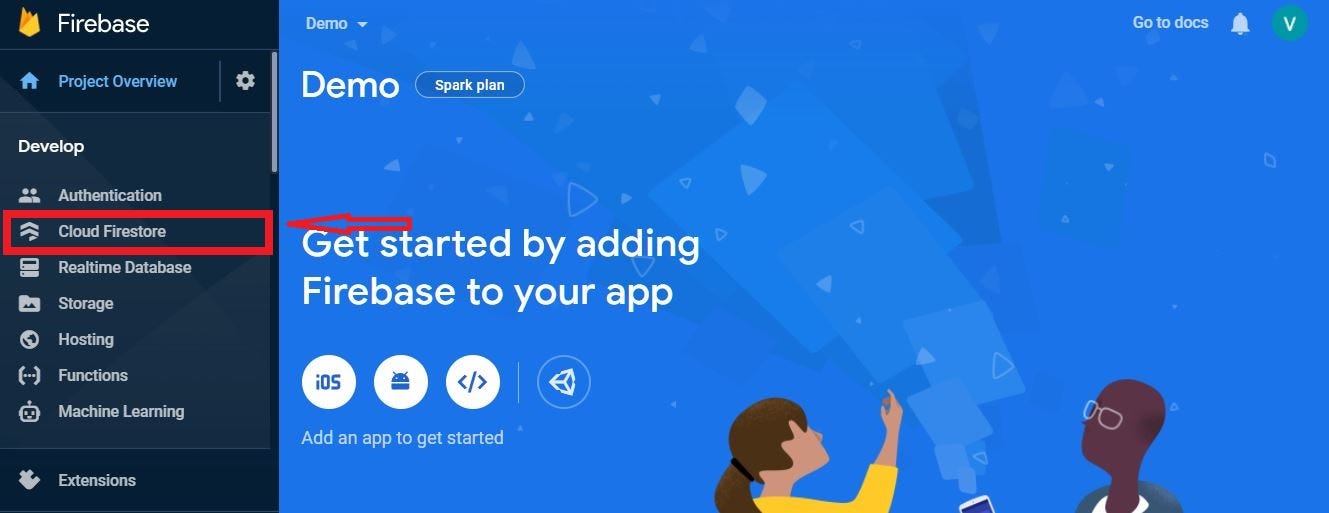
After clicking on Cloud Firestore you will see an option Create Database,then you will two options for the database.
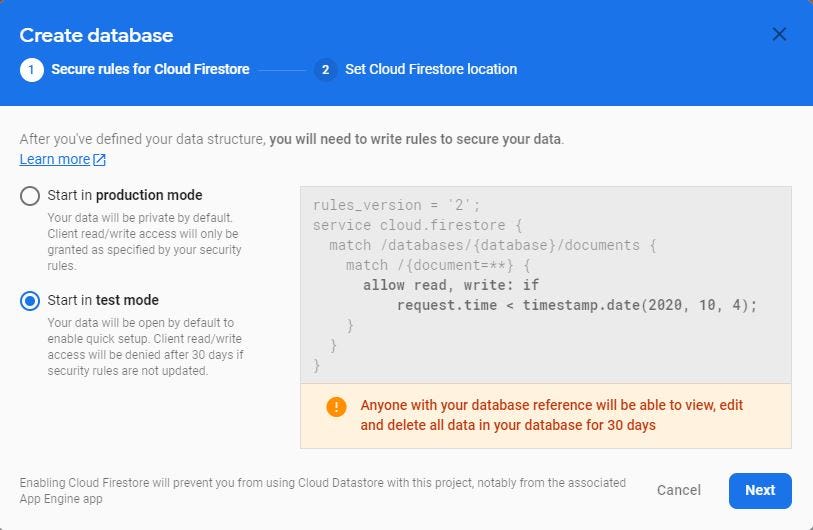
So for now as a beginner we have to select the Start in test mode, It removes all of the read-write restrictions from the database, Later on, we can change this script and allow only the users you want to modify the database.
Now you can click on NEXT → ENABLE and we are good to go, our database has been set up and the thing left is to integrate it with our application.
Now before starting we have to import AngularFirestoreModule in our projects app.module.ts, So that it can be used by other components in our project.
import { AngularFirestoreModule } from '@angular/fire/firestore';
And add to the imports section in app.module.ts file.
imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebase), IonicModule.forRoot(), AngularFirestoreModule, AppRoutingModule, IonicStorageModule.forRoot({ name: 'userdb' }), ]
Now we are ready to start CRUD(Create, Read, Update, Delete) operations with firebase and ionic. We are going to see all these operations one by one:
import { AngularFirestoreCollection, AngularFirestore } from ‘@angular/fire/firestore’; in your page.ts
and in the constructor inject:
constructor(private firestore
: AngularFirestore) { }
- Create
You will need to add the createDatabse()
method to persist an item collection in the Firestore database:
createDatabse(items: Item){
return this.firestore.collection('itemsList').add(items);
}
2. Read
You will need to add the readDatabse()
method to retrieve the available item from the Firestore collection:
readDatabse() {
return this.firestore.collection('policies').snapshotChanges();
}
3. Update
Next, you need to add the updateDatabse()
method to update an item data by its identifier:
updateDatabse(items: Item){
delete items.id;
this.firestore.doc('itemsList/' + items.id).update(items);
}
4. Delete
Finally, you can add the deleteItem()
method to delete an item by its identifier:
deleteItem(itemId: string){
this.firestore.doc('itemsList/' + itemId).delete();
}
You can find the fully-functioning ionic-firebase starter kit with lots of more features HERE.
Conclusion
In this tutorial, we learned how to deploy our ionic app on firebase using the firebase hosting method, then we got to know what are the various tasks in firebase authentications modules with the ionic app and how to achieve those?.
Last but not least we got to know how we can connect our firebase database with our ionic application very easily and we understood the methods and functions of firebase CRUD operation in the ionic application. Firebase provides us with these facilities for free up to a limit. Firebase is truly a great match for modern-day mobile apps and is really developer-friendly.
Next Steps
If you liked this blog, you will also find the following Ionic blogs interesting and helpful. Feel free to ask any questions in the comment section
- Ionic Payment Gateways — Stripe | PayPal | Apple Pay | RazorPay
- Ionic Charts with — Google Charts| HighCharts | d3.js | Chart.js
- Ionic Social Logins — Facebook | Google | Twitter
- Ionic Authentications — Via Email | Anonymous
- Ionic Features — Geolocation | QR Code reader| Pedometer
- Media in Ionic — Audio | Video | Image Picker | Image Cropper
- Ionic Essentials — Native Storage | Translations | RTL
- Ionic messaging — Firebase Push | Reading SMS
- Ionic with Firebase — Basics | Hosting and DB | Cloud functions
Ionic React Full App with Capacitor
If you need a base to start your next Ionic 5 React Capacitor app, you can make your next awesome app using Ionic 5 React Full App in Capacitor
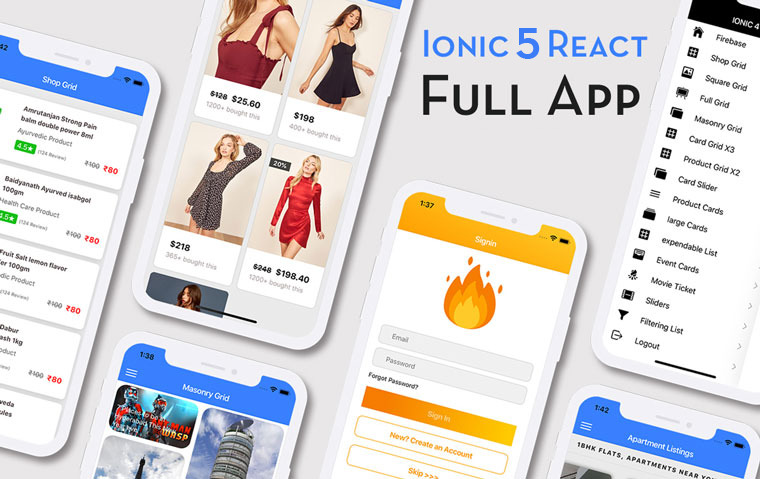
Ionic Capacitor Full App (Angular)
If you need a base to start your next Angular Capacitor app, you can make your next awesome app using Capacitor Full App
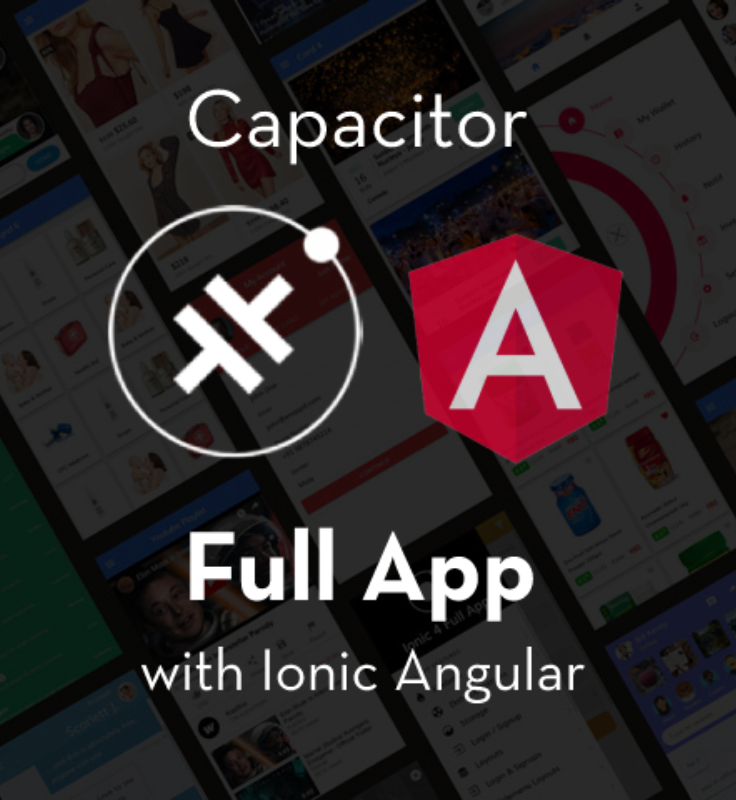
Ionic Full App (Angular and Cordova)
If you need a base to start your next Ionic 5 app, you can make your next awesome app using Ionic 5 Full App
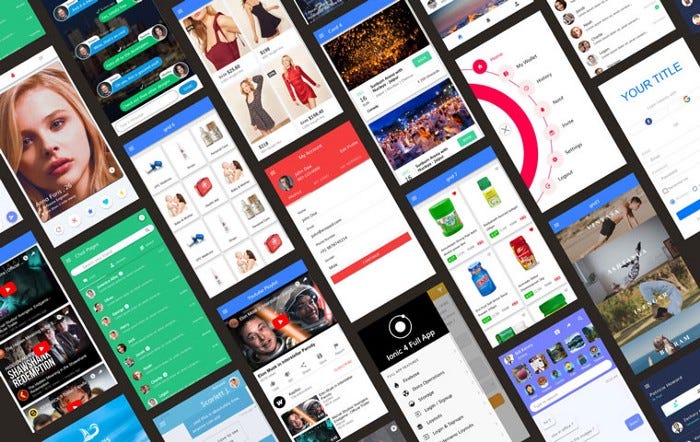