How to add Video Player in Ionic 4 App
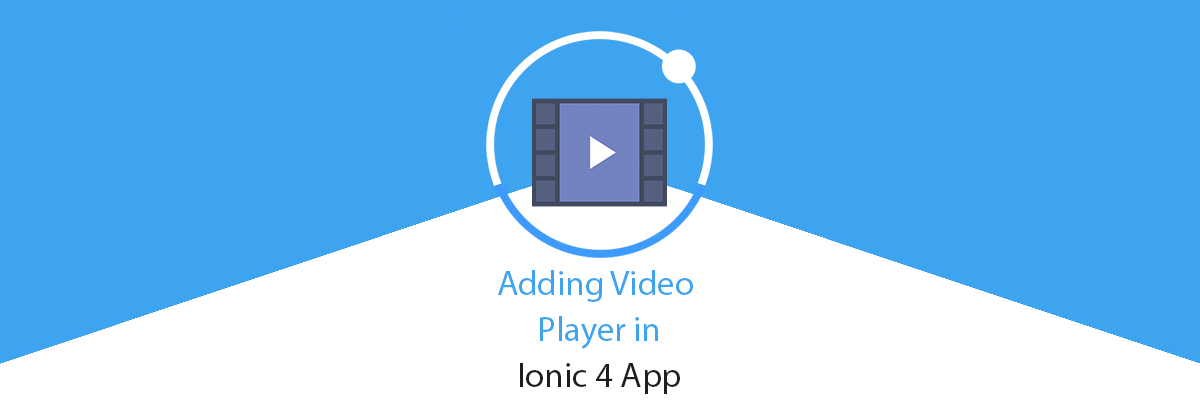
In this post, you will learn how to Add video players in Ionic 4 apps using Ionic Native Plugins. We will also learn how to add Youtube hosted video in a simple Ionic 4 app and test.
Complete source code of this tutorial is available in the Video Player In IONIC 4
What is Ionic 4?
You probably already know about Ionic, but I’m putting it here just for the sake of beginners. Ionic is a complete open-source SDK for hybrid mobile app development. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices.
In other words — If you create native apps in Android, you code in Java. If you create native apps in iOS, you code in Obj-C or Swift. Both of these are powerful, but complex languages. With Cordova (and Ionic) you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS.
Post structure
We will go in a step-by-step manner to explore how to add video player and Youtube video in IONIC 4 app
STEPS
- Create a simple IONIC app
- Install Plugin for Video Player in IONIC 4 app
- Play Video in IONIC 4 app with local URL and Server URL
- Play Youtube uploaded Video in ionic 4 app
We have three major objectives
- Play the locally stored video in IONIC 4 app
- Play video which is hosted on a server in IONIC 4 app
- Play Youtube video in IONIC 4 app
Let’s dive right in!
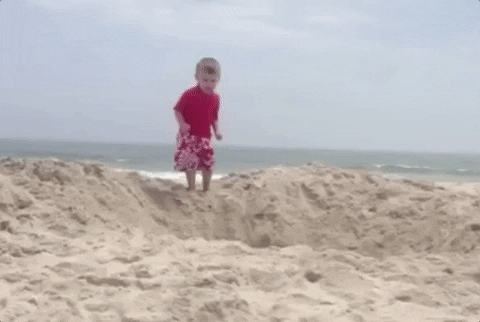
Step1 — Create a simple Ionic 4 app
I have covered this topic in detail in this blog.
In short, the steps you need to take here are
- Make sure you have node installed in the system (V10.0.0 at the time of this blog post)
- Install ionic cli using npm
- Create an Ionic app using
ionic start
You can create a blank
starter for the sake of this tutorial. On running ionic start blank
, node modules will be installed. Once the installation is done, run your app on browser using
$ ionic serve
Step: 2 — Install Plugin for Video Player in IONIC 4 app
moust cordova videoplayer plugin
A Cordova plugin that simply allows you to immediately play a video in fullscreen mode.
Requires Cordova plugin: com.moust.cordova.videoplayer
. For more info, please see the VideoPlayer plugin docs.
Installation
For that, open your terminal and type
ionic cordova plugin add https://github.com/moust/cordova-plugin-videoplayer.git
It’s a bit clumsy to work with Cordova plugin so the ionic team created Ionic Native, which is a wrapper for the Cordova plugins so we can use them in a more “Angular/Ionic” way.
So now we will open our terminal and try this command to install Facebook package from Ionic Native
npm install @ionic-native/video-player
Step:3 — Play Video in IONIC 4 app with local URL and Server URL
Using this plugin The first step you will need to do is Add this plugin to your app’s module
Import this plugin like this
import { VideoPlayer } from '@ionic-native/video-player/ngx';
and add this to Providers of your app Like this
providers: [
StatusBar,
SplashScreen,
VideoPlayer,
{ provide: RouteReuseStrategy, useClass: IonicRouteStrategy }
],
So after Adding Your app.module.ts look like this
Now time to import this plugin in your home.ts in which we are integrating our Video Player
So for using this plugin in our home.ts first, we will import the plugin like this
import { VideoPlayer } from '@ionic-native/video-player/ngx';
and inject it in your Constructor (Dependency injection) like this
constructor(private videoPlayer: VideoPlayer, public modalCtrl: ModalController) {
}
And use this code for Adding Video Player in IONIC App
this.videoPlayer.play('file:///android_asset/www/assets/SampleVideo.mp4').then(() => {
console.log('video completed');
}).catch(err => {
console.log(err);
});
If you want to play hosted video on server. you can change this local URL into your video hosted URL like this.
this.videoPlayer.play('https://sample-videos.com/video123/mp4/720/big_buck_bunny_720p_1mb.mp4').then(() => {
console.log('video completed');
}).catch(err => {
console.log(err);
});
so this code simply allows you to immediately play a video in fullscreen mode.
After adding this code your home.ts will something look like this
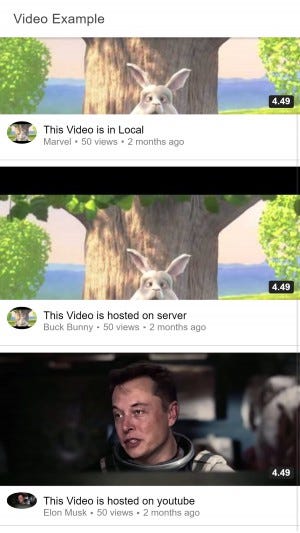
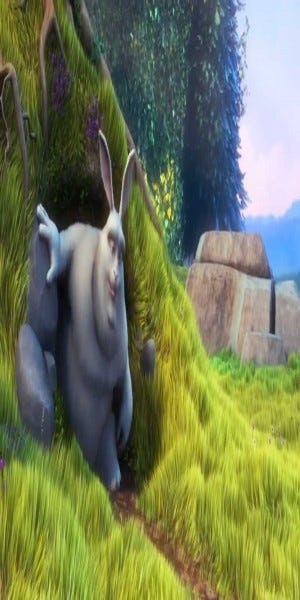
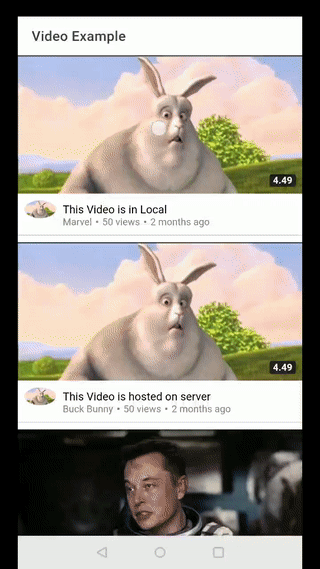
Step:4 — Play Youtube uploaded Video in ionic 4 app
In this step we will use iframe for playing Youtube video in IONIC 4 app
For this you have to use DomSanitizer
API from @angular/platform-browser
package
Import this API like this
import {DomSanitizer} from '@angular/platform-browser';
and eject it in our Constructor (Dependency injection) like this
constructor(public sanitizer:DomSanitizer) {
}
And we will use this API like this in our HTML
<iframe [src]="sanitizer.bypassSecurityTrustResourceUrl(videourl)" allow="autoplay;" frameborder="0"
allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
where video Url is basically what we want to play
DomSanitizer helps preventing Cross Site Scripting Security bugs (XSS) by sanitizing values to be safe to use in the different DOM contexts.
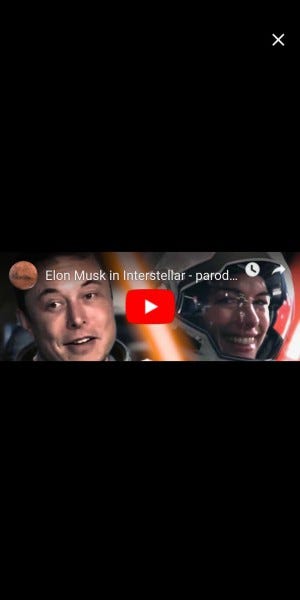
So After Adding This code, our html is something look like this.
Conclusion
In this blog, we learned how to implement Video Player In IONIC 4 app and also we have learned how to add Youtube video in IONIC 4 app
Complete source code of this tutorial is available in the Video Player in IONIC 4 app