Custom icon and splash in Ionic React Capacitor apps
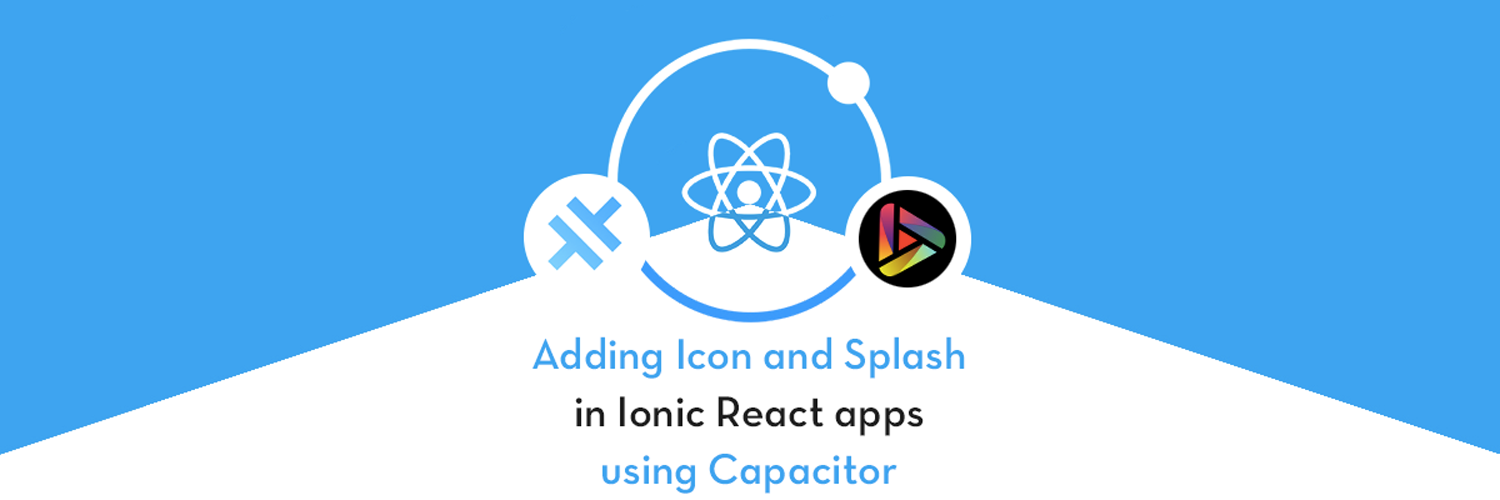
In this post, you will learn how to make custom Icon and Splash in your Capacitor Ionic React app.
Capacitor is the latest buzz in hybrid app world. It is created to be a replacement and an improvement over Cordova. Creating icon and splash is pretty straight-forward in Cordova apps. You can need to create two .png
files and run Cordova commands. But Capacitor gives user total command over native project code. So you’ll have to use native IDEs to create icons and splash for Ionic-React Capacitor apps. Also, Capacitor has its own Splash API for controlling Splash implementation.
In this post, you will learn
- How to create custom icon and splash for Capacitor Android apps using Android Studio
- How to create custom icon and splash for Capacitor iOS apps using Xcode
We will be doing this for Ionic-React apps in Capacitor, which doesn’t make much difference from Angular, as Icon and Splash are front-end independent.
Let’s see a brief intro to each of the included frameworks (Skip this if you already know about these) :
- Capacitor
- Ionic-React
What is Capacitor ?
Cordova helps build Ionic web app into a device installable app. But there are some limitations of Cordova, which Capacitor tries to overcome with a new App workflow.
Capacitor is a cross-platform app runtime that makes it easy to build web apps that run natively on iOS, Android, Electron, and the web. Ionic people call these apps “Native Progressive Web Apps” and they represent the next evolution beyond Hybrid apps.
Capacitor is very similar to Cordova, but with some key differences in the app workflow
Here are the differences between Cordova and Capacitor
- Capacitor considers each platform project a source asset instead of a build time asset. That means, Capacitor wants you to keep the platform source code in the repository, unlike Cordova which always assumes that you will generate the platform code on build time
- Because of the above, Capacitor does not use
config.xml
or a similar custom configuration for platform settings. Instead, configuration changes are made by editingAndroidManifest.xml
for Android andInfo.plist
for Xcode - Capacitor does not “run on device” or emulate through the command line. Instead, such operations occur through the platform-specific IDE. So you cannot run an Ionic-capacitor app using a command like
ionic run ios
. You will have to run iOS apps using Xcode, and Android apps using Android studio - Since platform code is not a source asset, you can directly change the native code using Xcode or Android Studio. This give more flexibility to developers
Due to these reasons, we’ll have to add custom Icon and Splash manually in each platform, using the platform’s IDE — Android Studio and Xcode
Plugins in Capacitor
Cordova and Ionic Native plugins can be used in Capacitor environment. However, there are certain Cordova plugins which are known to be incompatible with Capacitor. To build Splash functionality, we’ll use Capacitor’s Splash API. Icon doesn’t require any API from the framework.
Other than that, Capacitor also doesn’t support plugin installation with variables. Those changes have to be done manually in the native code.
Why Ionic React ?
(Read carefully)
Since Ionic 4, Ionic has become framework agnostic. Now you can create Ionic apps in Angular, React, Vue or even in plain JS. This gives Ionic great flexibility to be used by all kinds of developers.
It is important to note that Ionic React apps are only supported by Capacitor build environment.
Same is not true for Ionic Angular apps — Ionic Angular apps are supported by both Cordova and Capacitor build environments.
Hence, if you want to build apps in Ionic React, you need to use Capacitor to build the app on device.
I know if can get confusing as three frameworks are crossing their paths here. Bottom line for this post — Ionic + React + Capacitor with Splash API
Structure of Post
We’ll follow a stepped approach to create Icons and Splash in this Ionic React Capacitor App. Following are the steps
- Step 1 — Create a basic Ionic 4 React app
- Step 2 — Integrate Capacitor in the app
- Step 3 —Create Icon and Splash for Android
- Step 4 — Create Icon and Splash for iOS
- Step 5 —Conclusion
So let’s dive right in !
Step 1 — Create a basic Ionic-React app
First you need to make sure you have the latest Ionic CLI. This will ensure you are using everything latest (Duh ! ) . Ensure latest Ionic CLI installation using
$ npm install -g ionic@latest
Creating a basic Ionic-React app is not much different or difficult from creating a basic Ionic-Angular app. Start a basic blank
starter using
$ ionic start IRIconSplash blank --type=react
The --type=react
told the CLI to create a React app, not an Angular app !!
Run the app in browser using (yes you guessed it right)
$ ionic serve
You won’t see much in the homepage created in the starter. Just a blank page saying the “world is your oyster”. Yeah, like we didn’t know that 😄
Step 2— Integrate Capacitor in the app
Capacitor can be attached to an existing Ionic app as well. To attach Capacitor to your existing Ionic app, run
$ ionic integrations enable capacitor
This will attach Capacitor to your Ionic app. After this, you have to init
the Capacitor app with
$ npx cap init YOUR_APP_NAME YOUR_APP_ID
You should already be having YOUR_APP_NAME
and YOUR_APP_ID
in config.xml
. Use the same here, so that there is no mismatch or confusion.
Build the app in Device
Build the app in either an Android or iOS device to see default icon and splash. First build the web assets using ionic build
, then add platform using
$ npx cap add android $ npx cap add ios
Here’s how the default icon and splash looks in an Android device
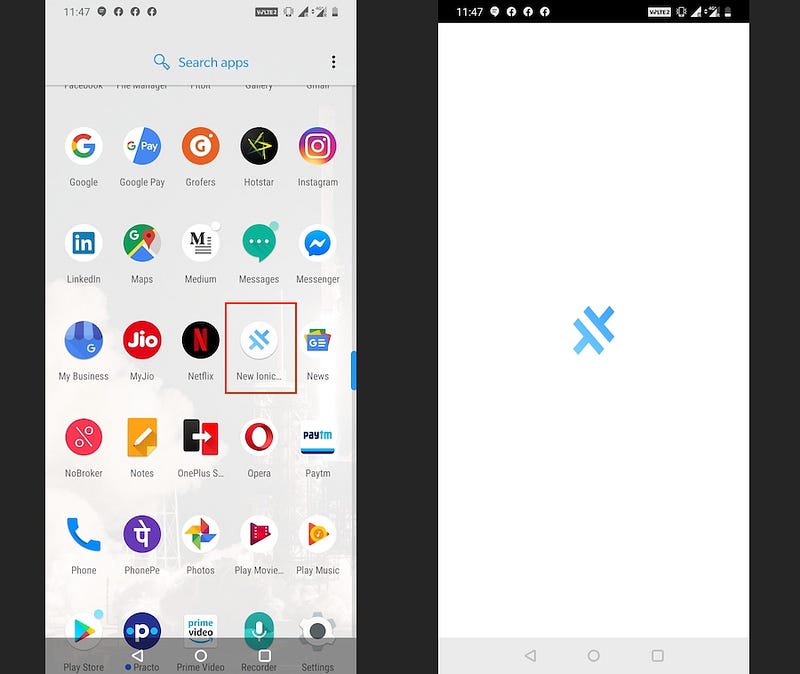
Step 3 — Create Icon and Splash for Android
Icons and Splash are made for all varieties of devices, so we’ll prepare the basic asset first.
Prepare base asset
Icon — requires a 1024x1024 png file (transparency allowed)
Splash — requires a 4096x4096 png file (no transparency) with the important stuff centered
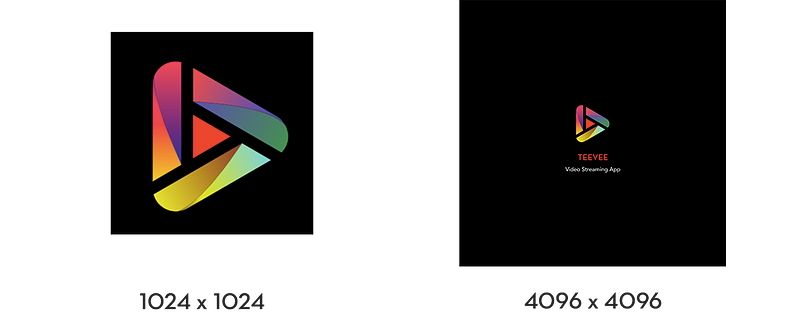
Generate all Sizes
It is cumbersome to create all sizes of Icon and Splash manually. For this, you can use online generators. Couple of them are
- ApeTools (recommended) — creates both icon and splash for iOS and Android
- MakeAppIcon — creates only icons for iOS and Android
Using Ape Tools, you can just select Android and iOS bundles, and generate all assets
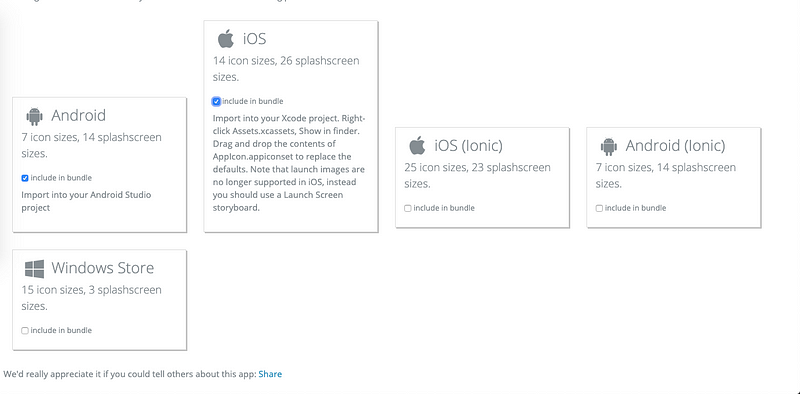
The generates assets will look like this, all sizes for iOS and Android
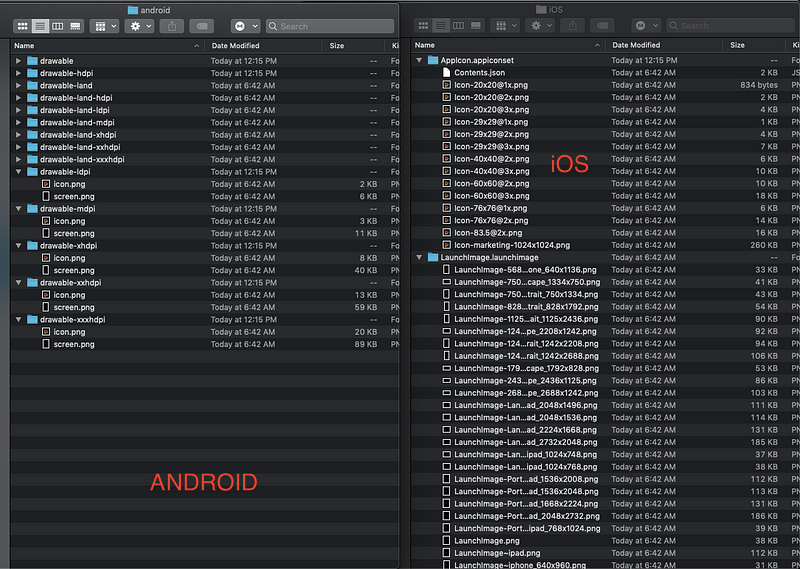
Add Icon assets using Android Studio
Once you have Android platform added in your project, open the project in Android studio using
$ npx cap open android
Icons in Android Platform reside in mipmap folder in app → res → mipmap
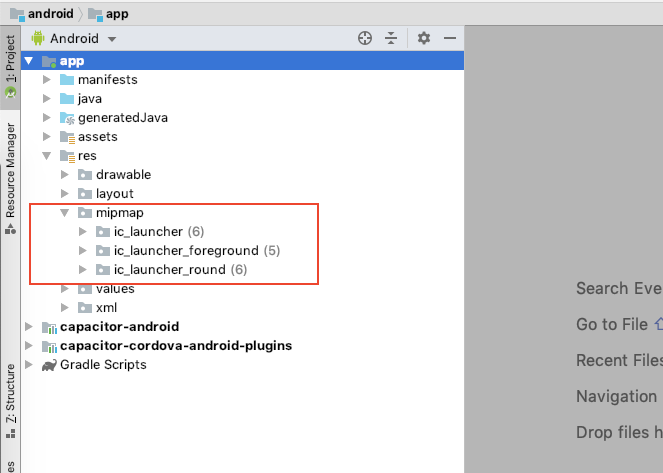
There are two ways of creating custom icon for the project
- Replace each individual icon with your custom assets, taking care of the file names
- Create new icon asset in Android Studio itself
Let’s take method 2, as it is faster. Right-click on res
folder, New → Image asset
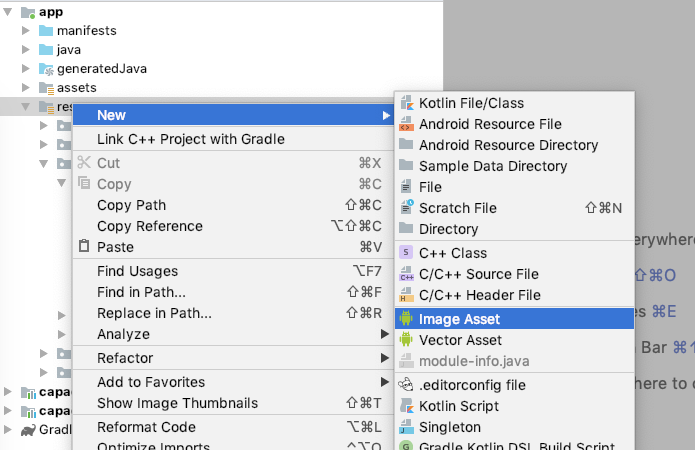
It will open another window, where you can select your icon image, and check previews of the icon
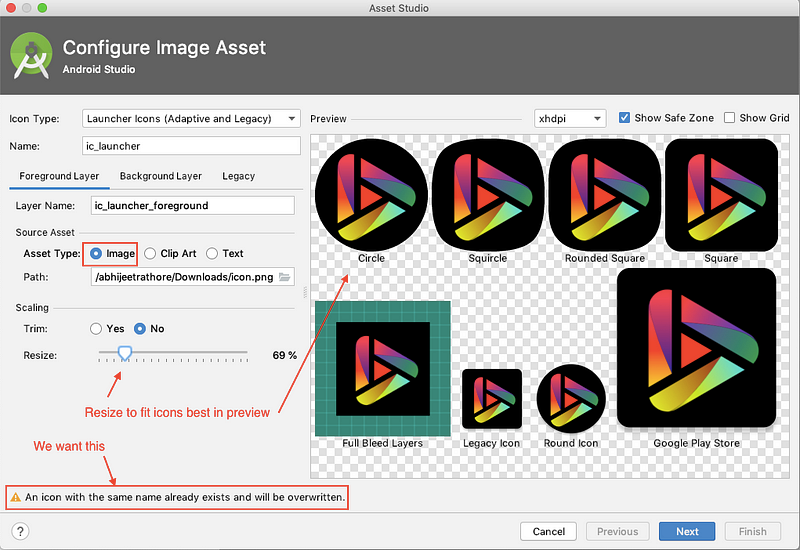
Finish the operation, and it will overwrite the existing icon files.
Add Splash assets using Android Studio
Adding splash is not that straight-forward. You’ll have to replace the splash files manually in each folder. Splash files can be found in
Android → res → drawable → splash
A better way to see these files is to change the top menu option to Project Files. You’ll now see the splash folders in
app → src → main → res
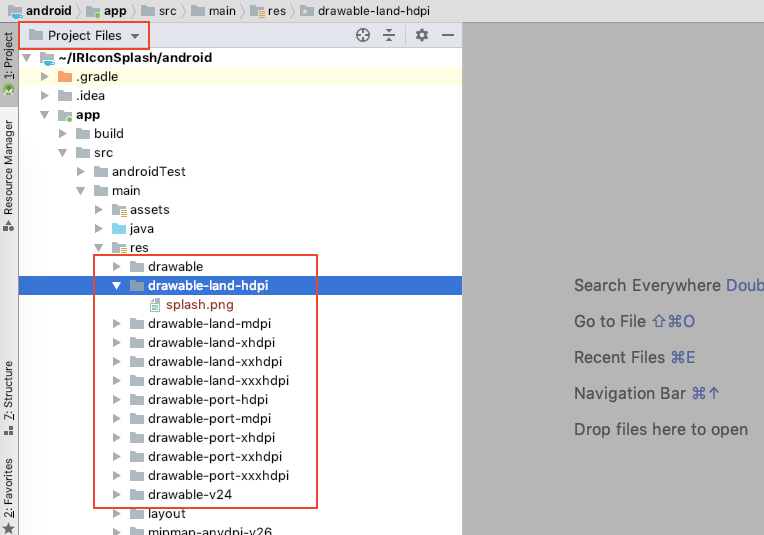
You can replace these splash.png
with the downloaded assets, keeping in mind the mdpi
, hdpi
etc. resolutions.
Note: When replacing, please take care that the folder and file names remain the same.
Here’s how my new icon and splash look like
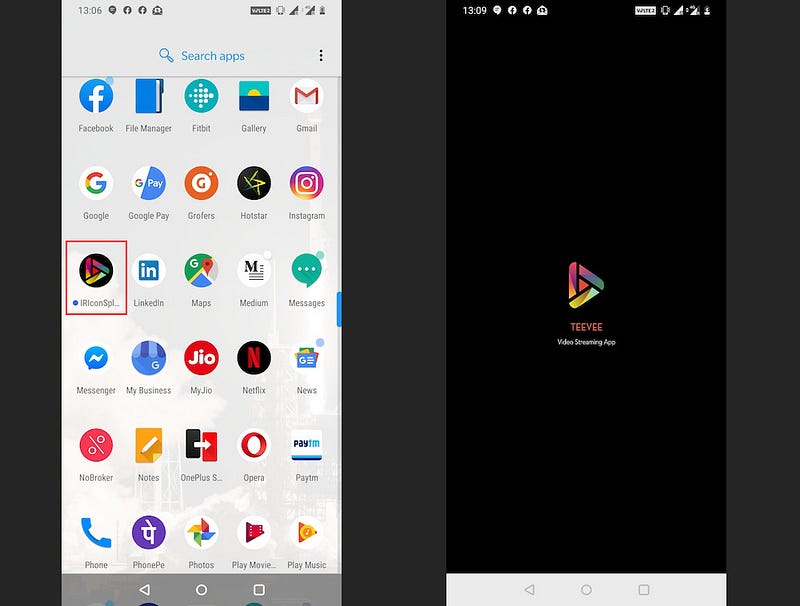
Step 4— Create Icon and Splash for iOS
For iOS also, the process is pretty similar. You add custom Icon and Splash assets in Xcode, using the assets you created from the generator
Prepare base asset
Icon — requires a 1024x1024 png file (any transparency will be converted to black by iOS)
Splash — requires a 4096x4096 png file (no transparency) with the important stuff centered
You already have the generated resources from ApeTools.
Add Icon assets using Xcode
Go to left sidebar, App → Assets.xcassets → AppIcon, and here you can replace the default icon set with your downloaded assets. Just drag and drop correct files on the correct placeholder, as shown below. For iPhone purpose, you only need to replace the top 8 icons + iTunes store icon at the very bottom. If you are building the app for iPad as well, then replace all the icons.
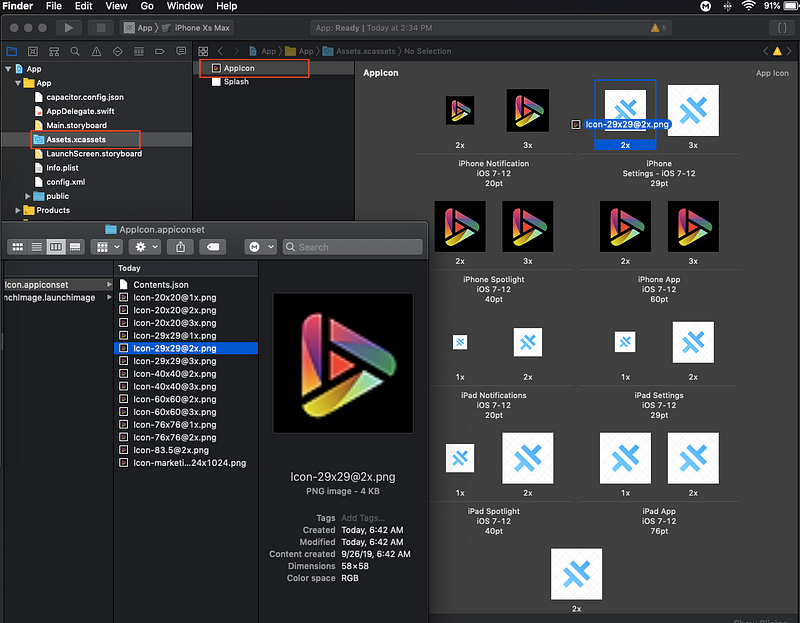
Add Splash assets using Xcode
Similar to replacing icons, go to left sidebar, App → Assets.xcassets → Splash, and replace the default splash image set with your downloaded assets.
Here you will see just three images under “universal” title. You can simply replace these with your 4096x4096 png, and Xcode will automatically crop it as per device. Make sure your useful content is in the center region, which doesn’t get cropped even for the smallest iPhone.
Just drag and drop correct files on the correct placeholder, as shown below.
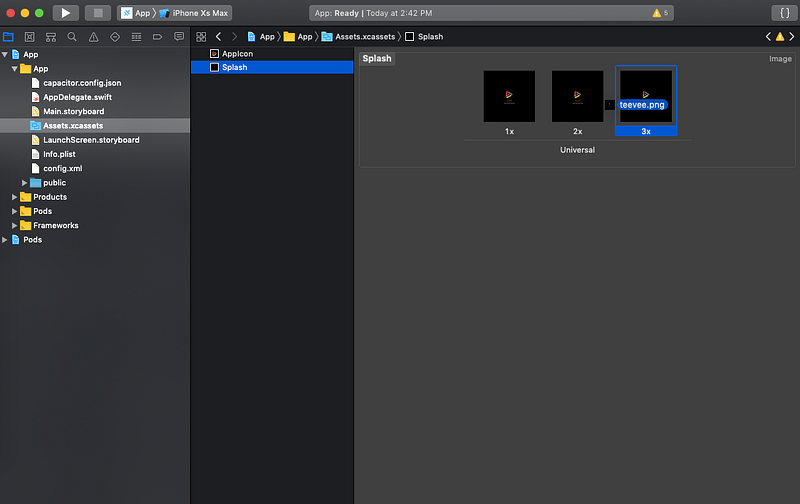
Now build the app on device or Simulator. The custom icon and Splash will look like this
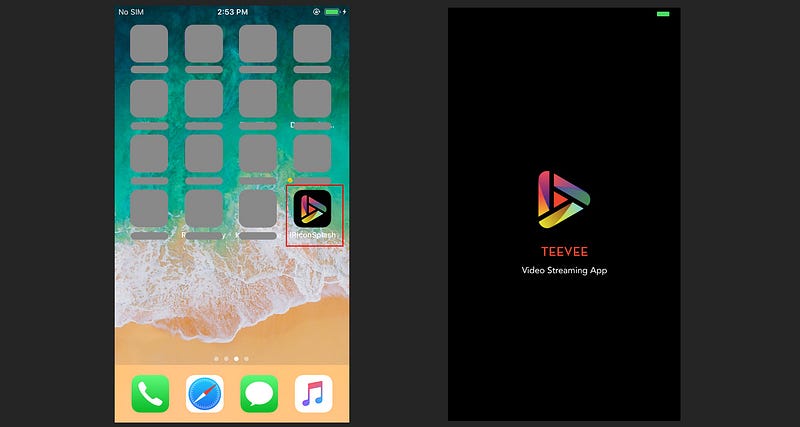
Congratulations, you have added custom icon and splash to your Capacitor apps. Next section we’ll see how to control Splash using Capacitor Splash API
Conclusion
In this post, we learnt how to add custom icon and splash in any Capacitor based app. While Cordova makes it very easy to add icons and splash, Capacitor allows user to access native project directly, and add icon/splash in Android Studio and Xcode.
Be careful when adding your icons and splash, as this will be the face of your app when it is published. Take your time to process and test each new icon/splash in different devices to make sure it appears properly, not pixelated or cropped somewhere.
Happy Coding !