Social sharing component in Ionic 5 - Mobile & Web apps
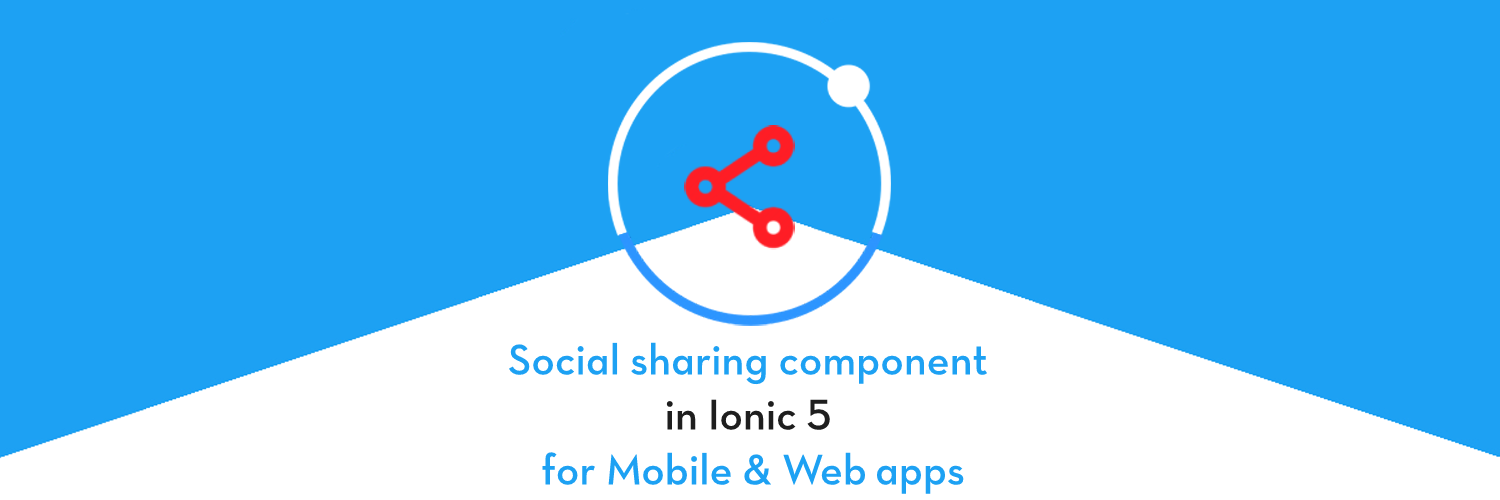
Social sharing is one of the most parts in a mobile app today. We all want to share the news, the new video, that one awesome meme or the photo we took when traveling, to all our friends. There can be various social sites or apps through which you can share the data like Twitter, Facebook, Google (i.e. through drive or email), Pinterest and more.
In this tutorial, we will cover some of the social sharing features in Ionic apps and will create separate component for it. You can use that component in any of your Ionic web or mobile apps.
Types of Social Sharing
As you might be aware, Ionic apps are almost as powerful as native apps. You can share a lot of types of data with your Ionic apps as far as the capability goes, but the Cordova / Phonegap / Capacitor plugins available for Social sharing in Ionic apps like to share things is specific ways. Following are the default types of data you can share across different apps using Ionic social share
- Text
- URLs — Which can be auto-read by apps to display a preview
- Image
- Files
- Emails — With subject, body text and attachments
Depending on the type of device (Android, iOS) and types of apps you have installed on your device, the sharing experience might differ slightly, but overall the sharing mechanism used is the same as native apps. In other words, whatever options you get while sharing data in a native app in your device, you can get the same sharing options in Ionic apps as well.
Forms of Ionic
Ionic has made itself language agnostic to some extent, integrating some of the most popular JS frameworks. At present, Ionic apps can be written with
- AngularJS as front-end
- ReactJS as front-end
- VanillaJS as front-end
- VueJS as front-end
- Cordova as build environment
- Capacitor as build environment
As you can see, Ionic can be adopted by a large community of developers, and hence there are always confusion about which plugin to use in which framework. This post is targeted towards Social Sharing in Ionic Apps with Angular Cordova framework
Structure of the post
As always, we’ll go through the post step-by-step
- Set up an Ionic Application
- Install social sharing plugin
- Create Social share component and add logic
- Implement Social share in Ionic Web View
- Test sharing in mobile device
- Test sharing in web app
Step 1: Set up an Ionic Application
First you need to make sure you have the latest Ionic CLI. This will ensure you are using everything latest. Ensure latest Ionic CLI installation using
$ npm install -g ionic@latest
At the time of writing this blog, this is my working environment
- Node V 14.x / NPM V 7.x / Angular 11.x / Ionic 5.5 / Cordova 10.0
You can create a new Ionic 5 Angular project using the below command OR use any existing application. If you want to learn more about how to create Ionic Apps — you can go through our Beginners blog.
$ ionic start socialShare blank --type=angular --cordova
--cordova
tells the CLI to create a Cordova integration- — type=angular creates an Angular app (there is a choice of React and Vue as well)
blank
template gives a blank home page
This will create a new blank application in your working directory with the name socialShare. More details on how to create an Ionic App is in this blog, Once the project is created, now we will generate the social-share component.
Step 2: Install Social Sharing Plugin
To implement social share, first we will install the required packages that will help us to share the content through the Ionic 5 application. To install package run the below command :-
$ ionic cordova plugin add cordova-plugin-x-socialsharing $ npm install @ionic-native/social-sharing
Once the Social share Plugin is installed, we can now create the modular Social Sharing Component, which you can use in multiple places in the same app, OR, just copy paste it and integrate easily with any new Ionic app. Writing the logic in a component keeps the whole logic as a package which can be used / moved anywhere in the app.
Step 3: Create social share component and add logic
To create the Ionic 5 component run the below command, this will create the component which will be passed in Modal Controller (when we click ‘Share’ button, this Modal will pop-up in the app)
$ ionic generate component components/social-share
Above command will generate the social share component in the project directory and project structure will look like below :-
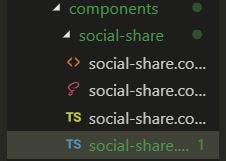
Now social share component can be used in either Ionic web app or in Mobile app. In mobile app, cordova is used to implement these features and to implement it in Web app, we will use a simple solution that will share the data using links.
Implement Social UI in component :-
Inject the social sharing package and component into app.module.ts
:-
Now we will look at the HTML of the social share component, you can modify the UI according to you. below is the HTML code for component :-
In the above code, we will render the list containing social sharing options. You can add more options to the list, currently we have WhatsApp, Facebook, Twitter, Instagram and Email as the sharing options. Add the below CSS to give the Styles to the HTML page.
Open the Sharing component from HomePage
To open the Sharing Modal from HomePage
, you just need to create a button, and open the Modal using a modal Controller. Following method is required in HomePage
, I believe you can figure out the HTML / CSS of the HomePage
on your own.
Now on clicking the ‘Share’ button, the pop-up will open and look like the following.
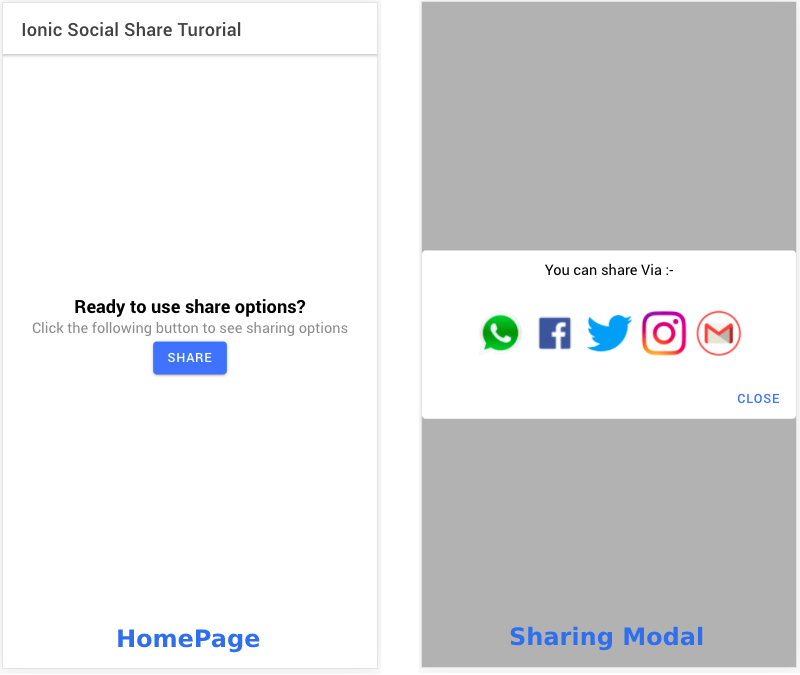
Sharing logic
Now we will look at the Logic related to the Social Sharing in Ionic 5 Mobile application using Cordova plugin :-
In the above code, To share the data, text, url or photos we use the sharing methods (i.e. shareViaWhatsApp, shareViaFacebook, shareViaTwitter and shareViaInstagram).
this.socialSharing[`${shareData.shareType}`](this.sharingText, this.sharingImage, this.sharingUrl) .then((res) => {})
Here in this.socialSharing[shareType]
, shareType
is the attribute defined in the socialShare list. Also, for the email sharing, first we check whether the device supports email sharing using canShareViaEmail
You can add the below array to the environment.ts
file :-
Make sure your tsconfig.json
has following options set inside compilerOptions
. And also make sure you have the relevant icons as mentioned in the environment.ts
, and in the same location.
"compilerOptions": { ..., "baseUrl": "src", "paths": { "@app/*": ["app/*"], "@env/*": ["environments/*"], "@assets/*": ["assets/*"], "@angular/*": ["../node_modules/@angular/*"] }
4. Implement Social share in Ionic Web App
To implement the Social share feature in Web app, we simply use the URL redirection with data encoded into it. We can send the data in the form of link or text only.
First we will look at the HTML for the web view :-
In the above HTML there is a small change from mobile view, we have simply added the <a> tag with dynamic href and inside that <a> tag we add the image.
And we have rendered the list of socialShare options with href (i.e. dynamic URL with encoded data and URL). Below is the SCSS file that can be used with the HTML file.
There is not much logic in sharing in web app, because everything is taken care of by the URLs itself. If you look carefully in the URL data in next gist, you can see the parameters like media
, subject
, recipient
etc are part of the URL itself. So no separate plugin or logic is required for sharing.
The list we have to render for sharing on web is defined in environment.prod.ts
file with title, logo and href.
Once again, make sure you have the relevant icons as mentioned in the environment.prod.ts
, and in the same location.
Href
attribute used in list is redirecting URL with data and link inside it, But this type of social sharing is limited as all the social sites do not have the redirecting URL. We have covered Facebook, Twitter, Pinterest and Email in this tutorial (In web View).
To open this social sharing component, again, we simply have to open the component from the HomePage
. This time we’ll add the transparent CSS styling to the component as well.
const modal = await this.modalController.create({ component: SocialShareComponent, cssClass: 'backTransparent' }); modal.present(); }
Below is the CSS code that make the background transparent, you have to define the CSS class in global.scss
.
.backTransparent { --background: transparent !important; }
The web-app looks pretty much the same as mobile app (in responsive mode). We’ll see how the sharing looks a little different in the testing section (Step5 and 6)
Step 5 — Testing Social sharing on mobile device
Let’s build the app for Android (easier) and test the social sharing. iOS app will behave pretty much the same, except for the different iOS native sharing UI.
To build the app, add android
platform to the project using
$ ionic cordova platform add android
Once the platform is added, build the APK to be installed using
$ ionic cordova build android
and run the app in device using (device should be attached via USB, in developer mode)
$ adb install <<APK address>>
Or you can directly run the APK using (device should be attached via USB, in developer mode)
$ ionic cordova run android
If you face AndroidX
related errors during build, try one or all of the following
- Make sure the following options are set in your
gradle.properties
in android platform
android.useAndroidX=true
android.enableJetifier=true
- Add the following inside the
<platform name=”android”>
tag in yourconfig.xml
<preference name="AndroidXEnabled" value="true" />
- Add following two plugins and re-build the android app
$ ionic cordova plugin add cordova-plugin-androidx $ ionic cordova plugin add cordova-plugin-androidx-adapter
Once the build is successful, run it on the device. Following clip shows sharing on an ionic mobile app via all 5 options we discussed above
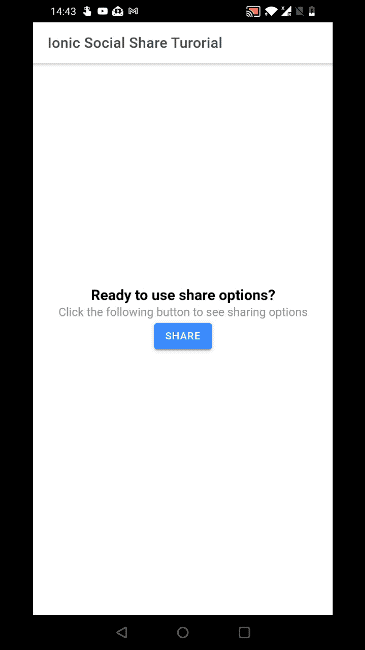
Step 6 — Testing Sharing on web app
You can directly test web app sharing capabilities in ionic serve
mode. The following clip shows how sharing works on web, using Ionic and the options we discussed in previous section.
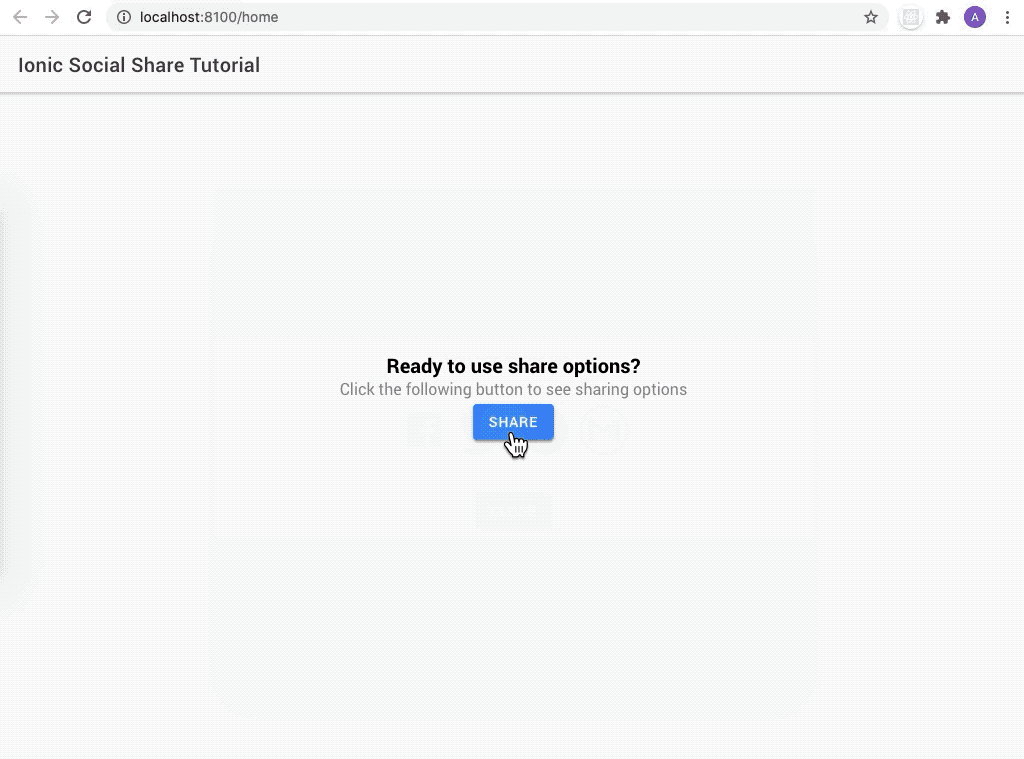
Congratulations, you have now learnt how to share data (text, image and url) in an Ionic web / mobile app. 🎉
Conclusion
You have the learnt how to make a Social Sharing Component in Ionic 5, which you can add to any of you apps and this will help grow your app with more and more sharing. You can use this social sharing component in both Web and Mobile Apps. You can also just pick this component folder, and put it in a new Ionic app, and will just start working out of the box.
Stay tuned for more Ionic blogs !