How to use Firebase Emulators with Ionic Angular Applications
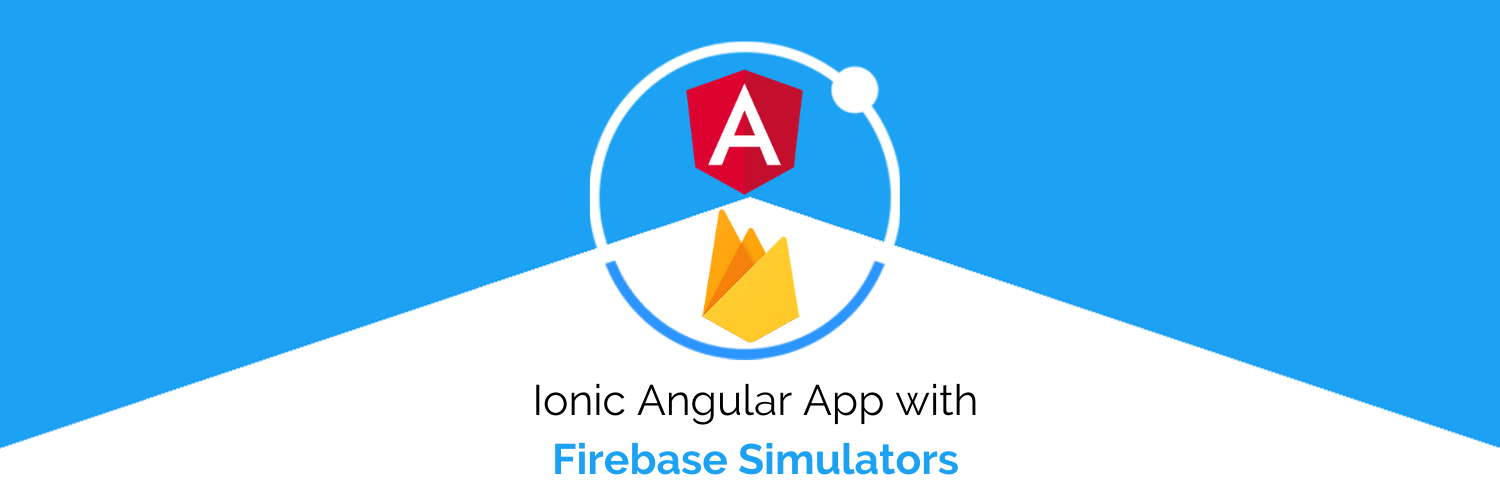
In this blog, we will go through a very important aspect of using Firebase backend Service — The use of Emulators.
First of all, we will need to understand — what is the need for these simulators?
As you know most Cloud Services charge you based on consumption and Firebase also acts similarly. Whenever you use any of their Services — your calls are charged. So it is not justified to use cloud services even in Debug mode or in a Scenario like Unit Testing (where you can make hundreds of calls in few seconds). To save such additional costs Simulators are the best way out. There can be other benefits also like total control over data, easy to clean database, etc.
Firebase 🔥
If you already know what is firebase — SKIP this section.
Firebase is a popular Backend-as-a-Service (BaaS) platform. It started as a YC11 startup and grew up into a next-generation app-development platform on Google Cloud Platform. It is getting popular by the day because of the ease of integration and the variety of functionalities available on it.
A lot of quick integrations are available with Firebase. Some of these are listed below:
- Real-time Database 🗄️
- FireStore Database 🗄️
- Email Authentication 📧
- Social logins📱
- In-app messages
- Push notifications
- Analytics 📊
- Crashlytics 💥
- Remote config
Firebase is quickly growing to become the most popular mobile app back-end platform. And now we got an easy way to handle the firebase project by using the emulator. You can be running a firebase project in the localhost rather than spending bucks on creating a cloud instance, It makes the work easy and clean.
Firebase Emulators 🤖
The Firebase Emulators make it easier to fully validate your app’s behavior and verify your Firebase Security Rules configurations. Use the Firebase Emulators to run and automate unit tests in a local environment, so that it doesn’t cost you for each firebase calls.
In Firebase Emulator, it provides various firebase services hosted on various local Server (Ports). The below list mentions some of the services which are available in the current Emulator and its default ports.
We can use almost all of the firebase features in its emulators like cloud firestore, Real-time database, Cloud Functions, and many more..!!
Setup Firebase Emulators in Ionic
Step 1 — Install Firebase CLI and other tools
Before installing Firebase Local Emulator Suite we need to install the Firebase-CLI, for more details you can go through these steps on their site.
To install the latest version, you can run the below command:-
$ npm i -g firebase-tools@latest
We have to check the firebase version installed on the local machine. To make the Emulator work we need the v8.4.* ( v8.4.1 OR greater) of firebase-tools.
$ firebase --version
Once Firebase CLI is installed, we have to configure the Emulator Suite that helps in test environments, anything from one-off prototyping sessions to production-scale continuous integration workflows.
Before installing the Emulator Suite you will need, so just keep them ready:
Step 2 — Initialize Ionic App
Before setting up the emulators, setup, a new Project (OR you can use the old ones if you have already working on Ionic Angular App).
We have to initialize the firebase project in an Ionic project. To do that run the below command to create a new Ionic project. For more details check out our blog here.
$ ionic start firebaseProject blank
This will create the new ionic project in the working directory.
Step 3— Initialize Firebase Project
Now initialize the firebase project inside the Ionic app root directory:-
$ firebase init
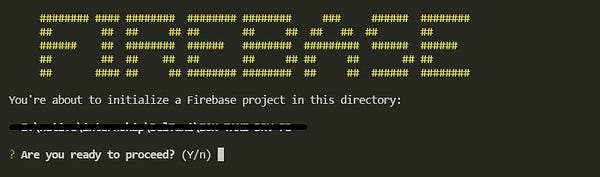
To initialize the project select ‘y’ in console, then select the firebase project OR create a new firebase project. You will be asked for some options further like below
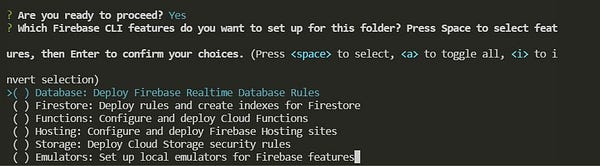
You can navigate the Emulators option by using the up 🔼 and down 🔽 arrow keys and selection is done using the spacebar. After that, you will be asked to select an existing project or to create a new firebase project.
Now you can select the features that you want to add to your firebase emulator:-

You can select only a few options you want OR you can select all the options — to see all the functionalities of the emulator in our local machine. After this firebase-cli will ask you for the port number where the Cloud Firestore, Cloud function, hosting emulators will run. We will select all default ports here unless you want to change for some reason
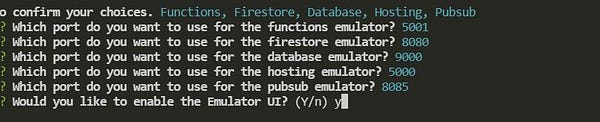
Finally, we have to enable the Emulator UI to get an interface using which we can interact with firestore, cloud function, and many more. Then firebase-cli will download the required emulator file to your local machine.

Now we are good to go..!!
Step 4— Run the Firebase Simulator
Let's start the firebase emulator and start interacting with the firestore and other features. To start the Firebase emulator we need to run the below command
$ firebase emulators:start
That will run an Emulator UI at the localhost:4000, And you will see an interface like this
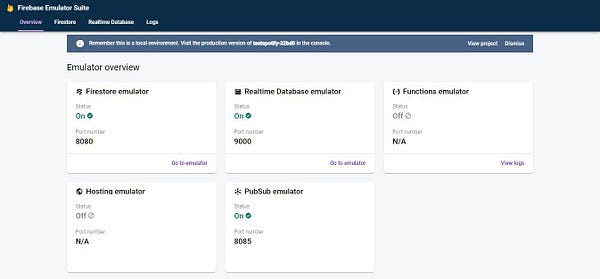
Here we can see all emulators are up and running, You can click on any of them and they will serve their purpose.
Connecting Firebase Project with Ionic Application
Now in this section, we will connect the firebase simulator with our Ionic Angular App. For a normal firebase cloud instance (not a simulator )we do the following :
You have to go on Firebase Console and then select the project that you have added in the Ionic project.
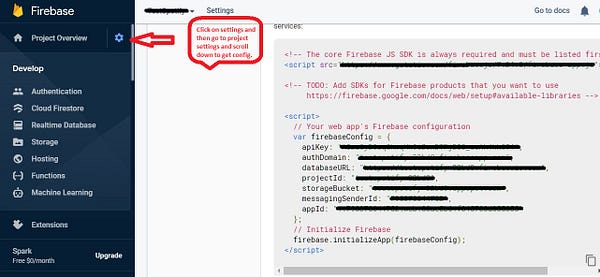
You can copy the above config and paste it in the Ionic App’s “src/environments/environments.ts” and later on initialize the firebase in “app.module.ts”. And if you want to know more(All) about firebase features you can visit and read our Blogs.
Now let's see what is different in the case of a simulator, we will actually go through the sample app code here. Don't worry too much about the code and logic of the app — just focus on firebase calls. You will find a small change later which makes it possible to use an emulator instead of a cloud project instance.
Example App (Grocery App Dashboard from Enappd)
To test the functionality, we have used a signup page with Email and password as the input and will enter in the Users collection.
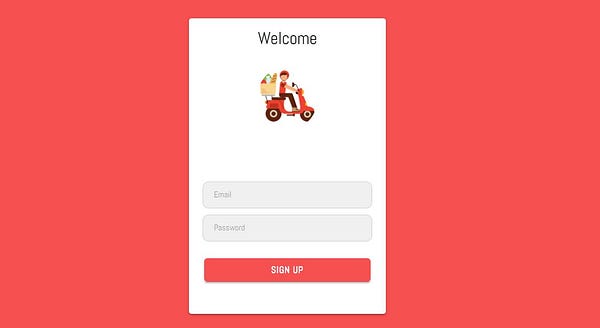
We have the code for making an entry in users' collection for this signup page.
Here we simply take some input from input fields and pass them in the function declared in API Service (this.api) — that you can create using ionic generate commands
The above code is from API Service, we have just created a user in firebase auth using this.auth.createAccount(user.email, user.password) and it returns the created user in firebase with some unique ID of the user (unique Id created by the Firebase). But till now we haven't saved our user details in the cloud firestore.
We will do that by sending the user details into the function created this.fs.createWithId(‘users’, userInfo) in firestore.service file (created using ionic generate command)
All of the above code was our app logic, now the important part — we have initialized the Firebase Emulator in this using few lines of code:-
intializeEmulator() { if (location.hostname === "localhost") { this.db.settings({ host: "localhost:8080", // port number for the firestore ssl: false }); console.log('Firestore Emulator connected') } }
In this we have just checked that if this project instance is running in the localhost or not ? If yes, connect to firestore local db instance running on port 8080.. !
Once the Emulator is initialized successfully then the “db” variable will contain the FireStore local instance using which we can add /update /delete documents from the various collections. And now it's time to make first entry in the collection.
public createWithId(collection: string, data: any): Promise<void> {
return this.db.doc(`${collection}/${data.id}`) .set(this.addCreatedAt(data));
}
The above code will add the document in the collection named user — this we have seen in the API Service file earlier.
Here how it will look on the Simulator. Great Success !! 🙌
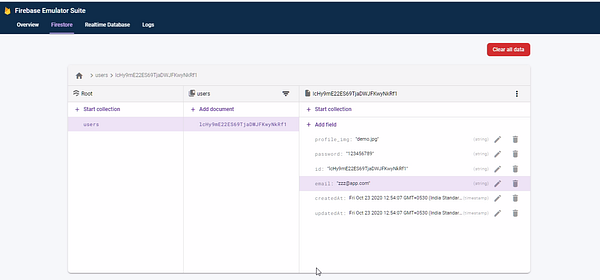
Conclusion :
In this tutorial, we have seen
- Why we need a Simulator and how it saves some cost?
- How to install and setup the Firebase Emulator?
- How to connect the Emulator with the Ionic Angular app?
Now it's your time, you can experiment with other features also and explore new things.