Ionic 5 Stripe Payment Integration - Firebase Cloud Functions v/s Node Express based Server
Stripe is one of the most widely used and fastest-growing payment gateway you can integrate into your website or app. It supports a wide variety of payment options and is quickly spreading across the globe. Stripe can take care of almost all your payment requirements in apps and websites. Stripe’s ease of integration has made it a popular developer choice over PayPal and other payment gateways. A good comparison between Stripe and PayPal can be studied here (spoiler — Stripe wins)
What is Ionic 5?
You probably already know about Ionic, so feel free to skip this section. I’m putting it here just for the sake of beginners.
Ionic is a complete open-source SDK for hybrid mobile app development created by Max Lynch, Ben Sperry, and Adam Bradley of Drifty Co. in 2013. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices by leveraging Cordova.
So, in other words — If you create Native apps in Android, you code in Java. If you create Native apps in iOS, you code in Obj-C or Swift. Both of these are powerful but complex languages. With Cordova (and Ionic) you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS.
Ionic 5 and Payment Gateways
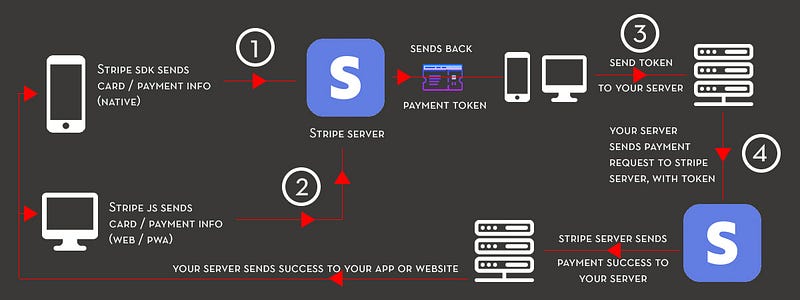
Ionic 5 can create a wide variety of apps, and hence a wide variety of payment gateways can be implemented in Ionic 5 apps. The popular ones are PayPal, Stripe, Braintree, in-app purchases, etc.
Stripe can be integrated into websites as well as mobile apps. There are different ways of integration of Stripe SDK. In this blog, we’ll learn how to integrate Stripe payment gateway in Ionic 5 apps
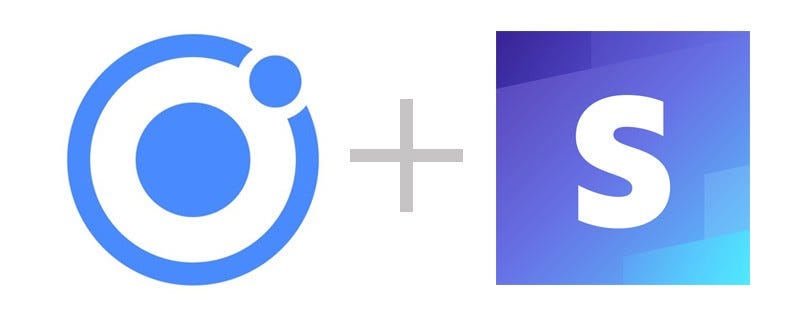
In this blog, we will use the cordova-plugin-stripe for the integration of stripe with our Ionic Application. And we will be covering the following steps
- Creating a new customer on Stripe server
- Adding payment cards and listing them in our Application
- Charging from the saved cards
For the integration of Ionic and Stripe, we have to build our own server-side script. In this blog, we will do it in 2 different ways
- Firebase Cloud functions
- NodeJS Express based API implementation.
Preparation 🧑🍳
Install Cordova Stripe Plugin
Before proceeding we will install the necessary plugin and add it to necessary files, Run the below command for installing stripe plugin —
$ ionic cordova plugin add cordova-plugin-stripe $ npm install @ionic-native/stripe
After installation completes, import the Stripe module in your app.module.ts
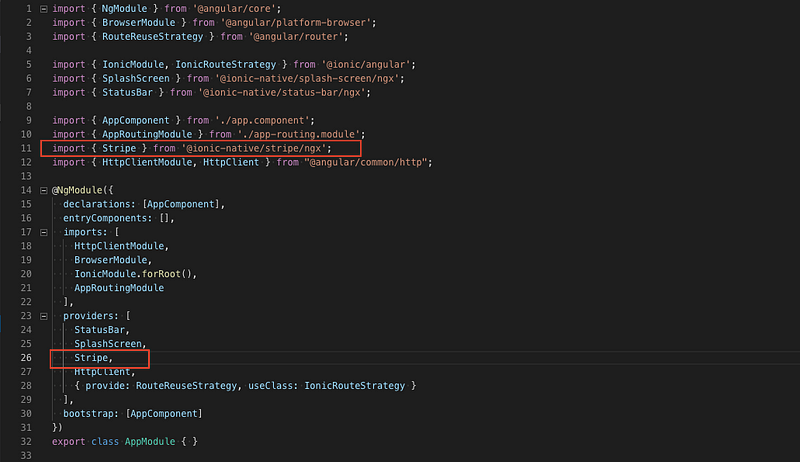
and also import Stripe in your stripe.page.ts
page file, like so
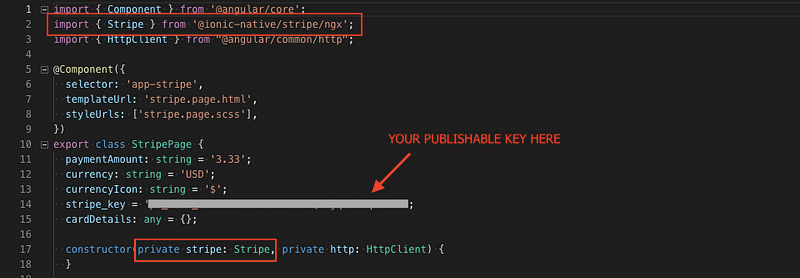
Before proceeding we want a pair of keys (public key and private key) to implement the stripe APIs in client and server. So to get it to follow the below procedure.
Setup Stripe Developer Account
Visit Stripe.com and create an account. Stripe payment services are currently available in limited countries as shown on this page.
Once you are inside the Stripe Dashboard, look for the Developer Tab -> API keys.
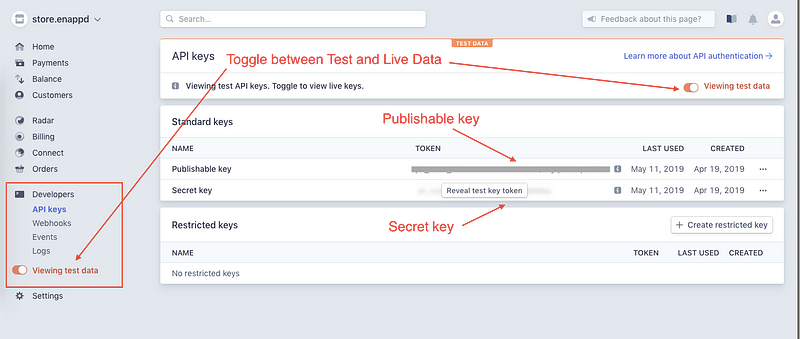
Publishable key is what you use for connecting the Strive Native SDK or Stripe.js in front-end. Secret key is used in the back-end, where your server connects with Stripe’s server for actual payment.
That’s all you need from the Stripe account, for now. This is your Test Mode/ Sandbox mode/ Developer mode. You can play with it and make any kind of API calls, dummy payments, etc without any cost.
You can toggle the Live keys and use them instead once you have tested the process.
Let’s look into the Ionic 5 integration of Stripe now.
Now we are ready to go……..!!
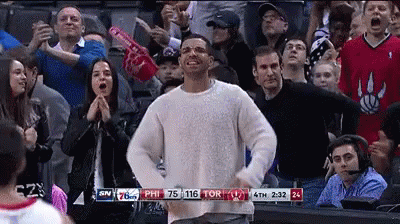
1. Adding customer to stripe
Before using any of the stripe’s API we have to first add the customer to the stripe server. This customer will have a customer, which will be used to have Customer Identity/Addresses, Add Cards, Charge Payments, etc
So for creating customers we can use different information that you are providing to your application — especially during the signup process. In this, we need to have the customer name and customer email address and pass it to our own server-side script (Node APIs OR Firebase cloud functions) which will call the Stripe APIs.
Mostly in our app flows, the stripe customer is generated at the time of registration of a user or at the time of first payment.
This is how your HTTP call in the Ionic app will look like whether it is a Node Server or Firebase Cloud functions APIs
this.http.post(`${this.url}/addCustomer`, {
cusName: user.name,
cusEmail: user.email,
})
Here this.url is the server URL that has our APIs — more on this later. We will pass the cusName(user name) and cusEmail(user email) in the body of the POST request.
a) Way 1 — Using Firebase Cloud Functions
Connect Firebase to your project
You will need to have the firebase CLI setup on your system. If you don’t have already use the below command to set it up
$ npm install firebase-tools -g
You can create a Firebase Project in the Firebase console webpage, connect it to the Ionic 5 app using firebase init
$ firebase init
then choose the project and choose functions
option from the choices — given in CLI.

With Firebase Functions, you can essentially write functions in the same environment, test locally with firebase serve
and can then deploy these to your Firebase project, so it can be connected to your app/website.
Once you have the Firebase project connected, you will see a functions
the folder in your project root, as shown below
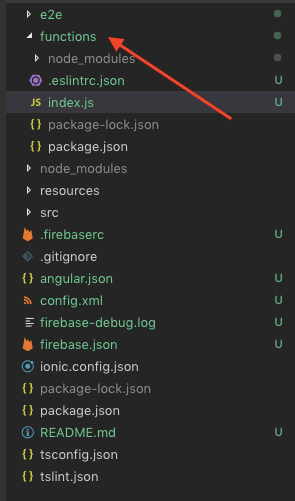
Create a firebase function to create customer
After this, you can create your first server function in functions/index.js
file. This function now will accept the customer information (as shown before) and should call the stripe server for the creation of the customer. Here the stripe library will be automatically installed by the firebase cloud — however, don’t forget to replace YOUR_SERVER_KEY
Here we will get the customer object in the response, and customerID can be stored in your database as the primary identifier for the customer. (Check the customer object in the section below)
b) Way 2— Using Node Express APIs
Here simply we create a REST API for handling the client's POST request on our own Node+ Express Server.
Here is the customer object response we will have —
We will use the “id”:”cus_HrWrKvNgklCDTL” for using other stripe APIs like adding a card and charging the customer.
As we can see both of the above approaches are almost the same and we have successfully added the customer to the stripe server and we are good to go and charge the customer.
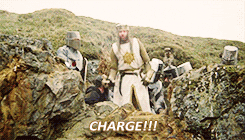
2. Adding Payment Methods
This step is important as many of us get confused over - how our app will save the user's cards. Often we think we have to manage user's cards in the database somehow. But let me tell you don’t have to manage the cards - Stripe will do this for you. We just simply save the above customerId in the user's collection. And use it for the APIs calls
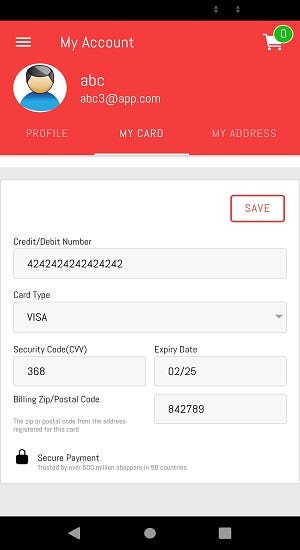
To add the payment card, we have to first generate the card token using the card information like card number, expiry month, expiry year, and the CVC code and pass it into a createCardToken function. It will return the card token. This whole code we run in the App (not on the server)
This piece of code will help us to generate the token for the card ( here you can try with some of the test cards provided by the Stripe.
Now we have generated the card token ( token represents the card itself ) and will pass this token and the customerId ( That we have generated above in step 1) to our Firebase cloud or the Node server.
Now we will call the server using a POST request in which we will pass the token and customerId in the body.
this.http.post(`${this.url}/addCard`, {
sourceToken: token,
userId: customer.id
})
Now we will add more code in our above script to enable the add Card feature.
a) Way 1 — Using Firebase Cloud Functions
We will now create the addCard API in the index.js of functions
folders in our project.
Here we are generating a source of payment (Payment Card) for a customer using the customer Id and passing card information into the source - via Token.
b) Way 2 — Using Node Express APIs
For this, we have to repeat the same step as above — according to the Express, and create a new endpoint of /addCard
.
This will return the complete information on the card.
Now we will learn how to get the saved cards for a customer.
3. Getting saved cards
To get the saved cards we only need the customerId (That we have saved in user collection/table). For this part I will only go through the code and minimu explanation.
this.http.post(`${this.url}/getAllCards`, {
custId: customer.id
})
Above shows a POST request which has only input as the customerID (we have this in step 1).
a) Way 1 — Using Firebase Cloud Functions
Here we just get a list of all sources (payment cards) for the customer using the listSources
API.
b) Way 2 — Using Node Express APIs
Similar kind of code we will use to create an Endpoint /getAllCards
on the express server.
This will give an Array of cards that will contain the card token in each object and — we will be using it for showing the list of cards as shown in the image.
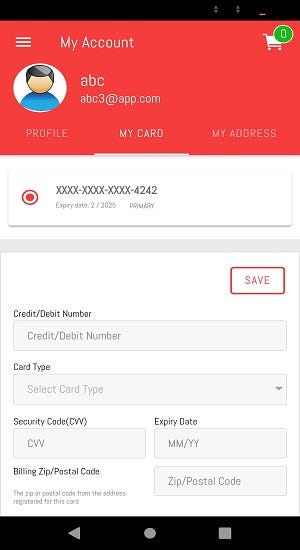
4. Charging the customer
Finally, we have reached our main goal — to charge the user. So in this part, we will use the paymentIntents API of the Stripe. We will need a set of values to make a successful payment.
We will need the following values to make it possible —
- Amount (The most important thing ..!)
- Currency (USD, EUR, INR, etc)
- Selected card token (Select card token from the array of card objects — this was returned in the previous section)
- CustomerId
- Shipping (Mandatory requirement for some countries, optional for others)
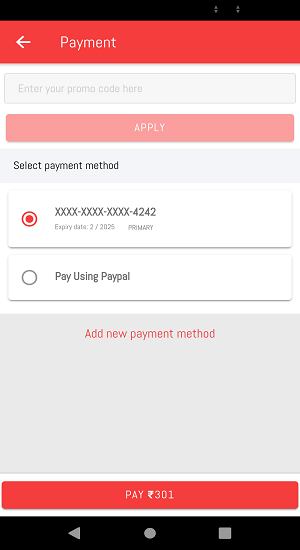
Make a POST request from the client containing all this information and let the server handle all of the things.
this.http.post(`${this.url}/charge`, {
amount,
currency,
token: card,
custId: stripeId,
shipping
})
Here you can pass in your own amount and currency but keep in mind if you are passing the shipping address then it will be of the following form —
const shipping = {
name: username,
address: {
line1: 'street',
city: 'locality',
postal_code: 'zipcode',
state: 'statecode',
country: 'IN'
}
};
Please check for the state code, country code etc. for their acceptable values. Now its server’s turn —
a) Way 1 — Using Firebase Cloud Functions
Here we create a payment Intent for the customer using the paymentIntents
API.
Here we just create a paymentIntent for the particular customer (Customer Id) by a selected card (CardToken).
b) Way 2 — Using Node Express APIs
Similar kind of code we will use to create an Endpoint /chage
on the express server.
It will charge the particular user and return a response object like this, if the payment is successful, look out for any error in response as they can be very helpful in debugging —
Finally this comes to an end….!!
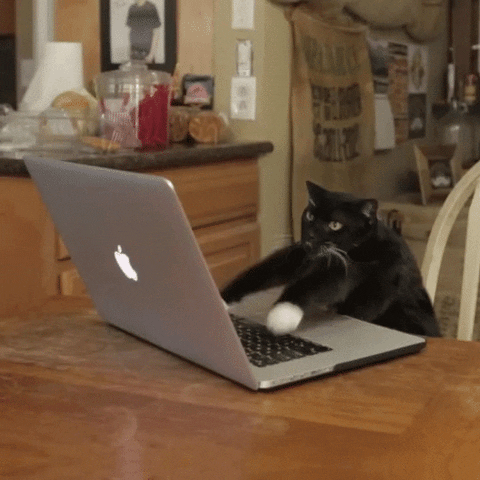
Conclusion :
So in this tutorial, we have learned about adding customers, adding cards, getting saved cards, and finally charging the customers. We have compared how similar both Firebase Cloud functions and Node Express API code looks. Its a matter of preference and system architecture that will guide your choice. If you want to be Scalable and ServerLess — Firebase Cloud functions is the way (may be a bit costly in the future), however, implementing your own Server is always provides more control in any scenario
Now you have enough knowledge to implement the stripe payment to your awesome Ionic application. If you want to add more payment gateways to your application you can go through Paypal integration blog.