Using Sentry — Error Monitoring with Ionic Angular Applications

In this tutorial, we will see how we can integrate Sentry — Error Monitoring with the Ionic Angular application.
Why Error Monitoring is Required? 🐞
Error tracking is quite important for every stage of product development. When your product first goes live — it is the scariest moment for the developers. Anything can go wrong from Devices compatibility, Network Issues to unknown ways users are using the app. To track which error was thrown in the live app is the best way to mitigate the issue in the early stages. And in today’s changing technological environment anything can go wrong — without any change from your side. A new operating system update, a new permission system by Smart Phone — the list is endless.
What is Sentry? 👮
Basically, Sentry is a Cloud Service for tracking the error in your application. It helps you debugging your app in development mode as well as in production mode. As per the definition provided by Sentry’s Site :
Self-hosted and cloud-based error monitoring that helps software teams discover, triage, and prioritize errors in real-time. It can help you to debug you problems in debug and production mode.
You can monitor your application errors in Sentry’s interface, categorize them as done or left, assign them to any of your Dev team members to fix it. Therefore not only it is an Error tracking System, but it is also a product management tool that helps the team to coordinate about incoming issues — without even being reported by users directly. Awesome 🤩
Integrating Sentry with the Ionic Angular app :
Few steps we will follow :
- Create a sentry account by visiting Sentry.io.
- Create an Ionic Angular application.
- Integrate the Sentry with the Ionic Angular application.
So lets start and go with the flow 🌊
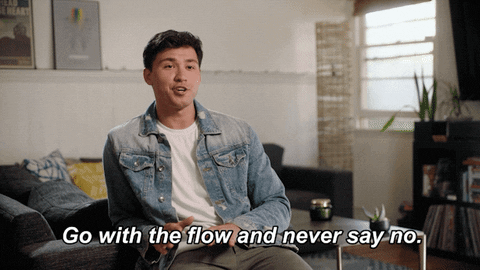
Step 1: Create a Sentry Account
For creating the account you can visit Sentry.io…!!
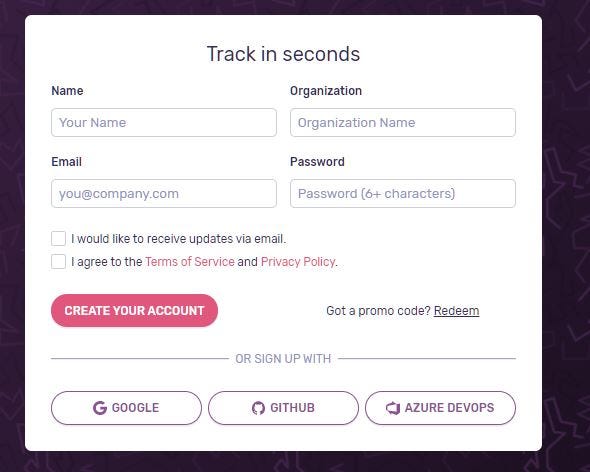
You can create a New Account or SignUp using any of the social platforms. This will create a new account where you can monitor errors of connected applications.
Now once you have created the account then you have to create a new project to connect it with the Ionic application.
At first, you will be asked to give your project a name
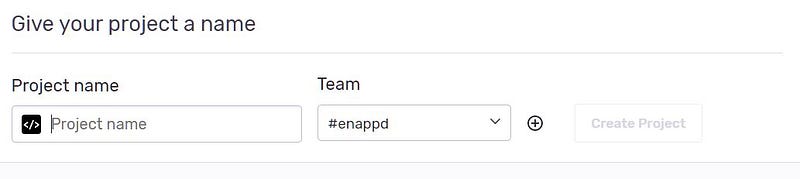
After that, you have to choose the platform with which you will integrate the sentry account like JavaScript, React Native, etc.
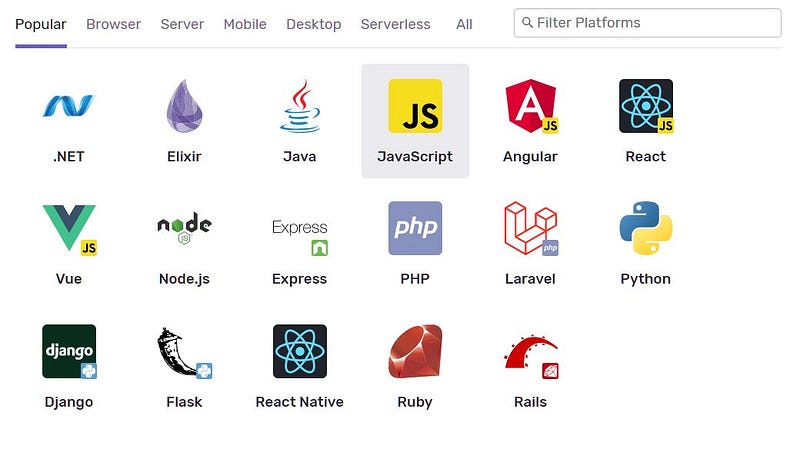
For integrating it with the Ionic application, you have to select JavaScript (or Angular also) as the platform and after that, you will see the instructions to integrate it with the application. (You can skip the integration instruction part as we will see it in detail)
Sentry provides us the interface where we can manage our actions on the errors like — Assigning work to teammates and categorizing the application errors. We will see all those later ⏳
Step 2: Create the Ionic application
To Create an Ionic Angular application, We can run the below command and if you want to learn in details - you can visit our blog HERE
ionic start sentry-integration blank
The above command will create the blank Ionic Angular application (sentry-integration) in the working directory
Step 3: Integrate the Sentry with Ionic Application
This is one of the most crucial steps — in this, we will see the main steps to integrate Sentry JS with the Ionic application.
Before we proceed you have to install the Sentry-Cordova packages and its plugin into your working project. To do that we can run the below command in the working directory.
$ npm i --save sentry-cordova
Now you can just add the platforms to your project (If you haven't done it yet). You can use the below command to add Android
$ ionic cordova platform add android
Once your platform is ready, we can add sentry-cordova plugin into our project. But keep in mind do not run the Ionic-CLI command like — ionic cordova plugin add… rather use the Cordova CLI command :
$ cordova plugin add sentry-cordova
The above commands complete the installation part.
After that, to add the Sentry JS we have to add a JS script CDN link to our index.html that resides in src. You can paste it inside the <head> tag. (You will get your own CDN JS link while initiating a Sentry project)
<script src="https://browser.sentry-cdn.com/5.27.1/bundle.tracing.min.js" integrity="*****************************************************" crossorigin="anonymous"> </script>
For configuring the source map creation in Ionic, you have to add the below lines of code to the package.json file
"config": {
"ionic_generate_source_map": "true"
}
To create the source map which will be uploaded to the sentry we have to run the below command in your terminal.
$ ionic build --prod --source-map
It will generate the source map files of your code which will be useful in debugging. Remember to run this command with every new version of your app. You can even replace this line — as your default build script in package.json ( I will let you do that on your own 😓)
After this, we have to add some Sentry Magic 🍄 into our main Ionic project and initialize our Sentry project in app.module.ts
import { NgModule, APP_INITIALIZER, ErrorHandler, } from '@angular/core'; | |
import { BrowserModule } from '@angular/platform-browser'; | |
import { RouteReuseStrategy } from '@angular/router'; | |
import { IonicModule, IonicRouteStrategy } from '@ionic/angular'; | |
import { SplashScreen } from '@ionic-native/splash-screen/ngx'; | |
import { StatusBar } from '@ionic-native/status-bar/ngx'; | |
import { AppComponent } from '@app/app.component'; | |
import { AppRoutingModule } from '@app/app-routing.module'; | |
import * as Sentry from 'sentry-cordova'; | |
import { SentryIonicErrorHandler } from './services/sentry-ionic-error-handler'; | |
Sentry.init({ | |
dsn: '**You own sentry URL**', | |
}); | |
@NgModule({ | |
declarations: [AppComponent], | |
entryComponents: [], | |
imports: [ | |
BrowserModule, | |
IonicModule.forRoot(), | |
], | |
exports: [], | |
providers: [ | |
{ provide: RouteReuseStrategy, useClass: IonicRouteStrategy }, | |
{ provide: ErrorHandler, useClass: SentryIonicErrorHandler }, // Intialize a error handler that will report to Sentry | |
], | |
bootstrap: [AppComponent] | |
}) | |
export class AppModule { } |
Here we have done a few things :
- Initialized the Sentry using the init method
- The DSN (used in initiating Sentry in your application) you will get while making the project in Sentry.io.
- Added the Error Handler class to providers part — the Application errors which pass it to the Sentry UI interface.
Error Handler Class
Let’s see Error Handler class generation and it functions in detail.
To generate the above Error Handler Class, use the below command to create a Class using Ionic CLI
$ ionic generate
This command will ask you for the path in the project:
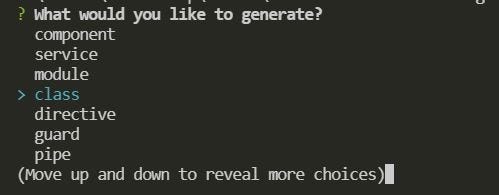

It will add the class file (sentry-ionic-error-handler) in your project folder, We can write the code for Handling complete errors in the Application and also passing them to the Sentry Interface — that will help us in tracking and managing the errors in our application.
import { ErrorHandler } from '@angular/core'; | |
import * as Sentry from 'sentry-cordova'; | |
export class SentryIonicErrorHandler implements ErrorHandler { | |
handleError(error) { | |
console.log('Error tracked Log', error.message); | |
try { | |
Sentry.captureException(error.originalError || error); | |
} catch (e) { | |
console.error(e); | |
} | |
throw error; | |
} | |
} |
This SentryIonicErrorHandler class is being added to the provider of the app.module.ts mentioned above as an ErrorHandler.
{ provide: ErrorHandler, useClass: SentryIonicErrorHandler },
If any error is Encountered by the app — then it will be redirected to the SentryIonicErrorHandler class. Here we have extended ErrorHandler interface that will help us by using its handleError method. Once the error is caught then we simply send it to the Sentry Server by using its captureException method (This exception will show up in the Sentry UI).
Now we are all set to run our application and will do it by running the below command.
$ ionic cordova run android
Caution ⚠️
You may or may not encounter the Build error like this, but we did

Problem:
sentry.properties does not exist in the project root!
This error may occur due to the command cordova plugin add sentry-cordova as it sometimes does not opens up the sentry-wizard, hence the sentry.properties file is not created automatically
Solution:
We just create the file with the name sentry.properties in your root project folder like the below structure.
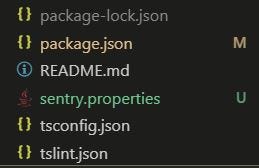
And in this file (sentry.properties), we mention some of the configuration code like the sentry base URL, project name (To which we want to connect), organization name (Used in creating the account), and the auth token.
defaults.url=https://sentry.io/ | |
defaults.org=your-organization-name | |
defaults.project=your-project-name | |
auth.token=your-auth-token |
Here you need to know your project name and your organization name that you have entered while signing up in Sentry. And to get your auth token you can visit HERE and copy the auth token and paste it into auth.token
Now you are all set to run your application and track errors and manage them into your teammates also.
$ ionic cordova run android
To check that setup and integration is working, we can make any code error (intentionally) so that we can track it using Sentry UI and use the further features of Sentry. For that, you can define an undefined (That does not contain the definition) function in app.component.ts constructor.
callingNotDefined();
Here we know that it will definitely cause an error, And it will be caught by the ErrorHandler Class and will be passed on to the Sentry UI.
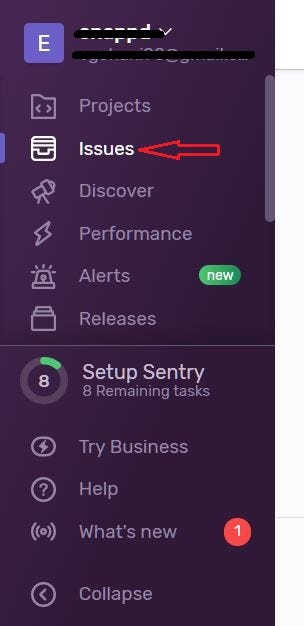
Once the Application is built Successfully, Then you can go to the Sentry Issues tab and check the stated entry there.
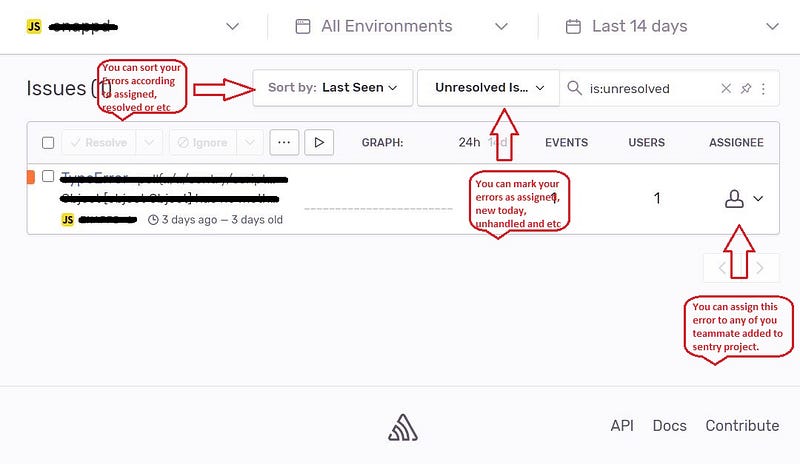
In the above image, you can see various management features of the Sentry that can help out a team to coordinate and make their product error-free and manageable. There are many more features to explore in Sentry.io
Conclusion
So this tutorial comes to an end, And we have seen how we can set up and integrate the Sentry with our Ionic application. You can also use it with any Angular Apps, but you will not need the Cordova plugins in those. Now you can create your own application (To add more features in your Ionic app you can visit our Blogs) and integrate it with Sentry to make error handling easy and manageable.