Using Background Geolocation in Ionic 5 Angular Apps
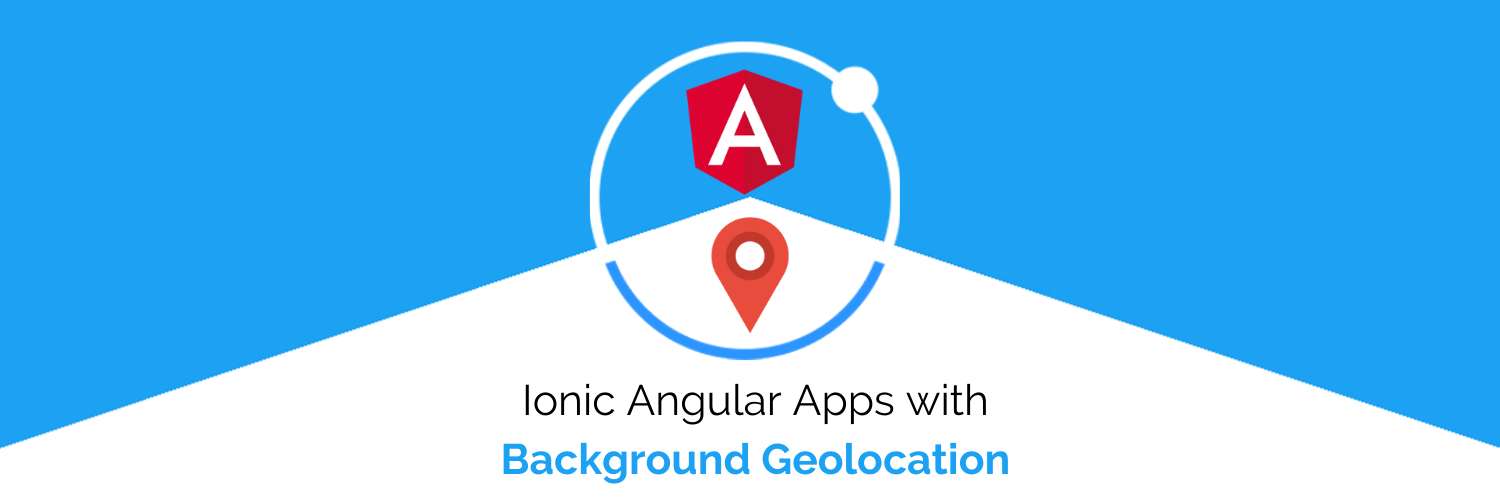
In this tutorial, we will how to implement background geolocation in the Ionic 5 application. And we will use Background Geolocation plugin to implement this.
You might have used geolocation in our project for getting the location of the user from device GPS and other network information. You might have also seen applications that track your location even when the app is not in the foreground. This is where the Background Location plugin comes in handy to track the user's location in the background also. Obviously, we should always take care of user’s privacy 😉.
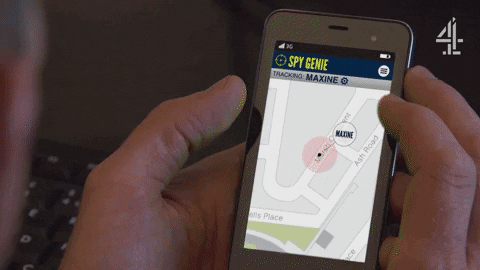
This type of tracking requires user permissions after the installation of the application. This type of plugin can be helpful in many projects where we need to track the user like Delivery Boy Application, Taxi Booking application, etc. So let’s just dive into the implementation 🤿
Step 1: Set up an Ionic Application
You can create a new Ionic 5 Angular project using the below command OR use any existing application. If you want to learn more about how to create Ionic Apps — you can go through our Beginners blog.
$ ionic start locationApp blank
This will create a new blank application in your working directory with the name locationApp. More details on how to create an Ionic App is in this blog
Step 2: Installing background geolocation Plugin
To install the plugin you just need to run the command given below, that will add the Cordova plugin and the native package to your Ionic application.
$ ionic cordova plugin add @mauron85/cordova-plugin-background- geolocation $ npm install @ionic-native/background-geolocation
Once you have installed the plugin and its package, we have to prepare the platform (Android or iOS). You can connect the physical device and run the command to check if the application is running without any errors.
ionic cordova run android
This will automatically prepare the platform for you and run the application on your physical device.
Note: At the current time, we have no way to install background geolocation plugin with new AndroidX compatible plugins. So you can face a few errors during the installation due to the conflict of support libraries. We are sharing a dedicated section to Solve these errors. If you don’t have such errors — You can SKIP the section
AndroidX related Errors
We have faced some of the errors and that you can solve using some solutions mentioned below, If your build ran successfully then you can skip this section and jump to the implementation section
- Failed to list versions for com.android.tools.build:gradle. Unable to load Maven meta-data from https://dl.google.com/dl/android/maven2/com/android/tools/build/gradle/maven-metadata.xml.
Solution:-
For solving this error we have to add the below code to the app/build.gradle and the project structure will look this
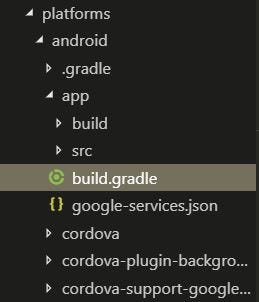
Now add the below lines of code to the build.gradle file shown in the above project structure
Now again run the ionic cordova run android command and you are good to go !!
2. AndroidX library migration error
This error occurs due to a conflict between the background location plugin and another plugin like fcm plugin (firebase cloud messaging plugin) or due to some other plugins. Due to conflict some of the v3 support libraries could not be changed to AndroidX, using simple migration. For this we will manually change the library in android studio
Solution:-
We have to open our project in the Android studio, by locating platform/android folder in it, then it will detect the Android framework now to get the error location we simply build the application and find the errors in the console.
Now we simply have to open the JAVA source code which is causing the error and replace the import statements with the library in AndroidX. You can do this replacement using the Class Mapping to AndroidX. This will help us to replace the included libraries to the new AndroidX libraries.
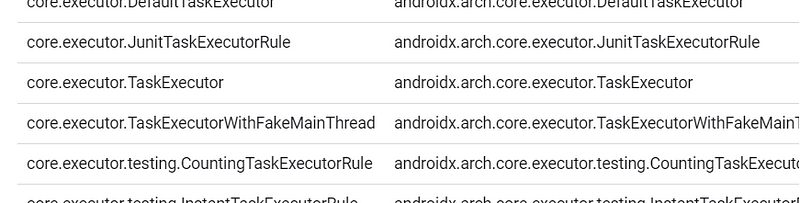
So, if we found an error in one of the old libraries which can be core.executor.TaskExecutor and simply replace it with the new AndroidX library which is androidx.arch.core.executor.TaskExecutor and so on. We have to do this mapping until all the errors are resolved.
Step 3: Implementation of Background Location
Once our application is successfully built, we can implement our logic and make the plugin do the work. We have to make sure — to initialize the Background Geolocation plugin before the Geolocation plugin as there can be some permissions issues due to this conflict.
First add the background location to the app.module.ts provider, So its instance should be available to the application. Now inject the Background GeoLocation service in the Home.page.ts (or wherever you want to use it) and write the further logic.
Here we define the BackgroundGeolocationConfig, according to the user's need as it helps to optimize the background tracking options, if you want to know more (completely) about the options you can go through this POST.
The app will ask for the permissions once the plugin is initialized and you can grant the permission so that plugin can work.
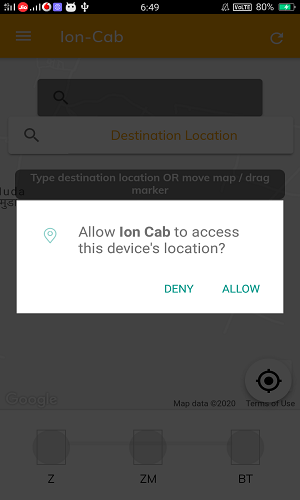
We can start the tracking of the location by calling the start() method, And stop the tracking using the stop() method of Service.
This plugin tracks the location continuously (unlike the geolocation plugin which works only when called and only in foreground) as the listener is being set until it is being cleared using the stop() method.
Once we start the tracking and if the application goes to the background, the Notification bar shows the background tracking tag.
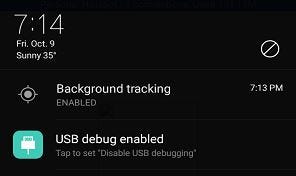
This shows that the application can also track when the application is in the background and help us to track user whenever required for the application. Success !! 🙌
You might have to write a logic to save all this data to your database and fetch it back via APIs to render in your application. But that’s something we can do by putting some logic.
Conclusion
In this tutorial, we have learned using the Background location plugin in the Ionic 5 application, which will help us to track the user location when the application is in the background.
- We saw how to set up the plugin
- Common errors you face in the Android platform.
- How to avoid conflicting permissions and properly initialize the plugin.
Now you are all ready to make your own awesome application having background tracking, you can also add many other features to your application by reading our blogs HERE.