Migrating Ionic Cordova to Capacitor application — Complete Guide
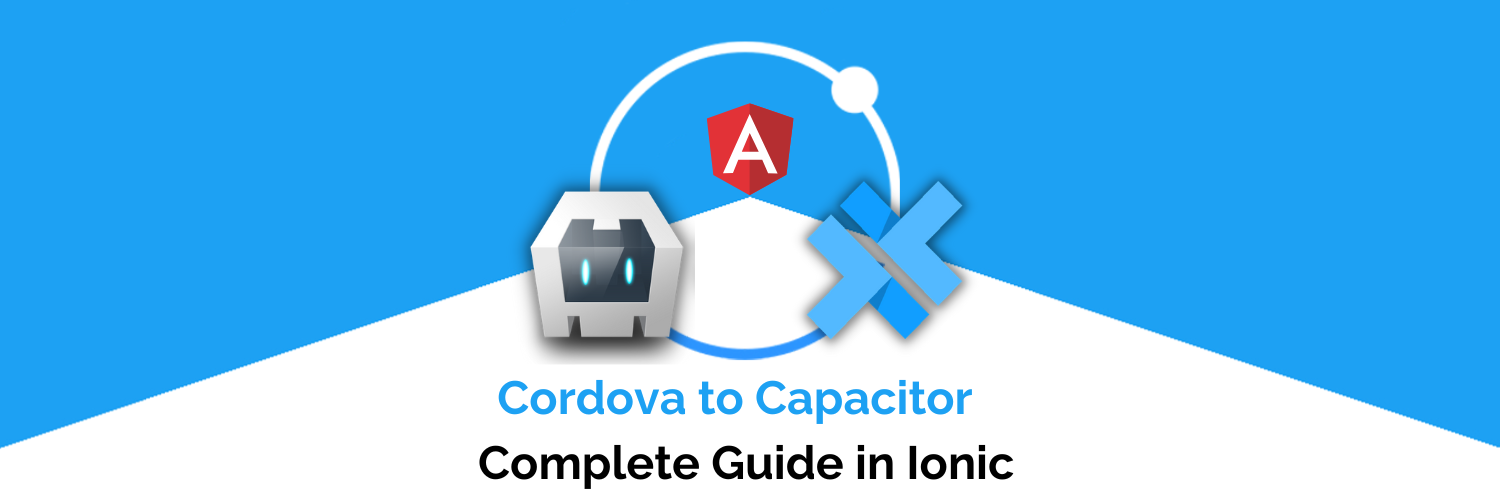
Here is the complete guide to converting the Ionic Cordova application to Capacitor (this article works best for Ionic version 5/6 Angular). These days most of the developers are moving to the Capacitor, as it has more stable plugins than Cordova, and Apache has stopped support for the Cordova platform. In this tutorial, we will not just go through the commands to convert Cordova to capacitor but the complete process of converting a Real life Cordova app to Capacitor. Yay !!
Capacitor — How is it different from Cordova?
This section is relevant to only those who have been working with Ionic / Cordova for some time. Cordova has been the only choice available for Ionic app developers for quite some time. Cordova helps build an Ionic web app into a device installable app.
Capacitor is very similar to Cordova, but with some key differences in the app workflow
Here are the differences between Cordova and Capacitor (You’ll appreciate these only if you have been using Cordova earlier, otherwise you can just skip this section)
- Capacitor considers each platform project a source asset instead of a build time asset. That means Capacitor wants you to keep the platform source code in the repository, unlike Cordova which always assumes that you will generate the platform code on build time
- Because of the above, Capacitor does not use
config.xml
or a similar custom configuration for platform settings. Instead, configuration changes are made by editingAndroidManifest.xml
for Android andInfo.plist
for Xcode - Capacitor does not “run on device” or emulate through the command line. Instead, such operations occur through the platform-specific IDE. So you cannot run an Ionic-capacitor app using a command like
ionic run ios
. You will have to run iOS apps using Xcode, and Android apps using Android studio - Since platform code is not a source asset, you can directly change the native code using Xcode or Android Studio. This give more flexibility to developers
In essence, Capacitor is like a fresh, more flexible version of Cordova.
Migrating Cordova to Capacitor
Before going on the steps for converting the Ionic Cordova app to Ionic Capacitor — we will look at the App code to be converted. Below is the package.json file for the Ionic Cordova project.
If you see the above package file, you will see there is a Cordova framework installed over Ionic. Now let’s start migrating Cordova to Capacitor.
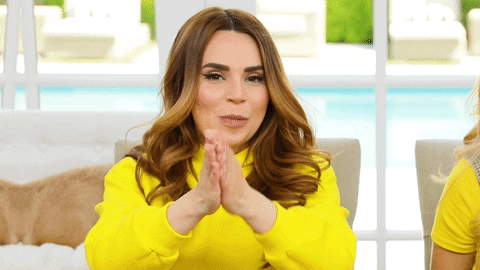
To migrate Cordova to Capacitor, you should have a Cordova project first (LAMMO 😂). Go to your project directory and run the below command to add the Capacitor traces to your Cordova application.
$ ionic integrations enable capacitor
The above command will initialize and add basic capacitor libraries to your Cordova project. There are some base plugins that are needed to run the app on the devices or browsers.
This command sometimes gives errors [in real life — always gives errors 🚨]. If it throws errors, then install the base plugin using the below command.
$ npm install @capacitor/app @capacitor/haptics @capacitor/keyboard @capacitor/status-bar
Now base plugins are installed, after that, we will initialize the capacitor using the below command
$ npx cap init
This command will ask you for some basic information related to App. Like the App name, and bundle ID. It will also ask if you want to copy plugin info from config.xml file, type ‘y’ for that.
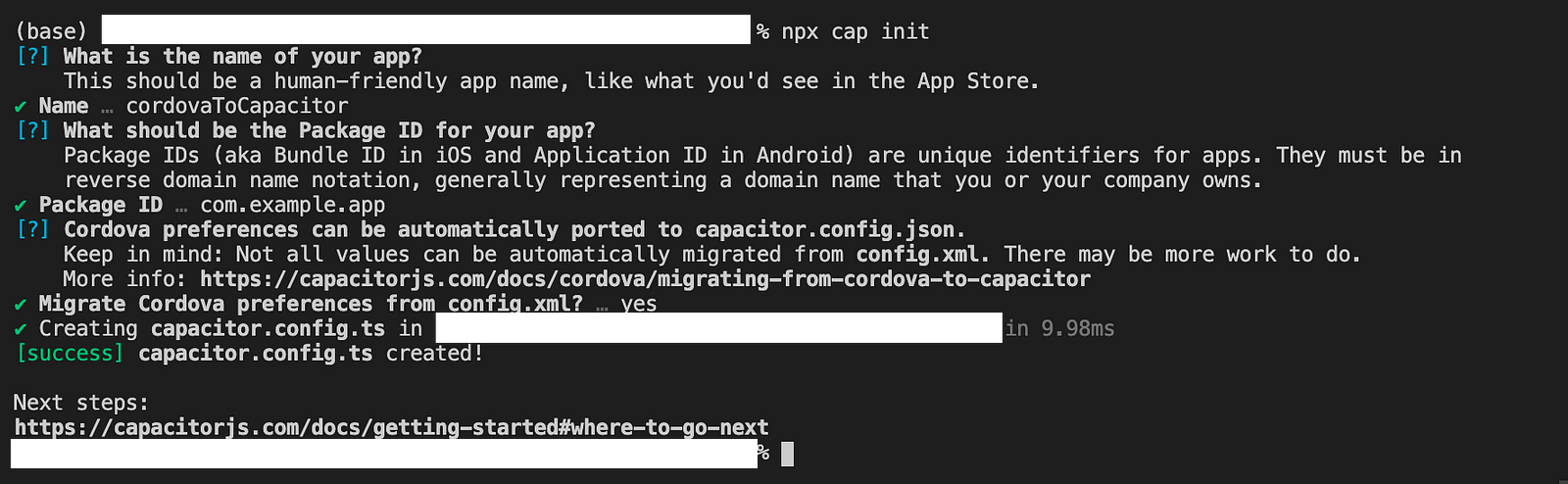
This will create ‘capacitor.config.ts’ file, it’s equivalent to config.xml file in the Cordova framework. Let’s have a look at capacitor.config.ts file and modify it for the capacitor framework.
Config file for Cordova setting
If you observe this file you will find a Cordova: {} block that contains information regarding Cordova plugins. But we don’t want Cordova now, so we will modify this file (capacitor.config.ts) for the capacitor.
Now we have added a plugins: {} block, that contains capacitor plugins information. Like Splash Screen, Push Notifications etc. plugins config (plugins {}) contains config of each plugin used in Ionic project. Capacitor can also have the Cordova plugin installed in it (but in our case we only want capacitor plugin to be used).
if you want to know more about how Config file is configured, the goto Capacitor config Schema.
Whenever you install a plugin, the plugin config needs to be added in this file. Like if you install Twitter plugin then its secret key will be put under config.
Note :- As we are initialising Capacitor 3, it needs capacitor.config.ts file rather than capacitor.config.json file (JSON file is supported by Capacitor 2 and older).
Now we are ready to take off. Before setting up Android and iOS platform, we will remove all the instances/plugin of Cordova and replace it with Capacitor. We will start this with cleaning up the package.json file. Here cleaning up means, removing all the Cordova existence from App (starting it with package.json file).
Note :- My way of removing cordova plugin from package.json file is to search for “cordova”and “@ionic-native” and remove all the strings or blocks related to it.
While removing Cordova plugin from package.json file, copy the removed plugin somewhere as we should know what plugin we need to add for capacitor. After removal of all the Cordova and ionic-native package.json will look like :-
But you must be feeling, our application is on state of complete break down. Yes, if you try to run ionic serve, you will see it will give lot of error. But wait for a while, our app will be fixed up soon.
After adding Capacitor to the application, we have to follow 3 steps:-
- Removing Cordova and ionic-native code usage instances from the Ionic project.
- Installing capacitor plugins
- Replacing Cordova plugin code with capacitor plugin code.
Note :- This part will differ for each of the application, as every application uses same or the different plugins.
For removing the Cordova and ionic-native instances, we can search for ionic-native and remove all the instances of it. I will take one example of removing ionic-native and Cordova instances of the Cordova push plugin and adding a new alternative to the Capacitor Push notification plugin.
To remove and replace the Plugin, we will follow the below steps:-
Step 1 — Removing Cordova and ionic-native code usage instances from the Ionic project
Remove initialization of Cordova push plugin from app.module.ts :- Goto app.module.ts, remove the import statement of push plugin and also remove the injection of Push module from the providers. You have to remove the below-mentioned lines from the file.
import { FCM } from '@ionic-native/fcm/ngx'; - Remove FCM import statement
Providers: [ ..., FCM // Remove injection of FCM module ]
Now we have to remove it from Implementation file as well. Goto the file where your push plugin is imported. [Simply search for “import { FCM } from ‘@ionic-native/fcm/ngx’”]
Once you are on the page, remove the import statement and remove all the existence of that Push Plugin. Our code is in such a place where we do have push plugin implemented.
Step 2— Installing capacitor plugins
Implementing Capacitor Push plugin (Replacing Cordova Push Plugin) :- Its just a simple step, you can go toCapacitor Plugins page and search for the plugin you want to use. Before using it we have to install the push plugin using the below code.
$ npm install @capacitor/push-notifications
Step 3— Replacing Cordova plugin code with capacitor plugin code.
Capacitor plugins are easier to use as we do not have to inject them. In our case, we have used the push plugin, so add the below logic accordingly to your application. Put this code in place of your old Cordova-based plugin code.
import { PushNotifications } from '@capacitor/push-notifications';
const addListeners = async () => { await PushNotifications.addListener('registration', token => { console.info('Registration token: ', token.value); }); await PushNotifications.addListener('registrationError', err => { console.error('Registration error: ', err.error); }); await PushNotifications.addListener('pushNotificationReceived', notification => { console.log('Push notification received: ', notification); }); await PushNotifications.addListener('pushNotificationActionPerformed', notification => { console.log('Push notification action performed', notification.actionId, notification.inputValue); }); }
const registerNotifications = async () => { let permStatus = await PushNotifications.checkPermissions(); if (permStatus.receive === 'prompt') { permStatus = await PushNotifications.requestPermissions(); } if (permStatus.receive !== 'granted') { throw new Error('User denied permissions!'); } await PushNotifications.register(); }
Like this, we can replace a Cordova plugin with a Capacitor plugin. This would be applicable for all of the plugins that need to be replaced with the Capacitor plugin.
Now our application is completely migrated to the Capacitor framework. And we can add android and iOS platforms to it by running the below command:-
// Creating www build folder
$ ionic build
// Installing android and ios packages
$ npm install @capacitor/android $ npm install @capacitor/ios
// Adding iOS and android platform to the Ionic app
$ npx cap add android $ npx cap add ios
Bonus : Using a Cordova plugin with Capacitor 🎉
You will find many of the plugins are not yet available in Capacitor but are available in Cordova. So, How to set the Cordova in a Capacitor app ? what to do in that situation?; we can also use Cordova plugins in the Ionic capacitor application. Let’s take an example, if you want to add a plugin to pick contacts from the contact book then the capacitor does not have any option for that but we have a Cordova alternative for it (cordova-plugin-contacts). Run the below command to install the contacts plugin
$ npm install cordova-plugin-contacts $ npm install @ionic-native/contacts
Once the plugin is installed, we have to inject it into module.ts file as shown below.
import { Contacts } from '@ionic-native/contacts/ngx'; - Importing Contacts
Providers: [ ..., Contacts // Adding Contacts module as provider ]
Now for using it, we can simply import the plugin in the component.ts or page.ts and inject the Contacts module.
import { Contacts } from '@ionic-native/contacts/ngx';
constructor(private contacts: Contacts) {}
pickContacts() { this.contacts.pickContact() }
Conclusion
Now we have successfully converted the Ionic Cordova application to the Ionic Capacitor application. Now the Ionic world is moving to the capacitor, as it has a more stable environment and does not cause any installation issues. You also found — How to set the Cordova plugins in a Capacitor app.
Next Steps
If you liked this blog, you will also find the following Ionic blogs interesting and helpful. Feel free to ask any questions in the comment section
- Ionic Payment Gateways — Stripe | PayPal | Apple Pay | RazorPay
- Ionic Charts with — Google Charts| HighCharts | d3.js | Chart.js
- Ionic Social Logins — Facebook | Google | Twitter
- Ionic Authentications — Via Email | Anonymous
- Ionic Features — Geolocation | QR Code reader| Pedometer
- Media in Ionic — Audio | Video | Image Picker | Image Cropper
- Ionic Essentials — Native Storage | Translations | RTL
- Ionic messaging — Firebase Push | Reading SMS
- Ionic with Firebase — Basics | Hosting and DB | Cloud functions
Ionic React Full App with Capacitor
If you need a base to start your next Ionic 5 React Capacitor app, you can make your next awesome app using Ionic 5 React Full App in Capacitor
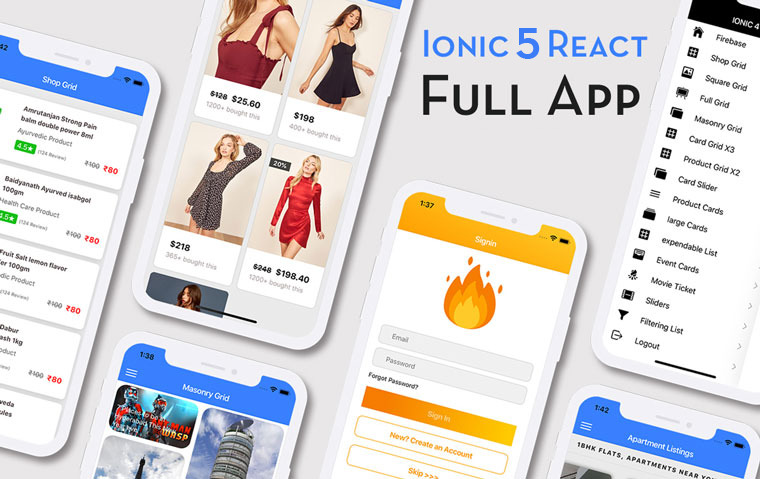
Ionic Capacitor Full App (Angular)
If you need a base to start your next Angular Capacitor app, you can make your next awesome app using Capacitor Full App
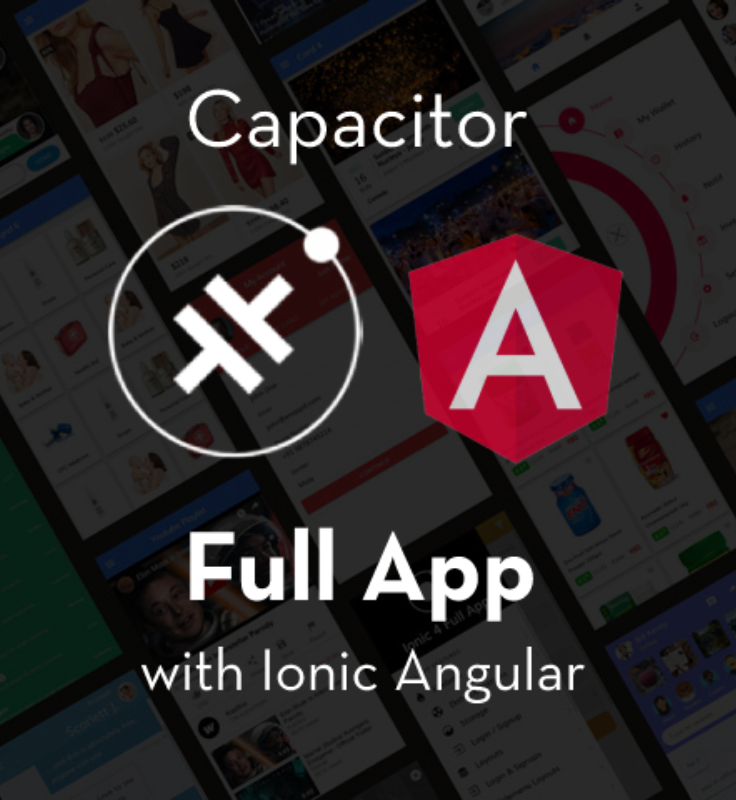
Ionic Full App (Angular and Cordova)
If you need a base to start your next Ionic 5 app, you can make your next awesome app using Ionic 5 Full App
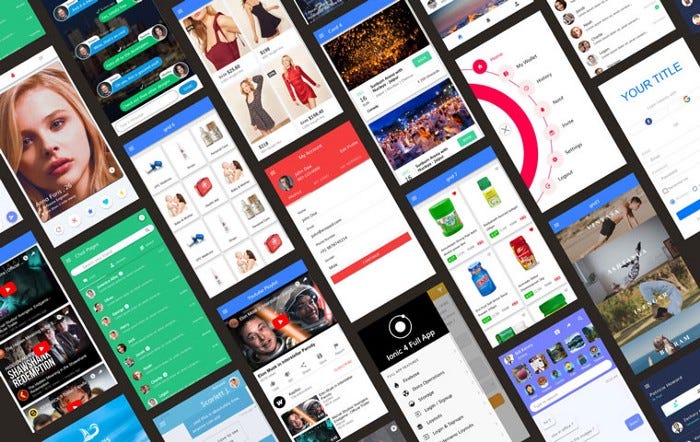