Detect Network in Ionic 5 application — WiFi and data connections

You may have come across situations in which, if your network/Internet goes off, the application starts up showing “No Internet” prompt or similar message to you — something like the image below
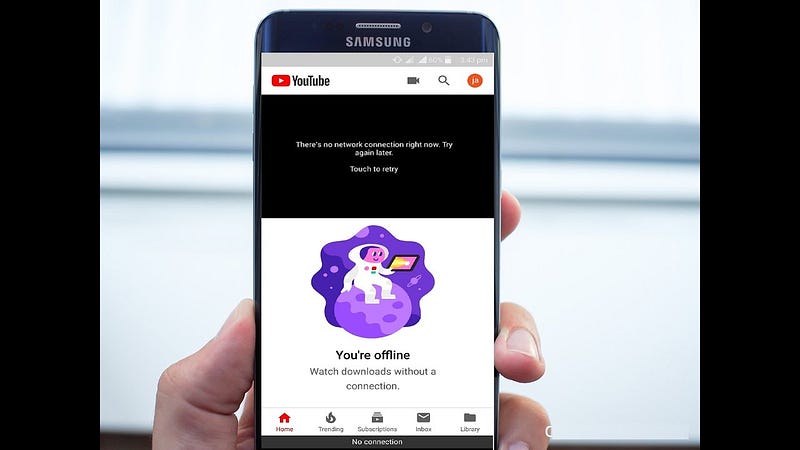
You must have wondered how it can be applied to your Ionic 5 application. So in this tutorial we will see how we can add a new feature i.e. Network detection to our Ionic 5 application. We will go step by step and will be learning every line of code in depth. So let’s start … !!
A word on Ionic Framework
You probably already know about Ionic, but I’m putting it here just for the sake of beginners.
To be concise — If you create Native apps in Android, you code in Java/Kotlin. If you create Native apps in iOS, you code in Obj-C/Swift. Both of these are powerful but complex languages. With Ionic you can write a single piece of code for your app that can run on both iOS and Android (and browser!), that too with the simplicity of HTML, CSS, and JS.
Forms of Ionic
Ionic has made itself language agnostic to some extent, integrating some of the most popular JS frameworks. At present, Ionic apps can be written with
- AngularJS as front-end ✅
- ReactJS as front-end
- VanillaJS as front-end
- VueJS as front-end
- Cordova as build environment ✅
- Capacitor as build environment
As you can see, Ionic can be deployed in various environments. This tutorial is based on Angular with Cordova, but the functionality remains similar in other environments as well (only the associated plugins change)
So let’s jump right in.
Post Structure
As always, we’ll go step by step so everyone — from beginners to existing developers — can follow the tutorial.
- Create a fresh Ionic project
- Install Network Check plugin
- Implement Network Check logic
- Detect what type of Network is connected
- Test the app in device
Step 1 — Create Ionic Project
To create a new Ionic project you can run below command.
$ ionic start networkApp blank --type=angular --cordova
(If you want to know more about creating Ionic 5 app you can visit — How to create Ionic app (for beginners))
The above command will add a blank project to the working directory with name = networkApp.
--type=angular
tells the CLI to setup Angular app, and --cordova
integrates Cordova with the app.
Step 2— Implementing Network Checking Feature
In this step, we will implement the logic to detect the network availability in the Ionic application. To do that we will use the cordova-plugin-network-information plugin. Install the plugin using following commands
$ ionic cordova plugin add cordova-plugin-network-information $ npm install @ionic-native/network
The above commands will install the plugin and native package to your Ionic application and after that, you have to add a platform to the Ionic project. You need to add platform because we can check network functionality only when the app is run on a device (android/iOS). To add an Android platform you can run the below command
$ ionic cordova platform add android
This command will add the android platform to the Ionic project. Now we are done with the setup and will start implementing the logic.
Step 3 — Implement Network checking logic
Define the Network module in app.module.ts
like below :-
Now this Network Module will be available to the entire application i.e. you can use this Module in any page of your application. Next, we will add the code to detect the network loss from the device.
Before that, we need to understand one thing that internet can disconnect at any stage of the application, so we need to apply that detection method somewhere globally in the Ionic application so that it can be called from any stage of the app i.e. we should use the detection method in app.component.ts
In intializeApp()
method, we call the checkInternetConnection()
that will check for the internet availability. Let’s go through each piece of code in the method.
this.disconnectSubscription = this.network.onDisconnect().subscribe(async () => { console.log('network was disconnected :-('); this.networkAlert = await this.util.createAlert('No Internet', false, 'Please Check you internet Connection and try again',{ text: '', role: '', cssClass: 'secondary', handler: async () => {} }); this.networkAlert.present(); }); this.connectSubscription = this.network.onConnect().subscribe(() => { console.log('network connected!'); if(this.networkAlert) { this.networkAlert.dismiss(); this.checkUser(); } });
In above code we set two subscriptions (Subscriptions are type of Observables that keep an eye on changes until they are unsubscribed i.e. if any change is observed then it is served under Subscribe
). One subscription is for onDisconnect()
and other is onConnect()
onDisconnect()
method keeps track if internet goes off while surfing the application. We simply shows the Alert with Message “No internet connection”onConnect()
method is called when internet is connected again after disconnecting. So this method can be used to recover the application state at which it was left while disconnecting.
For our demo purpose, we will simply show the Alert with the “No Internet” message on dis-connection and remove the Alert when internet is re-connected.
To show alert we call the createAlert
method defined under UtilService file (To generate the service file you can run ionic generate service utilService
).
Add the below lines of code in utilService file :-
async createAlert(header, backdropDismiss, message, buttonOptions1, buttonOptions2?): Promise<HTMLIonAlertElement> { const alert = await this.alertCtrl.create({ header, backdropDismiss, message, buttons: !buttonOptions2 ? [buttonOptions1] : [buttonOptions1, buttonOptions2] }); return alert; }
This above code will return an Alert instance, which can be presented when internet goes off. And this Alert is dismissed once Internet connects again.
Step 4 — Detect the network type
You can also detect if your app is connected with WiFi or data connection. This can be useful when you want to warn the user to not download large files on data connection. You can also automatically start scheduled downloads once the app detects a WiFi connection.
To detect the network type, we simply use the type class variable of the network class instance. below is the code we can detect the network type as wifi :-
let connectSubscription = this.network.onConnect().subscribe(() => {
console.log('network connected!');
setTimeout(() => {
if (this.network.type === 'wifi') {
console.log('we got a wifi connection, woohoo!');
}
}, 300);
});
Above code sets an onConnect()
listener in the app, when the app again connects to the internet then we can check for the type of connection using this.network.type . This happens even when your network switches from Wifi to data, or the other way.
To get the correct type you might have to delay the checking of the type variable (I have done it using setTimeout
method). Now we can perform any conditional operation using this type variable.
Step 5— Test the app in device
Below is the demonstration video, using the above logic in an existing application on the android operating system :-
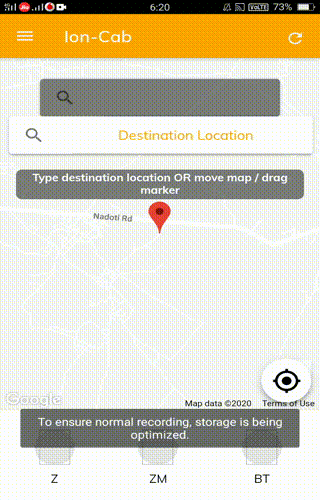
Conclusion
That is it! We just learnt how to add Network detection feature to our Ionic 5 apps, as well as how to detect the type of connection we have. It will help the application to maintain consistency even if internet goes off, or changes between WiFi and phone data. It will give a smooth experience to the application users.
Using this feature, you can
- Alert user on disconnection, and ask to reconnect
- Ask user to go offline and use app with limited functionality
- Ask user to selectively download items which consume heavy data, like Facebook and Youtube does
- Automatically change video/audio frame rate based on whether you’re on data or Wifi
- Allow payments only on stable network i.e. Wifi
- For a chat app, when user app goes offline, store the chats sent in local storage, and on re-connection, resolve the incoming chats and locally stored chats to give a seamless and temporally correct message stream
- And much more ….
Happy coding !
For latest blogs on Ionic and other app development, visit our blogs.