Implement Sounds and Vibration in Ionic apps
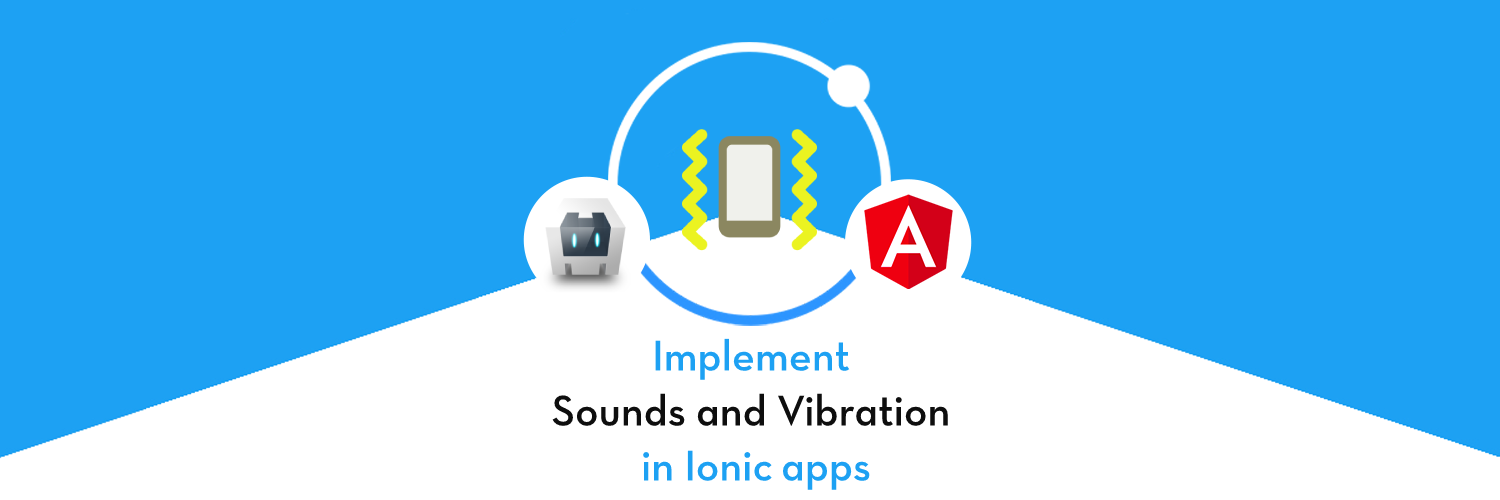
In this tutorial we will be learning how we can enable the sound and vibration feature in Ionic 5 application. According to the audio mode of the phone (Silent/ Vibrate/ Normal), we will decide to ring or vibrate the mobile phone. This can be for a notification, any in-app user activity or something else.
You must have came across some situation in which you have to handle push notification (Want to know more about push notification in Ionic ? You can read this blog) alerts in such a way that you want to alert user with some vibrate patterns or sounds, but you do not want to annoy the user. In such situation you can leave this decision on user whether they want the vibration or sound on notification, and also give a choice of which sounds the user wants on notifications.
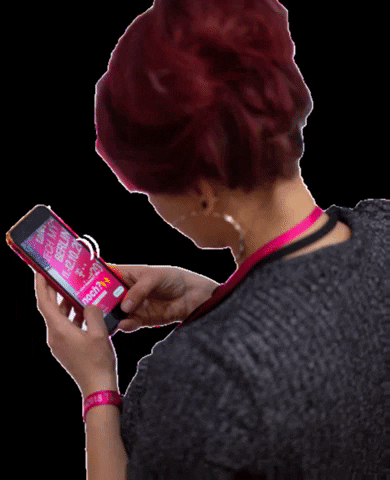
Ionic with Cordova
If you are reading this blog, you are probably already aware of Ionic and Cordova frameworks. While Ionic is the front-end wrapper framework for mobile UI, Cordova is the build framework that converts Ionic code into a device (Android /iOS) compatible code.
Capacitor is another option in place of Cordova to build apps using Ionic. While Capacitor is born couple of years ago, Cordova has been around for a decade. That’s the reason Cordova has more community made plugins for various unique features. Capacitor is quickly catching up as well.
Initial setup
Generally in all our blogs, we start with creating blank Ionic app, so a new come or beginner can also try the feature. To create an Ionic Angular Project you just have to run one command and that’s it ! Rest of the things are handled by the Ionic-CLI. Run the below command in you terminal.
$ ionic start phone-auth blank --type=angular --cordova
The --type=angular
told the CLI to create an Angular app, not a React app. And --cordova
tells the CLI to integrate Cordova support by default. You can also use --capacitor
instead of Cordova.
( My environment for this blog is — Node 14.x, Ionic 5.5, Angular 11.x, NPM 7.x, Cordova 10.0 )
This will create an empty ionic project in the working directory of your pc. For more details on creating a new Ionic app from scratch, check out our blog on Ionic Appshere.
Setup sound & vibration plugins
To achieve this dynamic behavior, we have to integrate some of the Cordova plugins and make this functionality work. We will basically do the following
- Use one plugin to find out phone’s current audio mode and we will decide whether to vibrate or ring the users mobile.
- Once we have the audio mode, we can vibrate (using a plugin) or ring (using a plugin).
In short, there are multiple plugins involved.
1. Cordova Audio Management Plugin
To know about the audio mode we want the audio-management plugin
$ ionic cordova plugin add clovelced-plugin-audiomanagement $ npm install @ionic-native/audio-management
Now we want some plugins to perform the vibrate and ring gestures, So for that we have to use Vibration plugin and Native Audio Plugin.
2. Cordova Vibration Plugin
To implement vibration, we install Cordova Vibration plugin
$ ionic cordova plugin add cordova-plugin-vibration $ npm install @ionic-native/vibration
3. Cordova Native Audio Plugin
To implement audio related functionality, we install Cordova native audio plugin
$ ionic cordova plugin add cordova-plugin-nativeaudio $ npm install @ionic-native/native-audio
Now we are done with installation part and now we can start to code for implementing the vibrate/ring feature.
Implementation
So before doing anything we have to inject all of the Modules in the app.module.ts
, so that it will be available for the complete application we can do it by adding it to Providers
Create basic UI
I will create a basic UI to test the functionality mentioned in this post. This is a very basic UI created with basic Ionic elements, no special CSS. Here’s how it looks.
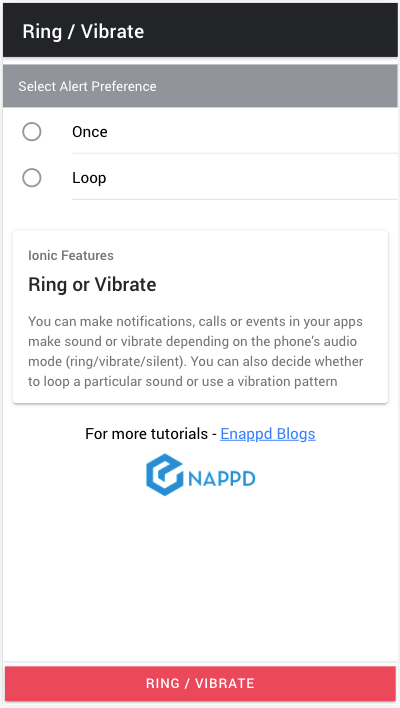
- Ring or Vibrate — This is selected based on phone’s audio mode
- Once / Loop — will be selected based on user’s input in the above UI
Here’s the HTML code for the UI
Methods for Sound and Vibration
We will implement a use case where clicking the button Ring/Vibrate will create an alert. Based on user’s audio mode (Ring/Silence/Vibrate) and repeat preference (once/loop), this action will create an audio or vibration alert. And we will dismiss the vibration or Ring by clicking in alert button.
First, we preload the audio file when the page loads. This avoids time lag for sound loading when the alert happens. Here’s the code to implement what we want
In the above code —
Preload audio track
We have initialized the ID for the ringtone, preloadSimple
takes the unique Id and path to the ringtone as the input and loads this ringtone with ID. Ringtone can be put under the assets folder that will be later loaded to the www folder of the project after the application is build.
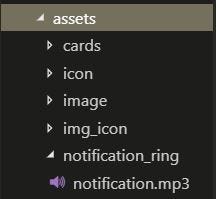
You can take any custom ringtone and load it in the assets folder and rest the plugin will handle automatically. Now once ringtone is loaded you can play the ringtone using module other methods.
Find device’s audio mode
getAudioMode
method checks the device’s audio mode. This function will decide whether the mobile phone is silent, vibrate or normal, The getAudioMode()
method of audio management plugin will return one of the following code according to the device status
AudioManagement.AudioMode.SILENT
= 0AudioManagement.AudioMode.VIBRATE
= 1AudioManagement.AudioMode.NORMAL
= 2
Note, (value.audioMode == 0 || value.audioMode == 1)
condition will return true for both Silent and Vibrate mode of the device. Otherwise you can create one more condition for Silent mode separately.
Select repeat mode
Next, we check the repeat mode of the alert based on user’s input — Once or Loop. Based on that we run audio or vibrate functionality.
- To play sound once —
playSingle
function withthis.nativeAudio.play()
- To play sound in loop —
playLoop
function withthis.nativeAudio.loop()
- To vibrate once —
this.vibration.vibrate(1000)
where 1000 is the duration in millisecond for vibration to happen - To vibrate in a pattern (or loop) —
this.vibration.vibrate([1000,500,1000])
— where vibration will happen for 1000ms, then 500ms gap, then 1000ms vibration. You can enter as many array elements as you need, or create a loop for the same
In short, either of repeat modes (once/loop) can be called to play the ringtone or vibration.
Stop sound or vibration
In the above code, the alert is shown after sound/vibration is played. The alert has one button, clicking which stops the sound or vibration. The alert is shown with showAlert
function, and is a default method of Ionic framework
- To stop sound playback —
this.nativeAudio.stop(‘uniqueId1’)
- To stop vibration —
this.vibration.vibrate(0)
Stopping the sound or vibration will stop it irrespective of whether it was Once or on Loop.
Testing on device
For ease of build, let’s build the code on Android and test. To build the code for Android run following commands
$ ionic cordova platform add android //adds android platform
$ ionic cordova build android //builds the APK
$ ionic cordova run android //runs on attached device or simulator
// OR run a live build in device for making quick changes $ ionic cordova run android -l
This will build the application on the device of emulator. Now set the sound mode of device as you like and test the functionality. This should look something like this (watch out for sound, vibration sound is very feeble)
Conclusion
So we have learnt how we can add a ring/vibrate feature to our Ionic 5 application in just few steps. Now you are ready to make your own awesome application with sounds/vibration happening at various actions, notifications and other events.