Introduction to Ionic Vue — Beginners Guide
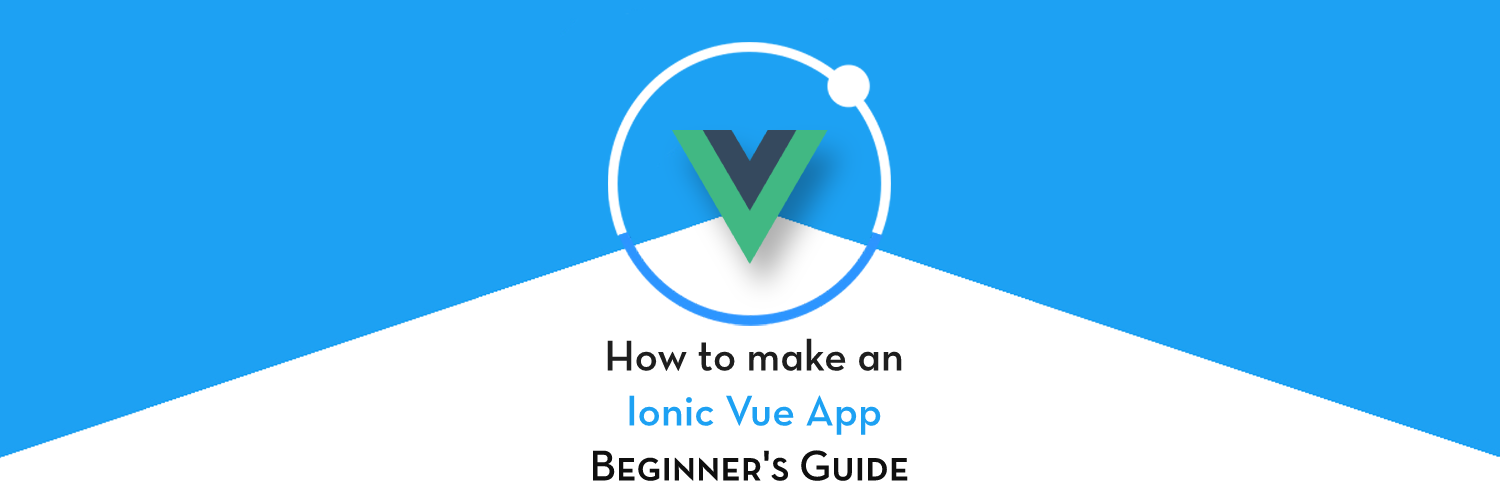
In this tutorial, we will make a new Ionic application with Vue as a support language. We know that Ionic application can be developed using React and Angular (You want to know more about it, you can visit this blog). But In this tutorial we will experiment an all new Vue framework. In Ionic, Vue by default integrates with Capacitor. So you can say we are writing THE most modern Ionic application which is Ionic Vue + capacitor.
What is Ionic?
Ionic is a complete open-source SDK for hybrid mobile app development. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices.
We can use Cordova or Capacitor to build these awesome apps and have native functionalities. With these, we get some amazing native plugins to use like Image cropper, Camera, and many more.
Forms of Ionic
Ionic has made itself language agnostic to some extent, integrating some of the most popular JS frameworks. At present, Ionic apps can be written with
- AngularJS as front-end
- ReactJS as front-end
- VanillaJS as front-end
- VueJS as front-end ✅
- Cordova as build environment
- Capacitor as build environment ✅
As you can see, Ionic can be adopted by a large community of developers, and hence there are always confusion about which plugin to use in which framework. This post will only talk about the basics of an Ionic Vue app.
Capacitor — How is it different from Cordova ?
This section is relevant to only those who have been working with Ionic / Cordova for some time. Cordova has been the only choice available for Ionic app developers for quite some time. Cordova helps build Ionic web app into a device installable app.
Capacitor is very similar to Cordova, but with some key differences in the app workflow
Here are the differences between Cordova and Capacitor (You’ll appreciate these only if you have been using Cordova earlier, otherwise you can just skip)
- Capacitor considers each platform project a source asset instead of a build time asset. That means, Capacitor wants you to keep the platform source code in the repository, unlike Cordova which always assumes that you will generate the platform code on build time
- Because of the above, Capacitor does not use
config.xml
or a similar custom configuration for platform settings. Instead, configuration changes are made by editingAndroidManifest.xml
for Android andInfo.plist
for Xcode - Capacitor does not “run on device” or emulate through the command line. Instead, such operations occur through the platform-specific IDE. So you cannot run an Ionic-capacitor app using a command like
ionic run ios
. You will have to run iOS apps using Xcode, and Android apps using Android studio - Since platform code is not a source asset, you can directly change the native code using Xcode or Android Studio. This give more flexibility to developers
In essence, Capacitor is like a fresh, more flexible version of Cordova. Ionic apps made in React and Vue, by default, integrate with Capacitor.
What is Vue ?
Vue is a progressive framework for building user interfaces. Unlike other monolithic frameworks, Vue is designed from the ground up to be incrementally adoptable. The core library is focused on the view layer only, and is easy to pick up and integrate with other libraries or existing projects. On the other hand, Vue is also perfectly capable of powering sophisticated Single-Page Applications when used in combination with modern tooling and supporting libraries.
If you have been working with React and/or Angular, Vue is like a combination of both. Read more about Vue on the official documentation page.
Creating Ionic Vue Project
Ionic Vue is built on Vue 3.0, which is the latest version. If you have worked on Vue 2.0, Vue 3.0 is only slightly different. But Ionic has wrapped Vue in its own way, which is efficient for making apps, and in alignment with Ionic’s syntax in other front-end frameworks.
To create Ionic Vue project, you have to follow same steps as for Ionic React or Angular. But keep in mind that you have the latest version of Ionic-cli as Vue is updated in newer version (Ionic-cli V6.12.2 — At the time of blog). You can update ionic-cli using below command :-
$ npm install -g @ionic/cli
Once the update is complete, Now we can create new Ionic + vue application. To create you can run below command :-
$ ionic start projectName blank --type=vue
Above command contains the name of the project and you can pass in blank
, tabs
to choose the layout of the Ionic Vue project. In the end, we have given--type=vue
as the type of language.
Once the command finishes, you can run this to start the app on browser
$ ionic serve
It will give the standard Ionic start screen, which you can edit easily, or add more pages and functionality to. Here’s the default home screen.
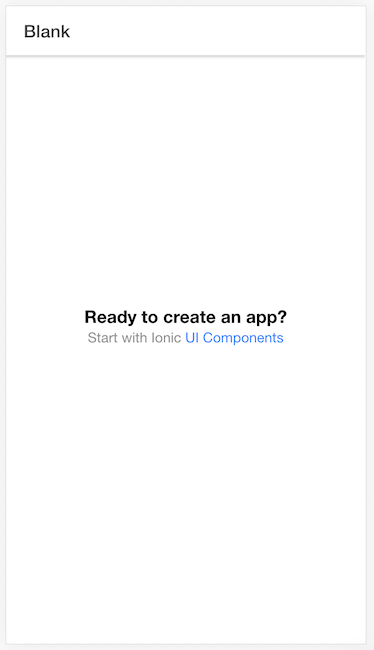
Folder structure
The start
command will create a new project in the working directory which will have a folder structure like this :-
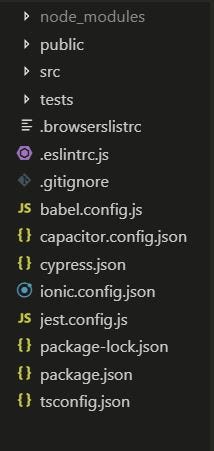
The project folder structure is petty similar to Ionic React and Ionic Angular. In this structure, src
contains the project logical files like router files, theme files and view files.
App.vue
contains the main instance of Vue, and the components of Ionic that go in the main instance. Ionic has designed ionic-vue
in a way that the terminologies stay the same across different frameworks, namely Angular, React and Vue. Here’s a snippet of App.vue
where IonApp
and IonRouterOutlet
components are added to main Vue instance
<template> <ion-app> <ion-router-outlet /> </ion-app> </template>
<script lang="ts"> import { IonApp, IonRouterOutlet } from '@ionic/vue'; import { defineComponent } from 'vue';
export default defineComponent({ name: 'App', components: { IonApp, IonRouterOutlet } }); </script>
Essentially IonApp
is the parent component, and IonRouterOutlet
is defined inside it.
The src
folder contains the next level of important files — router
, theme
and views
. Let’s see what these are
- Router files :- This folder contains the router file in which all routes of the application is added and each route have its
View
component with route name (pretty similar to Ionic Angular) .These files essentially decide how each page can be reached, with what name, and if there are any conditions attached to the routing.
Ionic Vue router essentially is based on Vue router itself, hence the concepts can be taken from Vue router itself.
In above code, Home
route is defined as the default path (i.e. the first page to load when application starts). We can define any other page to be the first to load.
You can lazy load routes as
{
path: '/detail',
name: 'Detail',
component: () => import('@/views/DetailPage.vue')
}
Read more about routing in official documentation here.
2. Theme files :- It contains the CSS files, In which we can define the global and default theme for the application. E.g. If we want to have a global theme in the application, we can define the color theme in CSS file. Ionic already has a theme defined where primary
, secondary
, danger
etc keywords provide specific colors to different UI elements.
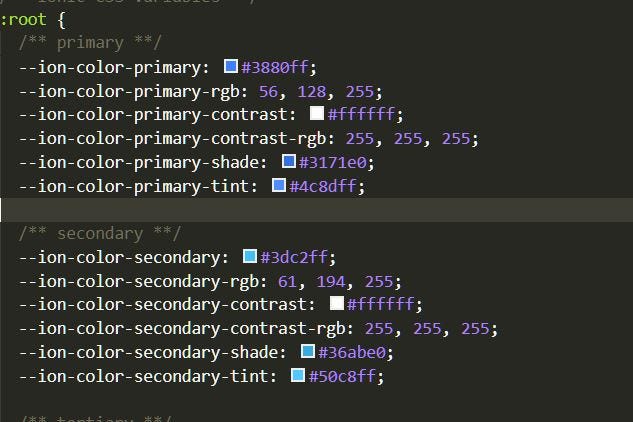
You can define your own primary, secondary colors which will effect your application themes.
3. View files :- Views are basically the screens in you application like Home screen, Settings screen. In this section we have to define our physical as well as logical view.
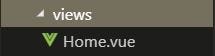
Add a new page and Navigate
You can generate more screens in your application by simply creating new file in you view page and add its route in router file.
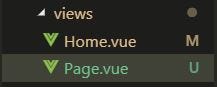
I have added a Page.vue
file in views folder and now we can create a new route for it in our routers file (i.e. router/index.ts
)
Add the following in the router/index.ts
... , { path: '/page', name: 'Page', component: Page }, ...
In above code, we have added a new route for the Page
Component that we have created. We’ll now do the following
- Navigate from Home to Page
- Navigate back from Page to Home
This will essentially just require us to define a click
action on an IonButton
and define the router
in each of the pages. The home page code will look like this
Notice the following
- Router import
- Setup function defining router
- The expression for click action and navigation
The above Vue component code is the most basic code anyone can write, But we will understand the basics of it like above code is divided in three major parts :-
- Template Part :- The HTML or the View part is enclosed by the template tags. This contains the button as well
- Functional Part (i.e. JavaScript part) :- In this part most of the logics and events resides e.g. we can import various components here and then use them in the template.
- CSS part :- In this part we can define the CSS classes and IDs.
In the export default part (i.e. functional part), we can define various options like :-
- Components :- In this we can define the
IonComponents
(Prebuild) or Some custom components. To use any component in template part first we have to define it in the Components option. - setup() method :- The
setup()
function is part of Vue 3.0, and it defines a slightly improved method of defining the components using Composition API. It’s a little different if you have been using Vue 2.0. This function acts like a constructor in Vue, like if we want to load it we can load it and return, and later use that in template code. For example we load therouter
in each component like this
setup() { const router = useRouter(); return {router}; },
We can define the router by using global useRouter()
method and then return it. And we used it on a button click :-
<ion-button @click="()=>{router.push('/page')}">Go to next page</ion-button>
This button will take us to the PageComponent
. Similarly, from next page we can return back to Home
, using similar code as above.
3. Props options :- Yes like React, we can also pass props
in Ionic Vue. You can define the custom component in Home Page and pass in the prop value :-
<template> <custom-component fist-prop="somePropValue"></custom-component> <template>
Once you have defined the custom-component in the template of Home Page, then you have to register the custom component in main.ts
file below the createApp()
method.
app.component('custom-component', customComponent);
You can get the props in the CustomComponent
and use it by interpolation :-
<template> <ion-page> <h1>{{fistProp}}</h1> </ion-page> </template> export default defineComponent({ import {IonPage} from '@ionic/vue'; props: ['firstProp'], name: 'CustomComponent', components: { IonPage, } });
By using this procedure you can pass on the props between the different level components and make you work easy.
Lifecycle Methods
Vue has its own set of lifecycle methods, but Ionic has done a superb job to standardize those on the same lines as Angular and React. This way you end up with the same set of lifecycle methods in any framework.
We’ll use ionViewDidEnter
lifecycle method on Page.vue
component to trigger an alert when we navigate to this page from Home. Here’s how the <script>
tag for Page.vue
looks like
Notice ionViewDidEnter
is not written as another method, but outside the methods
object, and it call a presentAlert
method from the methods
object.
Here’s how the two page app will look like with navigation and alert triggered in a lifecycle event.
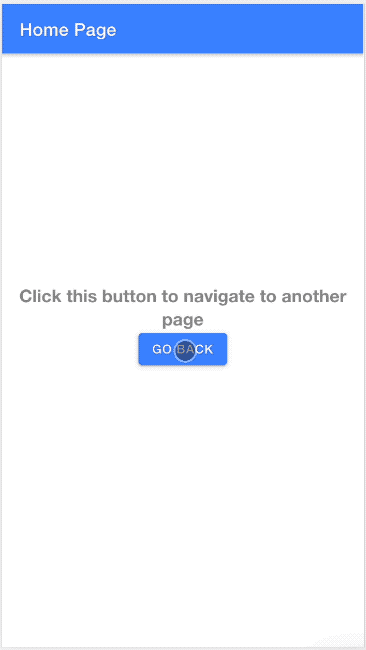
Ionic Lifecycle methods vs Vue Lifecycle methods
There are few subtle differences between Vue and Ionic lifecycle methods and associated components.
- During navigation Ionic Framework keeps the old page in the existing DOM, but hide it from your view and transition the new page. This is for smoother transition and state preservation in a mobile app. Due to these transitions certain Vue Router components such as
<keep-alive>
,<transition>
, and<router-view>
should not be used in Ionic Vue applications - Since Ionic Vue manages the lifetime of a page, certain events might not fire when you expect them to. For instance,
mounted
fires the first time a page is displayed, but if you navigate away from the page Ionic might keep the page around in the DOM, and a subsequent visit to the page might not callmounted
again.
For these reasons, you should use Ionic lifecycle methods as they are supposed to be used. Main lifecycle methods are
ionViewWillEnter
, ionViewDidEnter
, ionViewWillLeave
and ionViewDidLeave
More details on the lifecycle methods is available in official documentation.
Build Ionic Vue app for device
To build Ionic Vue application in your Android or iOS device/emulator you just have to run some ionic commands :-
// Add android platform $ npx cap add android
// Add iOS platform $ npx cap add ios
// Build web assets to copy to platforms $ ionic build
// copy the build assets to platforms $ npx cap copy
// open android studio to build app $ npx cap open android
// open xcode to build app $ npx cap open ios
These above command will build the application on you device or emulator and you can use them as rest of the native applications.
Conclusion
In this tutorial we learnt how to work with Ionic Vue application. We also learnt basics of Vue framework — Routing, Lifecycle events and basic syntax of view files.
Stay tuned for next Ionic Vue blogs where we will be exploring more features. If you want to explore more about Ionic or any other technology you can visit Enappd Blogs.