PayPal Payment Integration using Braintree in Ionic 6 Vue app
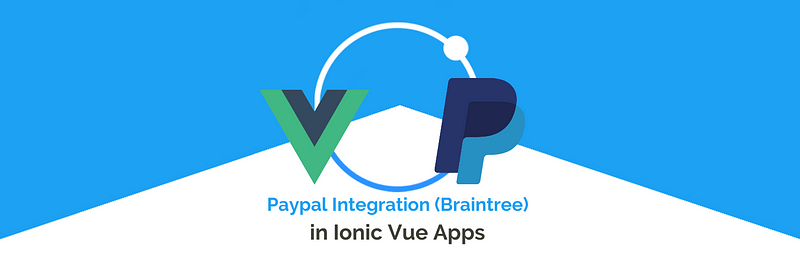
In this tutorial, we will go through PayPal payment using Braintree in Ionic 6. Previously Paypal supported the Cordova PayPal Plugin, which is no more maintained by PayPal, hence it has issues while making payments using PayPal Login. PayPal now recommends using Braintree for mobile payments, while web payment still works fine with the generic PayPal JS script. You can read more about the web integration in our blog — PayPal integration in Ionic PWA.
For ease of development, it is recommended to have same payment method i.e. Braintree for both mobile and web, instead of PayPal for web and Braintree for mobile.
This type of integration requires a Backend (server) to generate tokens and the final transaction is also done by the server. For this tutorial, we will be using a Node JS server and will be calling APIs from the Ionic app using an HTTP client.
Steps to Implement PayPal Integration Using Braintree:-
- Create a Braintree sandbox account and get the required keys
- Setup Ionic 6 Vue project
- Setup Node JS project
- Creating Node JS APIs for Braintree
- Braintree/PayPal integration in an Ionic app using Node JS API’s
- Testing
Before we proceed, a little bit about Ionic and Braintree
What is Braintree?
Braintree offers a variety of products that make it easy for users to accept payments in their apps or website. Think of Braintree as the credit card terminal you swipe your card through at the grocery store. Braintree supports the following major types of payment methods
- ACH Direct Debit
- Apple Pay
- Google Pay
- PayPal
- Samsung Pay
- Credit / Debit cards
- Union Pay
- Venmo
What is Ionic?
Ionic framework is a mobile app framework, which supports other front-end frameworks like Angular, React, Vue, and Vanilla JS, and builds apps for both Android and iOS from the same code.
If you create Native apps in Android, you code in Java. If you create Native apps in iOS, you code in Obj-C or Swift. Both of these are powerful but complex languages. With Ionic you can write a single piece of code for your app that can run on both iOS and Android and web (as PWA), that too with the simplicity of HTML, CSS, and JS.
It is important to note the contribution of Cordova/Capacitor in this. Ionic is only a UI wrapper made up of HTML, CSS, and JS. So, by default, Ionic cannot run as an app on an iOS or Android device. Cordova/Capacitor is the built environment that containerizes (sort of) this Ionic web app and converts it into a device installable app, along with providing this app access to native APIs like Camera, web access, etc.
Ionic and Payment Gateways
Ionic can create a wide variety of apps, and hence a wide variety of payment gateways can be implemented in Ionic apps. The popular ones are PayPal, Stripe, Braintree, in-app purchases, etc. For more details on payment gateways, you can read our blogs on Payment Gateway Solutions in Ionic, Stripe integration in Ionic, Apple pay in Ionic etc.
Also, there are different types of Ionic Apps you can build for the same functionality. The most popular ones are :
- Front-end: Angular | Build environment: Cordova
- Front-end: Angular | Build environment: Capacitor
- Front-end: React | Build environment: Capacitor
- Front-end: Vue | Build environment: Capacitor ✅
PayPal can be integrated into websites as well as mobile apps. In this blog, we’ll learn how to integrate the PayPal payment gateway in Vue/Capacitor Ionic 6 apps using Braintree.
OK, enough of talking, let’s start building our PayPal Braintree Payment System.
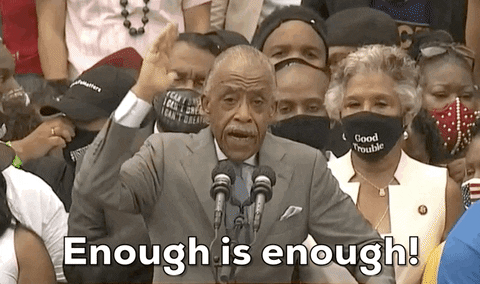
Step 1 — Create Braintree sandbox account and get the required keys
This step is required to proceed further, we will be needing several keys to integrate PayPal in the Ionic 6 app as well as in Node JS script. To get these keys to go to Braintree Sandbox Account. Once you log in, you can go to settings and select API.
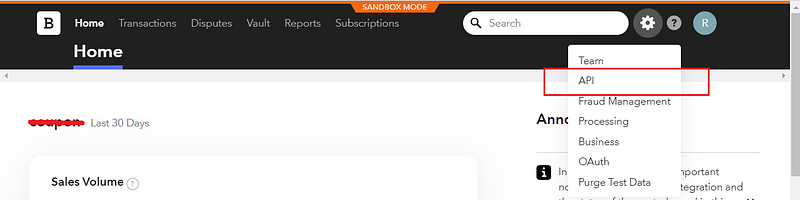
In the API section, scroll down and you will get the Required API key. We need three keys to initialize Braintree in Node JS scripts
- Merchant Id
- Public Key and
- Private Key

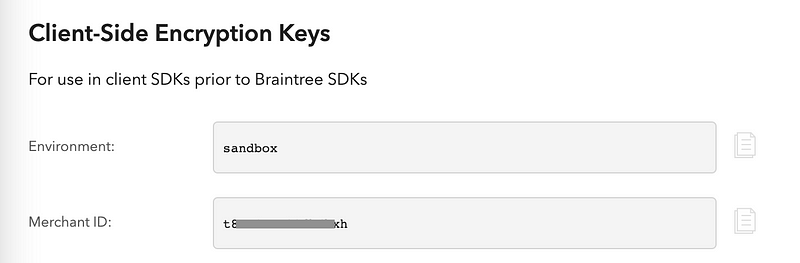
Save these IDs, we will use them in initializing the Braintree on the server-side.
Step 2 — Set up the Ionic 6 project
In this part, we will create and configure the Ionic 6 Vue Project and also set up a new Node JS Script.
Creating and configuring the Ionic 6 application.
To create a new Ionic 6 project, run the below command:-
$ ionic start PayPal --blank --type=vue
This will create a blank app in the working directory and if you want to know more about creating the Ionic 6 project, go to Ionic Vue Beginners Blog. Now once we have created the Ionic Vue app, we can configure the app for Braintree, to do that follow the below steps. Before that, you can install the Axios (that will be needing to call node server APIs) package using the below command:-
$ npm i axios
a) Mount CDN to Ionic Vue app in home.vue
file
How will the Ionic app load Braintree? Currently, there is no Cordova or Capacitor plugin for Braintree. Hence we will add the CDN to the home file i.e. home.vue, below is the CDN for Braintree JavaScript SDK.
<script src="https://js.braintreegateway.com/js/braintree-2.32.1.min.js"></script>
By adding this SDK CDN, Braintree will be available throughout the application. Below is the method you can use to mount the CDN on home.vue page.
createElement method will create an HTML component dynamically and we can add the src element (i.e. Braintree CDN). Once the script tag is loaded, then we have to initialize the Braintree setup using the event listener.
b) Using Braintree variable in Ionic 6 application
Once we mount the SDK CDN, we can use the Braintree variable on any page by using the below-defined syntax
declare const braintree;
Now in home.vue we can add this, we will use this variable to set up the Client-side application for successful payment.
Step 3 — Setup Node JS project
Create a separate folder for the node server. To create a Node JS script, we will run npm init
in the working directory.
$ npm init
The above command will ask a few basic questions and creates the package.json
file in the working directory. Now you can create the index.js
file (All logic will be contained in the index file because we’re making a simple server). Once you have run the above commands, the project structure will look like this
We have to install some of the libraries that will help in implementing the node script. To install the libraries run the below command:-
$ npm install cors express braintree body-parser
To know more about cors, express, body-parser, and braintree you can follow the links. You can also check official Ionic documentation on CORS which we believe is very good for understanding.
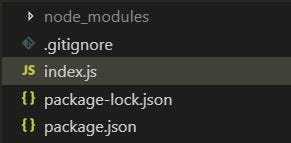
Now we have all the basic requirements to start our node script.
Note — Check your project’s package.json
file, it should contain the value stated below in the scripts section. If it doesn’t, just add manually:-

Step 4 — Creating Express JS APIs for Braintree
Creating Braintree APIs will allow us to get the Client ID requested from the client-side. We will use the Braintree package to implement those APIs. First of all, import the Braintree using the below command in index.js
file
const braintree = require("braintree");
Now use the Braintree object to initialize the Braintree Gateway. This initialization requires those keys (which we have saved in step 2) and environment value (i.e. for now we are using Sandbox, later you can use production value). Use the below command to initialize the Gateway.
const gateway = new braintree.BraintreeGateway({ environment: braintree.Environment.Sandbox, merchantId: "USE_YOUR_MERCHENT_ID", publicKey: "USE_YOUR_PUBLIC_KEY", privateKey: "USE_YOUR_PRIVATE_KEY" });
This Gateway object will be used to get the Client ID and used in doing transactions.
Payment Flow with Braintree
The following flowchart will get you up to speed with the payment flow. With this in mind, you’ll be able to better follow the code steps given further
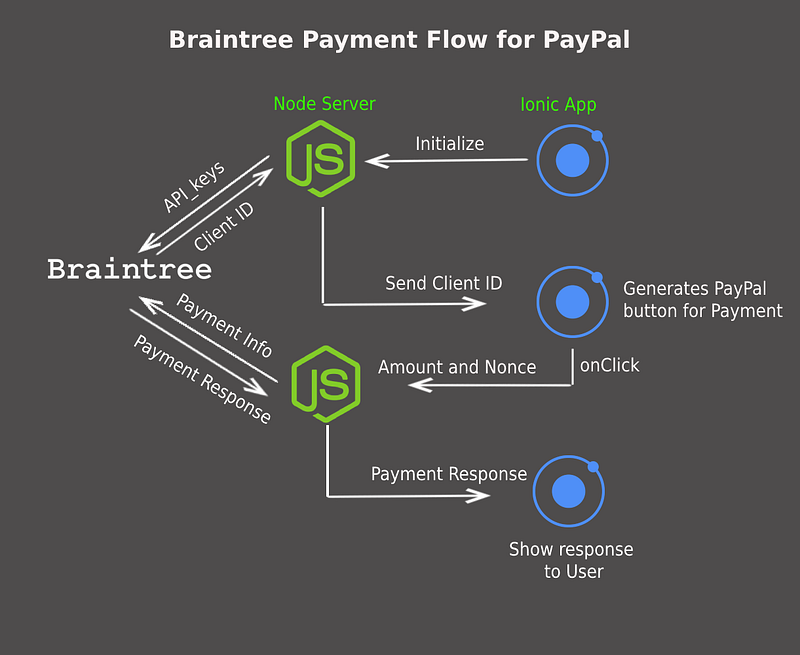
First, our Ionic application will call the Node JS API that will return the CLIENT ID generated using the API mentioned below:-
app.get("/brainTreeClientToken", (req, res) => { gateway.clientToken.generate({}).then((response) => { console.log('Token', response); res.send(response); }); });
‘/brainTreeClientToken’ API will be called from the Ionic app using Axios (later we will see in step 4). Once the client-side (Ionic app-side) gets the token it will set up the Braintree using the setup call (will see in step 4). After setup, the client will initiate payment using the Pay with PayPal button. Clicking the PayPal button will open In-App Browser and the sandbox will create a random user and provide info containing nonce (the token string used in making the transaction successful).
For making transactions, we will create Node JS API that will checkout payment using amount and nonce. Below is the transaction API:-
app.post("/checkoutWithPayment", jsonParser, (req, res) => { const nonceFromTheClient = req.body.nonceFromTheClient; const payment = req.body.paymentAmount; gateway.transaction.sale({ amount: payment, paymentMethodNonce: nonceFromTheClient, options: { submitForSettlement: true } }).then((result) => { res.send(result); }); });
In the above API nonceFromTheClient and paymentAmount will be passed through the Ionic 6 app and using the gateway object we will make a transaction. This will return a transaction status containing complete info about the customer, and payment and also contains the status of payment. This will complete the flow of payment using PayPal. Below is the complete script code:-
Note
- In the above script, you can replace
route
it inapp.listen
with the port3000
. So it becomesapp.listen(3000,()=>...
- You can also create
.catch
for the.generate
and.sale
methods in the server. This way, if you get any error from the Braintree server, you will be able to see that in the node server terminal
Run the server using npm start
or if you want to deploy code to some cloud server you can do that as well. If you’re running the server in the local system, the APIs will have a localhost
URL. You can test web payments directly in the local system. For phone payments, you might have to deploy the node server on a cloud.
Step 5 — Braintree integration in Ionic Vue App using Node JS APIs
In this part we will go through client-side (Ionic app) integration, using which we can make the PayPal transaction. We have seen how to initialize Braintree in the Ionic app (stated in step 2).
declare const braintree
The following method can be used to retrieve the client ID from the server. This method will be called during the Braintree setup.
getClientTokenForPaypal(): Promise <any> { return new Promise((resolve) => { Axios.get('https://couponappserver.herokuapp.com/brainTreeClientToken').then((res) =>{ resolve(res.data); }); }) },
Now we can set up Braintree using the below code. Notice that the .setup
is the function that defines for the first time that we are going to use PayPal as the payment gateway, using Braintree as the provider.
Keep in mind this setup will require the CLIENT_ID that will be generated by server API (mentioned in Step 3). So first we will call the server API and then pass the CLIENT_ID to setup method. You can call this function when page loads ( i.e. in mounted() method). In this case, we are calling this function once script is loaded to application
initializeBraintree() { const that = this; this.getClientTokenForPaypal().then((res: any) => { let checkout: any; braintree.setup(res.clientToken, 'custom', { paypal: { container: 'paypal-container', }, onReady: function (integration: any) { checkout = integration; }, onCancelled: () => { checkout.teardown(() => { checkout = null }); }, onPaymentMethodReceived: (obj: any) => { checkout.teardown(() => { checkout = null; that.handleBraintreePayment(obj.nonce); }); } }); }); }
In the above method, first, we call the getClientTokenForPaypal()
the method that will call the Node JS server brainTreeClientToken
API and returns the token. Once we get the client token, we can set up Braintree using it. This setup method will initialize the PayPal-container that will be used to render the PayPal button in HTML.
Once the client token is obtained, the Paypal button will be automatically rendered in the HTML file in this place. Make sure you have this in your HTML file
<ion-button class="paypalCss" id="paypal-container"></ion-button>
This will render the Paypal button in Page view and will look like the below screen :-

Now we have completed the setup and we can make payment using this button. When we click the button it will open the In-app Browser and the sandbox will handle the payment and users info. This will return the nonce value in the callback defined in the setup call.
One more thing we have to keep in mind is that sometimes, the PayPal payment screen does not go off when we make a payment (due to some screen refresh JavaScript issues). To remove it manually, we have to use the teardown() method defined in the checkout variable (initialized on onReady() callback).
onReady: function (integration) { checkout = integration; },
Below is the callback that will handle the nonce token and pass it on to another method that will handle the transaction.
onPaymentMethodReceived: (obj) => { checkout.teardown(() => { checkout = null; that.handleBraintreePayment(obj.nonce); }); }
We will pass the nonce value to handleBraintreePayment() method that will further call the transaction API defined in Node JS server. Here you can reinitialize the Braintree and show an alert after payment.
handleBraintreePayment(nonce: any) { this.makePaymentRequest(this.paymentAmount,nonce) .then((transaction: any) => { this.initializeBraintree(); this.showDoneAlert(); }) }
The abovemakePaymentRequest() method will call the transaction API defined in Node JS server, which will make the original payment using Braintree. Below is the function call:-
makePaymentRequest(amount: any, nonce: any): Promise <any> { return new Promise((resolve) => { const paymentDetails = { paymentAmount: amount, nonceFromTheClient: nonce } Axios.post('https://couponappserver.herokuapp.com/checkoutWithPayment', paymentDetails) .then((res) =>{ resolve(res.data); }); }); }
The above function will contain the paymentAmount and nonce value and will pass them to the server API. That will return the transaction object and we can decide the client end logic according to the response that we get from the server. This will complete the PayPal Braintree integration from both the client-side and server-side. Below is the complete Vue and ts file code:-
Step 6 — Testing
As mentioned earlier, it is easy to test this setup in a web domain, because the server can run on localhost, and the Ionic app can call the server while running with ionic serve
. Here is how the payment flow looks
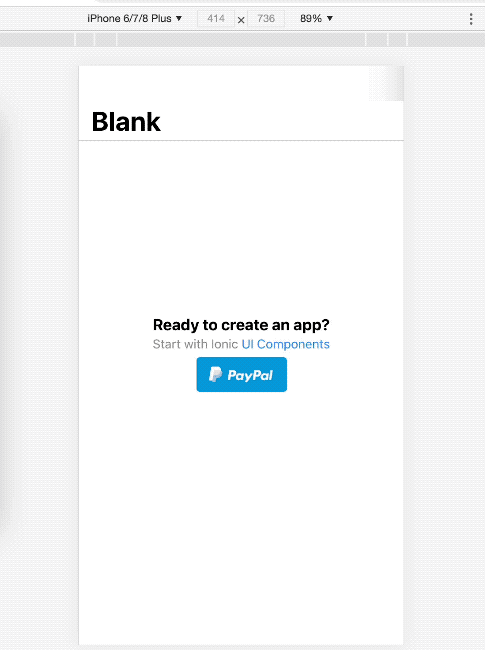
To test it on your device, you will need to deploy the server on a cloud so you can call the APIs from the app. If there is a way you can call localhost APIs from your app (I don’t know any), that should work as well!
Conclusion:-
Congratulations !! 🎉 You just learned how to integrate the awesome Braintree payment system to make payments using PayPal.
You can make payments using both PayPal accounts and Debit / Credit Cards. This way of integration can work both in Web view and mobile apps. If you want to know more about any feature integration, you can check out our amazing tutorials at Enappd Blogs.
Happy coding!
Next Steps
If you liked this blog, you will also find the following blogs interesting and helpful. Feel free to ask any questions in the comment section
Ionic Capacitor
- Basic — Geolocation | Barcode & QR code | Facebook Login (Angular) | Facebook Login (React) | Icon and Splash | Camera & Photo Gallery | Debugging with browser|Theming in Ionic apps
- Advanced — AdMob | Local Notifications | Google Login | Twitter Login | Games using Phaser | Play music | Push Notifications
Ionic Cordova
- Taxi Booking App example with Ionic, Node, Express and MySQL
- Ionic Payment Gateways — Stripe with Firebase | Stripe with NodeJS | PayPal | Apple Pay | RazorPay
- Ionic Charts with — Google Charts| HighCharts | d3.js | Chart.js
- Ionic Authentications — Via Email | Anonymous | Facebook | Google | Twitter | Via Phone
- Ionic Features — Geolocation| QR Code reader | Pedometer| Signature Pad | Background Geolocation
- Media in Ionic — Audio | Video | Image Picker | Image Cropper
- Ionic Essentials — Debugging with browser| Native Storage | Translations | RTL | Sentry Error Monitoring | Social sharing
- Ionic messaging — Firebase Push | Reading SMS | Local Notifications
- Ionic with Firebase — Basics | Hosting and DB | Cloud functions | Deploy App to Firebase | Firebase simulator
- Unit Testing in Ionic — Part 1 | Mocks & Spies| Async Testing
Ionic React Full App with Capacitor
If you need a base to start your next Ionic 5 React Capacitor app, you can make your next awesome app using Ionic 5 React Full App in Capacitor
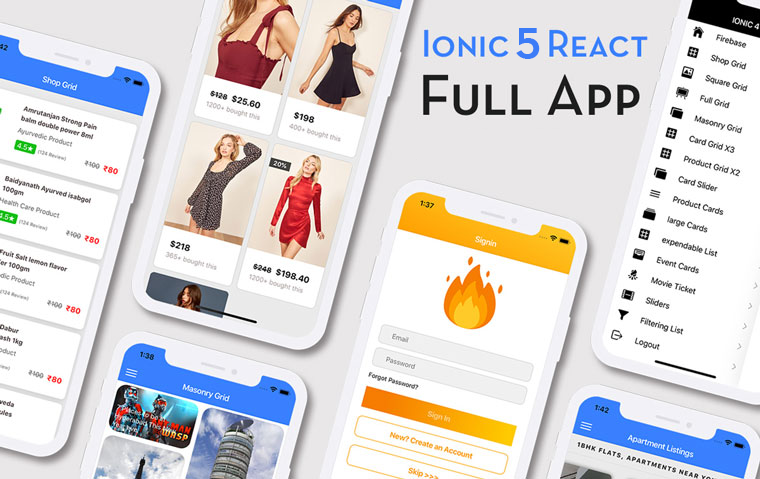
Ionic Capacitor Full App (Angular)
If you need a base to start your next Angular Capacitor app, you can make your next awesome app using Capacitor Full App
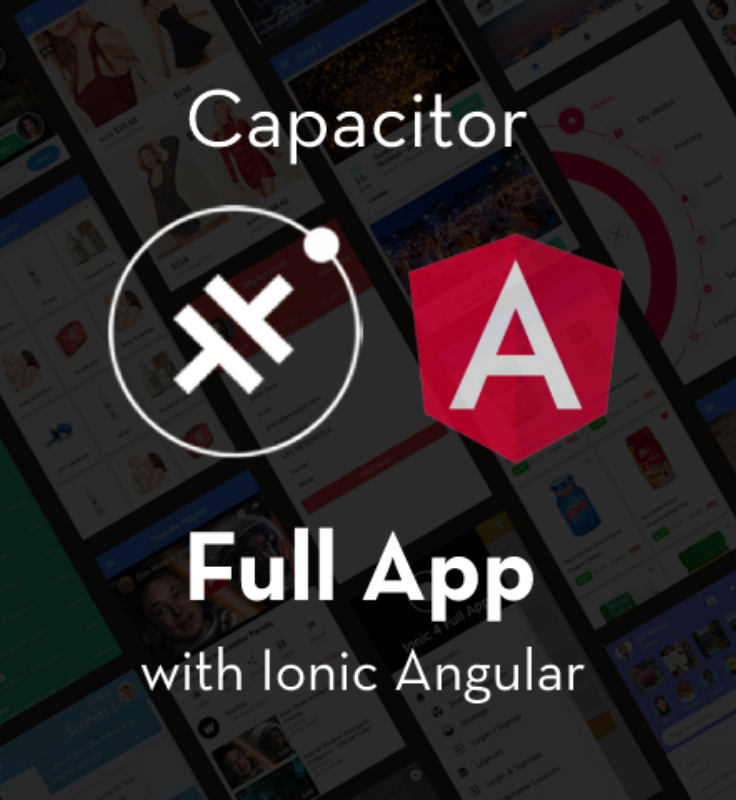
Ionic Full App (Angular and Cordova)
If you need a base to start your next Ionic 5 app, you can make your next awesome app using Ionic 5 Full App
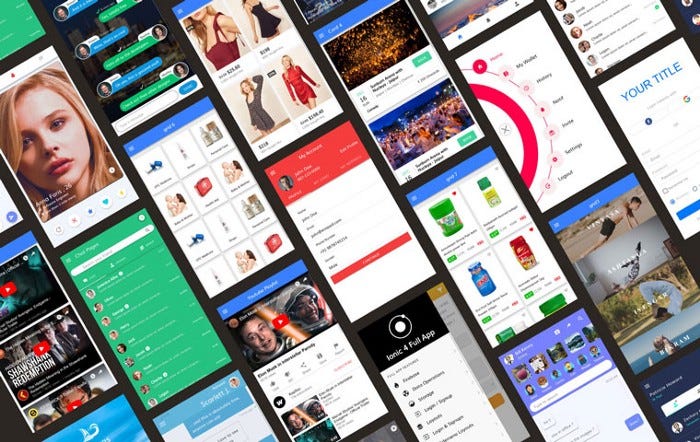